In C programming, programmers commonly perform the task of combining two lists for various scenarios like merging datasets or extending arrays dynamically.This is useful when you want to combine information from different sources. In this blog post, you will learn an easy way to combine two lists in C programming. We’ll provide code, explain what it does, and show you the result so you can use this trick in your own programs!
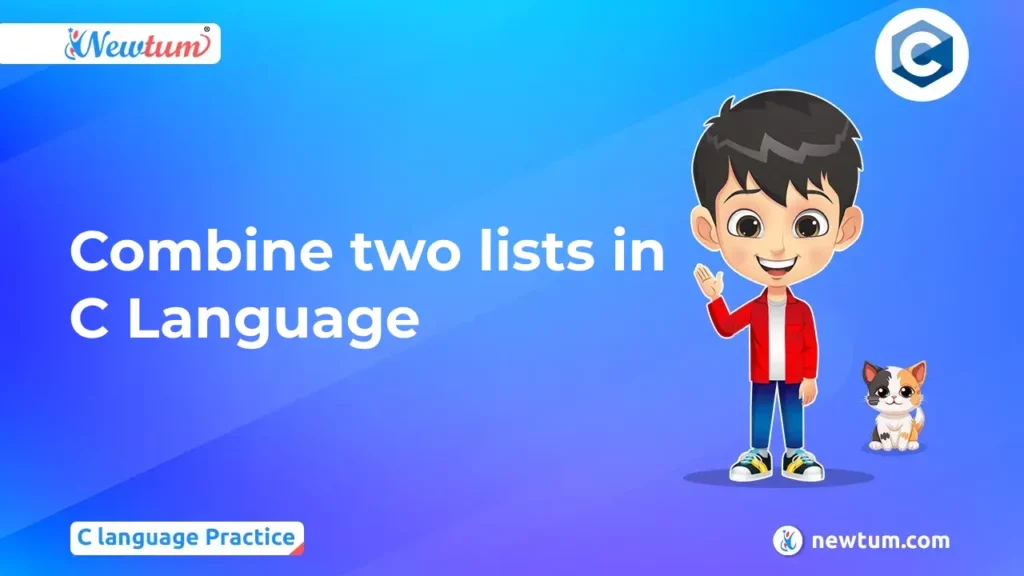
Combine two lists in C- Code
Check out the below code for a better understanding of how to Combine two lists in C.
//Combine two lists in C #include <stdio.h> void combineArrays(int arr1[], int size1, int arr2[], int size2, int result[]) { for (int i = 0; i < size1; i++) { result[i] = arr1[i]; } for (int i = 0; i < size2; i++) { result[size1 + i] = arr2[i]; } } int main() { int arr1[] = {1, 2, 3, 4}; int arr2[] = {5, 6, 7, 8}; int size1 = sizeof(arr1) / sizeof(arr1[0]); int size2 = sizeof(arr2) / sizeof(arr2[0]); int result[size1 + size2]; combineArrays(arr1, size1, arr2, size2, result); printf("Combined array: "); for (int i = 0; i < size1 + size2; i++) { printf("%d ", result[i]); } return 0; }
Explanation in the code:
The code illustrates how to combine two arrays in C.
The combineArrays
function accepts two arrays (arr1
and arr2
), their sizes (size1
and size2
), and a result
array. It first copies elements from arr1
into result
, then appends elements from arr2
. In the main
function, two arrays (arr1
and arr2
) are initialized. Their sizes are calculated using sizeof
, and the result
array is declared to hold the combined elements. The combineArrays
function merges arr1
and arr2
into result
. Finally, we print the combined array. The for
loop iterates over the combined array, outputting each element, resulting in the sequence “1 2 3 4 5 6 7 8”.
Output
Combined array: 1 2 3 4 5 6 7 8
Possible Errors and Solutions: Combine Two Lists in C
1. Uninitialized Result Array
Error: Declaring the `result` array without specifying its size.
Solution: Ensure that you properly size the result
array to hold the combined elements from both arrays.
int result[size1 + size2]; // Correctly sized array
2. Incorrect Array Size Calculation
Error: Using `sizeof` on pointers instead of arrays to calculate sizes.
Solution: Always use sizeof
on the array itself within the scope where you declare it.
int size1 = sizeof(arr1) / sizeof(arr1[0]); // Correct
int size2 = sizeof(arr2) / sizeof(arr2[0]); // Correct
3. Array Index Out of Bounds
Error: Accessing indices outside the bounds of the array due to incorrect loop conditions.
Solution: Ensure loop conditions accurately reflect the array bounds.
for (int i = 0; i < size1; i++) {
result[i] = arr1[i];
}
for (int i = 0; i < size2; i++) {
result[size1 + i] = arr2[i];
}
4. Incorrect Function Parameters
Error: Passing incorrect sizes or arrays to the `combineArrays` function.
Solution: Ensure the function parameters match the expected data types and sizes.
combineArrays(arr1, size1, arr2, size2, result); // Correct parameters
5. Memory Allocation for Dynamic Arrays
Error: Using dynamic memory allocation without properly allocating and freeing memory.
Solution: Allocate memory for the result
array using malloc
if its size is not known at compile time, and remember to free the memory afterward.
int *result = (int *)malloc((size1 + size2) * sizeof(int));
if (result == NULL) {
printf("Memory allocation failed");
return 1; // Exit program if memory allocation fails
}
// Use the result array as needed
free(result); // Free the allocated memory
6. Missing Return Statement in Main
Error: Forgetting to return an integer value from the `main` function.
Solution: Always include a return statement to indicate successful completion.
return 0; // Indicate successful execution
By addressing these common errors and following the solutions, you can ensure your code to combine two lists in C is robust and error-free.
In this blog, we’ve explored the blog ‘Combine two lists in C’, highlighting its educational and practical value. Experiment with this code to enhance your understanding. For more tutorials and courses in Python, C, PHP, HTML, and more, visit Newtum. Engage in programming and enjoy your coding journey. Happy coding!
Combine two lists in C- FAQ
Combining two lists in C is useful for merging datasets or extending arrays dynamically.
combineArrays
function work? The combineArrays
function copies elements from the first array and then appends elements from the second array into the result array.
Use sizeof(arr) / sizeof(arr[0])
to calculate the number of elements in an array.
result
array is not properly initialized? Ensure the result
array is correctly sized to hold all elements from both arrays.
Use malloc
to allocate memory for the result array and remember to free it afterward.