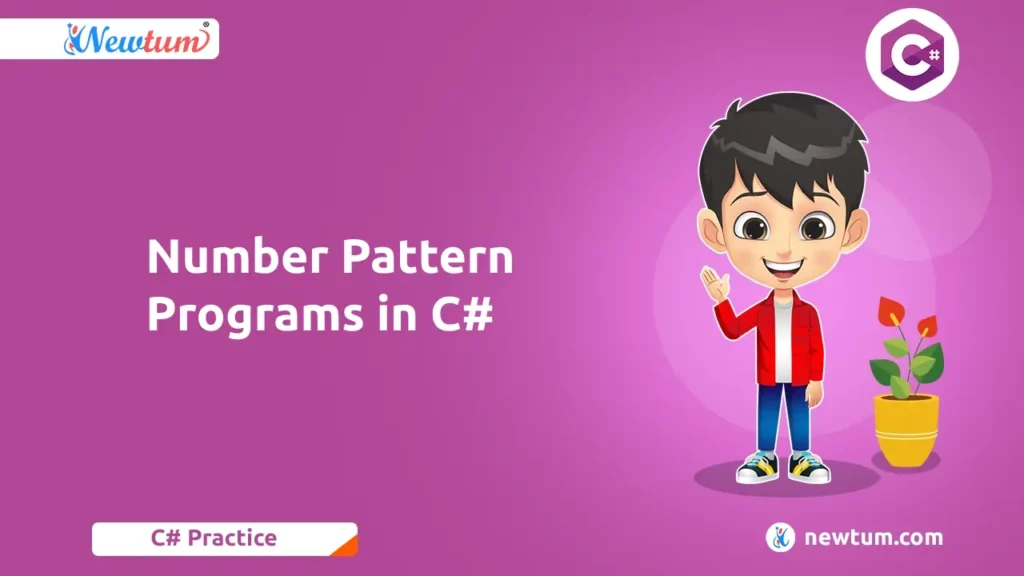
In this blog, Learn about Number Pattern Programs in C#. Delve into the fascinating world of coding as we explore the generation of captivating numerical patterns.
Number Pattern Program in C#
Number Pattern Programs in C# explore the generation of various numerical patterns using C# programming language, ranging from simple shapes to complex designs. By leveraging C#’s syntax and logic, programmers can create intricate visual patterns, enhancing their skills and understanding of algorithmic problem-solving.
Basic Number Patterns in C#:
Table of Contents
1. Ascending Order Pattern
// Import necessary libraries using System; // Create a class class Program { // Main method static void Main(string[] args) { // Prompt user to enter the number of rows Console.WriteLine("Enter the number of rows: "); // Read the input and convert it to integer int rows = Convert.ToInt32(Console.ReadLine()); // Loop through each row for (int i = 1; i <= rows; i++) { // Loop through each column for (int j = 1; j <= i; j++) { // Print the current number Console.Write(j + " "); } // Move to the next line Console.WriteLine(); } // Wait for user input before closing the console Console.ReadLine(); } }
Explanation of the code:
1. Import necessary libraries: The code begins with importing the System namespace, which contains classes used for basic operations such as input/output.
2. Create a class: A class named “Program” is defined, which serves as the entry point of the program.
3. Main method: Within the “Program” class, the Main method is declared. This is the starting point of execution for the program.
4. Prompt user to enter the number of rows: The program displays a message asking the user to input the number of rows for the number pattern.
5. Read user input: The Console.ReadLine() method reads the user’s input from the console, and Convert.ToInt32() converts the input string to an integer.
6. Loop through each row: The program iterates through each row from 1 to the number of rows entered by the user.
7. Loop through each column: Within each row, another loop iterates from 1 to the current row number.
8. Print the current number: The inner loop prints the current column number followed by a space.
9. Move to the next line: After printing each row, the program moves to the next line using Console.WriteLine().
10. Wait for user input: The program waits for the user to press the Enter key before closing the console window.
Output:
Enter the number of rows: 5
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
2. Square Pattern
//Importing necessary libraries using System; //Defining class class Program { //Main method static void Main(string[] args) { //Prompting user to enter the number of rows Console.WriteLine("Enter the number of rows: "); //Converting user input to integer int rows = Convert.ToInt32(Console.ReadLine()); //Outer loop for rows for (int i = 1; i <= rows; i++) { //Inner loop for columns for (int j = 1; j <= rows; j++) { //Printing the square pattern Console.Write(i * j + " "); } //Moving to next line Console.WriteLine(); } //Keeping the console window open Console.ReadLine(); } }
Explanation of the code:
This C# code generates a square number pattern based on user input for the number of rows. Here’s a step-by-step explanation:
