The Turtle module in Python simplifies graphics and animation creation, inspired by a turtle’s movement, using commands for drawing shapes, animations, and interactive applications. The turtle module, included in the Python library, offers a user-friendly interface, requires no additional installations, and is suitable for beginners and young students.
Importance of animations in programming
Animations enhance user experience and interactivity in programming by enhancing game, simulation, and data representation. Animations effectively communicate complex ideas, capture attention, and create memorable experiences, making applications dynamic and visually appealing.
So, let’s dive in and embark on this exciting journey of creating a simple animation using Python’s Turtle module. Get ready to unleash your creativity and bring your ideas to life through the power of animation!
Python Program To Create a Simple Animation Using Turtle
Approach:
- Import the required modules
- Set up the turtle window and the race track
- Create the turtle objects for each player
- Animate the turtles
- Run the animation
Learn How to Generate Random Numbers in Python, Now!
#Python program to create a simple animation using turtle # import the reqd modules from turtle import * from random import randint # Original turtle shaped speed(0.1) penup() goto(-140, 140) # the race track for j in range(15): write(j, align ='center') right(90) for num in range(8): penup() forward(10) pendown() forward(10) penup() backward(160) left(90) forward(20) # details of the first player plyr1 = Turtle() plyr1.color('magenta') plyr1.shape('turtle') # Proceedings of the first player to the race track plyr1.penup() plyr1.goto(-160, 100) plyr1.pendown() # Turning 360 degrees on the spot for turn in range(10): plyr1.right(36) # details of the second player plyr2 = Turtle() plyr2.color('red') plyr2.shape('turtle') # Proceedings of the second player to the race track plyr2.penup() plyr2.goto(-160, 70) plyr2.pendown() # Turning 360 degrees on the spot for turn in range(72): plyr2.left(5) # details of the third player plyr3 = Turtle() plyr3.shape('turtle') plyr3.color('light green') # Proceedings of the third player to the race track plyr3.penup() plyr3.goto(-160, 40) plyr3.pendown() # Turning 360 degrees on the spot for turn in range(60): plyr3.right(6) # details of the fourth player plyr4 = Turtle() plyr4.shape('turtle') plyr4.color('blue') # Proceedings of the third player to the race track plyr4.penup() plyr4.goto(-160, 10) plyr4.pendown() # Turning 360 degrees on the spot for turn in range(30): plyr4.left(12) # turtles running at random speeds for turn in range(100): plyr1.forward(randint(1, 5)) plyr2.forward(randint(1, 5)) plyr3.forward(randint(1, 5)) plyr4.forward(randint(1, 5))
Get Complete Python Interview Questions and Answers, here!
Explanation of the code:
- The random and turtle libraries import the respective modules.
- The animation sets the turtles’ movement speed to 0.1, determining how fast they move.
- Using penup(), the pen is raised, and goto() is used to set the turtle’s starting location to (-140, 140).
- To make the race track, we use a loop that writes numbers, draws lines, and moves the pen to the next position.
- We create and named four turtles with different colors and shapes: plyr1, plyr2, plyr3, and plyr4.
- Penup() and goto() are used to position each turtle on the race track.
- To simulate a pre-race warm-up, the turtles turn.
- The turtles begin the race by looping. The forward() function is called with a random distance between 1 and 5, causing each turtle to move at a different speed.
In summary, the code generates a race animation using the Turtle module, featuring four unique turtles racing on a course.
Output:
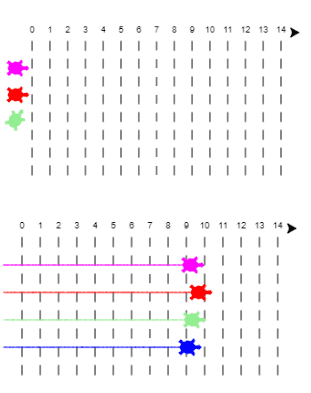
We hope our blog post on “Create a Simple Animation in Python Using Turtle” has been helpful to you. To continue enhancing your coding skills, visit our website Newtum and explore our online coding courses covering Java, HTML, PHP, and more. Keep learning with us!
FAQ about How to create simple animation in Python using turtle
Python developers create Turtle animation, a graphical representation using the Turtle module in Python, often leveraging it for educational purposes to visually teach programming concepts.
You can import the Turtle module in Python using the statement: from turtle import *.
Setting the animation speed determines how fast the Turtle graphics move, allowing you to control the pace of the animation.
The penup() and goto() functions raise the pen and move each turtle to its starting position on the race track.
The forward() function with a random distance parameter determines the speed of turtles in the animation, causing each turtle to move at different speeds.