In JavaScript, combining two lists is a fundamental operation with various practical applications. Whether merging data from multiple sources or dynamically extending arrays, the ability to combine lists efficiently is crucial for many programming tasks. This blog explores the importance of combining lists in JavaScript, delving into scenarios where it proves invaluable. Readers will discover multiple methods for combining lists, including basic concatenation, the ES6 spread operator, and using array methods like `push()` and `apply()`.
1. Basic Method: Concatenation
Refer to the below code of Combine Two lists in Javascript using concat()
<!DOCTYPE html> <html> <head> <title>Add two lists using concat()</title> <style> body{ font-family: Arial, sans-serif; } </style> </head> <body> <h1>Add two lists using concat()</h1> <!-- create two lists with different items --> <ul id="list1"> <li>Strawberry</li> <li>Blueberry</li> <li>Cranberry</li> </ul> <ul id="list2"> <li>Potato</li> <li>Broccoli</li> <li>Tomato</li> </ul> <!-- create a button to trigger the function --> <button onclick="addLists()">Add Lists</button> <!-- create a placeholder for the result --> <p id="result"></p> <script> // define a function to add two lists function addLists(){ // get the two lists using their ids var list1 = document.getElementById("list1"); var list2 = document.getElementById("list2"); // use the concat() method to combine the two lists var combinedList = list1.concat(list2); // display the result in the placeholder document.getElementById("result").innerHTML = combinedList; } </script> </body> </html>
Explanation of the code:
This HTML document illustrates how to combine two lists in Javascript using the `concat()` method in JavaScript.
- Two unordered lists contain different items, and a button labeled “Add Lists” triggers the
addLists()
function when clicked. - Within this function, it retrieves the two lists by their IDs, then applies the `concat()` method to merge them into a single array.
- Finally, it updates the content of a paragraph element with the ID “result” to display the combined list.
- However, there’s a mistake in the code: `getElementById()` returns DOM elements, not arrays. To convert the HTMLCollection to arrays, use Array.from() to properly concatenate the lists.
Learn how to combine two lists in Python with unique values here!
Output:
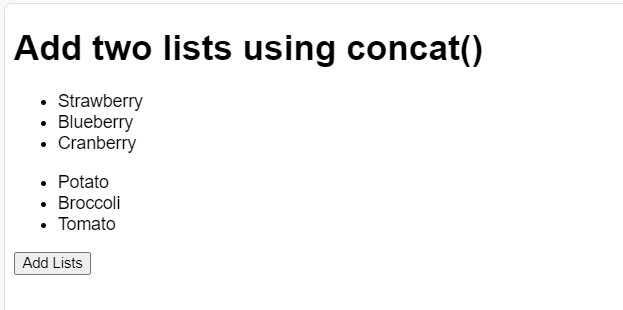
2. Combine Two lists in Javascript- ES6 Spread Operator
Below is a code snippet showcasing the spread operator for combine two list in JavaScript:
<!DOCTYPE html> <html> <head> <title>Add two lists using ES6 Spread Operator</title> <style> body { font-family: Arial, sans-serif; } </style> </head> <body> <h1>Add two lists using ES6 Spread Operator</h1> <!-- create two lists with different items --> <ul id="list1"> <li>Shark</li> <li>Elephant</li> <li>Eagle</li> </ul> <ul id="list2"> <li>Water</li> <li>Land</li> <li>Sky</li> </ul> <!-- create a button to trigger the function --> <button onclick="addLists()">Add Lists</button> <!-- create a placeholder for the result --> <ul id="result"></ul> <script> // define a function to add two lists using the ES6 Spread Operator function addLists() { // get the two lists using their ids var list1 = document.getElementById("list1").getElementsByTagName("li"); var list2 = document.getElementById("list2").getElementsByTagName("li"); // convert HTMLCollection to arrays and extract text content var array1 = Array.from(list1, item => item.textContent); var array2 = Array.from(list2, item => item.textContent); // use the ES6 Spread Operator to combine the two arrays var combinedList = [...array1, ...array2]; // display the result in the placeholder var resultElement = document.getElementById("result"); resultElement.innerHTML = ""; combinedList.forEach(item => { let li = document.createElement("li"); li.textContent = item; resultElement.appendChild(li); }); } </script> </body> </html>
Explanation of the Code:
1.HTML Structure:
- Two unordered lists (<ul>) with IDs list1 and list2, each containing list items (<li>).
- A button that triggers the addLists() function when clicked.
- A placeholder unordered list with ID result where the combined items will be displayed.
2. JavaScript Function:
- addLists() retrieves the list items from list1 and list2 using getElementsByTagName(“li”).
- Converts the HTMLCollection to arrays using Array.from(), extracting the text content of each list item.
