Python Program to Draw Chess Board Using Turtle
# Draw Chess Board in Python Using Turtle import turtle sc = turtle.Screen() cb = turtle.Turtle() # method to draw square def draw(): for i in range(4): cb.forward(30) cb.left(90) cb.forward(30) # Driver Code if __name__ == "__main__": # set screen sc.setup(400, 600) # set turtle object speed cb.speed(100) # loops for board for i in range(8): # not ready to draw cb.up() # set position for every row cb.setpos(-100, 30 * i) # ready to draw cb.down() # row for j in range(8): # conditions for alternative color if (i + j) % 2 == 0: col = 'black' else: col = 'white' # fill with given color cb.fillcolor(col) # start filling with colour cb.begin_fill() # call method draw() # stop filling cb.end_fill() # hide the turtle cb.hideturtle() turtle.done()
Intoduction
Python is a high-level programming language that is widely used in various fields, such as web development, data science, machine learning, and more. It is known for its simplicity and ease of use, making it an excellent choice for beginners and experts alike.
In this tutorial, we will learn how to draw a chessboard in Python using the turtle module. The turtle module in Python provides a simple way to create graphics and shapes using a virtual turtle. We will be using the turtle module to create a chessboard consisting of 64 squares, each square having a different color.
Explanation
1. First, we import the turtle module, which provides a simple way to create graphics and shapes using a virtual turtle.
import turtle
2. We then create a screen object and a turtle object named “cb”. The “cb” turtle will be used to draw the chessboard.
sc = turtle.Screen() cb = turtle.Turtle()
3. Next, we define a method named “draw()” that will be used to draw a square. This method uses a for loop to draw the four sides of the square by moving the turtle forward by a distance of 30 pixels and then turning it left by 90 degrees. After completing the square, the turtle moves forward by 30 pixels.
def draw(): for i in range(4): cb.forward(30) cb.left(90) cb.forward(30)
4. We then set the speed of our turtle object to 100 using the speed() method.
cb.speed(100)
5. We use a nested for loop to draw the chessboard consisting of multiple squares. The outer loop iterates over the rows of the chessboard, while the inner loop iterates over the columns. We use the up() and down() methods to move the turtle without drawing any lines. We then set the position of the turtle for each row using the setpos() method, and use the draw() method to draw each square.
We fill each square with a color based on its position using the fillcolor() and begin_fill() methods. If the sum of the row and column indexes is even, we fill the square with a white color, otherwise we fill it with black.
for i in range(8): cb.up() cb.setpos(-100, 30 * i) cb.down() for j in range(8): if (i + j) % 2 == 0: col = 'white' else: col = 'black' cb.fillcolor(col) cb.begin_fill() draw() cb.end_fill()
6. Finally, we hide the turtle using the hideturtle() method and use the done() method to keep the window open.
cb.hideturtle() turtle.done()
By following the above steps, we can create a beautiful chessboard using the turtle module in Python.
Output:
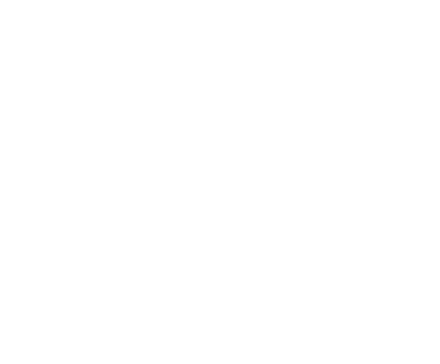
When you run the above code, a turtle graphics window will be opened with a chessboard drawn in the center of the screen. The design will consist of 64 squares, each square having a different color. The use of alternative black and white colors creates a chessboard-like pattern, making the design more visually appealing.
There are multiple ways to draw a chessboard in Python. Here are some of the other ways to draw a chessboard in Python:
- Using the Pillow Library: The Pillow library is a popular Python library for working with images. Using the Pillow library, we can create an image of a chessboard by creating a new image and drawing rectangles on it. We can use the ImageDraw module to draw rectangles on the image. We can also use the ImageFont module to add text to the image to label the rows and columns. The resulting image can be saved in various formats such as JPEG, PNG, etc.
- Using the Pygame Library: The Pygame library is a popular Python library for game development. Using the Pygame library, we can create a graphical chessboard using sprites. We can use the Sprite class to create sprites for each piece and the Board class to create the chessboard. We can also use the Pygame module to add animations and sound effects to the game.
- Using the Tkinter Library: The Tkinter library is a built-in Python library for creating graphical user interfaces. Using the Tkinter library, we can create a graphical chessboard by creating a canvas widget and drawing squares on it. We can use the Canvas widget to draw rectangles on the canvas and the Text widget to label the rows and columns. We can also use the Tkinter module to add buttons and other GUI elements to the chessboard.
- Using the Matplotlib Library: The Matplotlib library is a popular Python library for creating graphs and charts. Using the Matplotlib library, we can create a chessboard by creating a new figure and drawing squares on it. We can use the Axes object to draw rectangles on the figure and the Text object to label the rows and columns.
There are various ways for drawing a chessboard in Python, each with its own advantages and disadvantages. The choice of method depends on the specific requirements of the project and the expertise of the developer.
Conclusion
In this tutorial, we learned how to draw a chessboard in Python using the turtle module. We used the turtle module to create graphics and shapes using a virtual turtle. We also learned how to use the fillcolor(), begin_fill(), and end_fill() methods to fill squares with different colors. With a little creativity, you can create all sorts of shapes and graphics using the turtle module in Python.