1. The program prompts the user to enter the number of rows for the pattern.
2. User input is converted to an integer using `Convert.ToInt32()`.
3. Two nested `for` loops iterate through each row and column.
4. The product of row number `i` and column number `j` is printed as the pattern.
5. After each row, the program moves to the next line.
6. Finally, the program waits for user input to keep the console window open.
Output:
Enter the number of rows: 3
1 2 3
2 4 6
3 6 9
3. Right Triangle Pattern
using System; //importing the System library namespace RightTrianglePattern //defining the namespace { class Program //defining the class { static void Main(string[] args) //defining the main method { Console.WriteLine("Right Triangle Number Pattern"); //printing the title of the pattern Console.Write("Enter the number of rows: "); //asking for user input for the number of rows int rows = Convert.ToInt32(Console.ReadLine()); //converting the user input to integer and storing it in the variable 'rows' for (int i = 1; i <= rows; i++) //outer loop for the rows { for (int j = 1; j <= i; j++) //inner loop for the columns { Console.Write(j + " "); //printing the numbers in the pattern } Console.WriteLine(); //moving to the next line after each row is printed } } } }
Explanation of the code:
This C# code generates a right triangle number pattern based on user input for the number of rows. Here’s a step-by-step explanation:
1. The program prompts the user to enter the number of rows.
2. User input is converted to an integer using `Convert.ToInt32()`.
3. Two nested `for` loops iterate through each row and column.
4. The inner loop prints the numbers in the pattern, incrementing from 1 to the current row number.
5. After each row, the program moves to the next line using `Console.WriteLine()`.
6. The pattern is displayed in the console.
Output:
Right Triangle Number Pattern
Enter the number of rows: 5
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
4. Left Triangle Pattern
// Import necessary libraries using System; // Create a class class Program { // Main method static void Main(string[] args) { // Prompt user to enter the number of rows Console.WriteLine("Enter the number of rows: "); // Read the input and convert it to integer int rows = Convert.ToInt32(Console.ReadLine()); // Loop through each row for (int i = 1; i <= rows; i++) { // Loop through each column for (int j = 1; j <= i; j++) { // Print the number Console.Write(j + " "); } // Move to the next line Console.WriteLine(); } } }
Explanation of the code:
This C# code generates a pattern of numbers forming a right triangle. Here’s a step-by-step explanation:
1. The program prompts the user to input the number of rows.
2. User input is read and converted to an integer.
3. Two nested `for` loops iterate through each row and column.
4. Inner loop prints numbers incrementally from 1 to the current row number.
5. After printing each row, the program moves to the next line.
6. The resulting pattern is displayed in the console.
Output:
Enter the number of rows: 4
1
1 2
1 2 3
1 2 3 4
5. Diamond Pattern
// Import necessary libraries using System; // Create a class class Program { // Main method static void Main(string[] args) { // Prompt user to enter the number of rows Console.WriteLine("Enter the number of rows: "); // Read the input and convert it to integer int rows = Convert.ToInt32(Console.ReadLine()); // Loop to print the upper half of the diamond for (int i = 1; i <= rows; i++) { // Loop to print the spaces for (int j = 1; j <= rows - i; j++) { Console.Write(" "); } // Loop to print the numbers for (int k = 1; k <= i; k++) { Console.Write(i + " "); } // Move to the next line Console.WriteLine(); } // Loop to print the lower half of the diamond for (int i = rows - 1; i >= 1; i--) { // Loop to print the spaces for (int j = 1; j <= rows - i; j++) { Console.Write(" "); } // Loop to print the numbers for (int k = 1; k <= i; k++) { Console.Write(i + " "); } // Move to the next line Console.WriteLine(); } } }
Explanation of the code:
This C# code generates a pattern of numbers forming a right triangle. Here’s a step-by-step explanation:
1. The program prompts the user to input the number of rows.
2. User input is read and converted to an integer.
3. Two nested `for` loops iterate through each row and column.
4. Inner loop prints numbers incrementally from 1 to the current row number.
5. After printing each row, the program moves to the next line.
6. The resulting pattern is displayed in the console.
Output:
Enter the number of rows: 4
1
2 2
3 3 3
4 4 4 4
3 3 3
2 2
1
In conclusion, exploring Number Pattern Programs in C# offers both educational insight and practical application. I encourage readers to delve into the provided code on the Newtum Compiler, fostering hands-on learning and experimentation. Additionally, take advantage of the resources available on Newtum for comprehensive programming tutorials and courses. Keep coding and enjoy the journey ahead!