- Combines the arrays using the ES6 Spread Operator ([…array1, …array2]).
- Updates the result list by creating new <li> elements for each combined item and appending them to the result element.
This approach ensures the lists are combined and displayed correctly using modern JavaScript syntax.
Don’t miss to check out the blog on how to combine two lists in C!
Output:
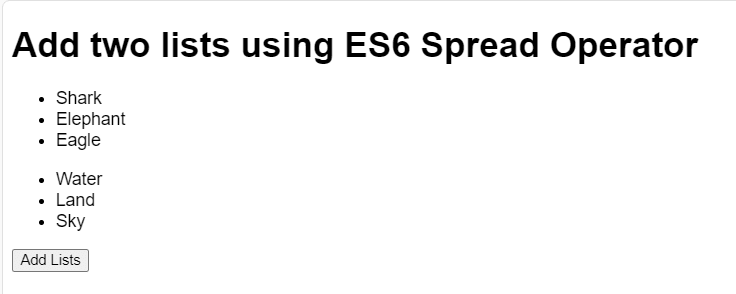
The provided code displays how to combine two lists in JavaScript using the `push()` and `apply()` application.
3. Using Array Methods: `push()` and `apply()`
<!-- This program combines two arrays using the push() and apply() methods in JavaScript. --> <!DOCTYPE html> <html> <head> <title>Combine Lists</title> <style> body { font-family: Arial, sans-serif; } </style> </head> <body> <h1>Combine Lists</h1> <p>Enter the elements of the first list:</p> <!-- Create a form to input the elements of the first array --> <form id="form1"> <input type="text" id="array1-1" placeholder="Enter element"> <input type="text" id="array1-2" placeholder="Enter element"> <input type="text" id="array1-3" placeholder="Enter element"> <input type="text" id="array1-4" placeholder="Enter element"> <input type="text" id="array1-5" placeholder="Enter element"> </form> <p>Enter the elements of the second list:</p> <!-- Create a form to input the elements of the second array --> <form id="form2"> <input type="text" id="array2-1" placeholder="Enter element"> <input type="text" id="array2-2" placeholder="Enter element"> <input type="text" id="array2-3" placeholder="Enter element"> <input type="text" id="array2-4" placeholder="Enter element"> <input type="text" id="array2-5" placeholder="Enter element"> </form> <button onclick="combineArrays()">Combine Lists</button> <!-- Create a button to trigger the combineArrays() function --> <p id="result"></p> <!-- Create a paragraph to display the result of the combined arrays --> <script> // Define a function to combine the arrays function combineArrays() { // Get the input elements from the forms var form1 = document.getElementById("form1"); var form2 = document.getElementById("form2"); // Initialize arrays to hold the values from the input fields var arr1 = []; var arr2 = []; // Loop through the input fields of form1 and form2 to gather values for (var i = 0; i < form1.elements.length; i++) { if (form1.elements[i].value !== "") { arr1.push(form1.elements[i].value); } } for (var i = 0; i < form2.elements.length; i++) { if (form2.elements[i].value !== "") { arr2.push(form2.elements[i].value); } } // Use the push() method to add the elements of the second array to the first array Array.prototype.push.apply(arr1, arr2); // Display the combined array in the result paragraph document.getElementById("result").innerHTML = "The combined array is: " + arr1.join(", "); } </script> </body> </html>
Explanation of the Code:
- Unique Identifiers: Each input field now has a unique id attribute to ensure they can be uniquely identified.
- Forms: Both forms have unique id attributes (form1 and form2) to easily access their elements.
- JavaScript Function:
- The combineArrays function retrieves all values from the input fields in both forms.
- It pushes these values into separate arrays (arr1 and arr2).
- The Array.prototype.push.apply(arr1, arr2) method is used to combine arr2 into arr1.
- The combined array is then displayed in the result paragraph using innerHTML.
With this setup, users can enter values into the input fields, click the “Combine Lists” button, and see the combined array displayed below.
Output:
In conclusion, we hope you find our blog on ‘Combine Two Lists in Javascript’ beneficial in the way of mastering coding language. we encourage you to dive into programming activities and continue your learning journey with us. Experiment with combining lists in JavaScript and explore the vast resources on Newtum, including user-friendly blogs and courses. Happy coding!
FAQs about Combine Two Lists in Javascript
Combining lists allows for efficient data merging from multiple sources, essential for various programming tasks.
Key methods include concatenation, the ES6 spread operator, and array methods like push()
and apply()
.
Sure, list concatenation can be achieved using the concat()
method, as demonstrated in the blog.
By using the spread operator [...array1, ...array2]
, you can efficiently merge two arrays into one.
Yes, ensure proper data type handling and avoid unintended side effects, especially when dealing with heterogeneous data.