Python Program to Draw Square Using Turtle
#import turtle import turtle t = turtle.Turtle() t.forward(100) t.right(90) t.forward(100) t.right(90) t.forward(100) t.right(90) t.forward(100) turtle.done()
Python is a high-level, general-purpose programming language that is widely used for web development, data analysis, artificial intelligence, and more. It is known for its simplicity, readability, and ease of use. Python has a vast collection of libraries and frameworks that make it easy to develop complex applications with minimal code. In this tutorial, we will learn how to draw a square in Python using the Turtle module.
Drawing a square in Python using Turtle is an excellent starting point for beginners who want to learn about graphics programming in Python. Here are a few reasons why:
- Simplicity: Drawing a square using Turtle is a simple and easy-to-understand process that requires minimal code. It is an excellent starting point for beginners who are just getting started with Python.
- Visualization: Drawing shapes using Turtle provides a visual representation of code, making it easy for beginners to understand how code works and how it affects the output.
- Turtle Metaphor: The Turtle module uses a turtle metaphor to enable users to draw shapes on the screen. It is a beginner-friendly tool that makes it easy for beginners to get started with graphics programming.
- Fundamental Concepts: Drawing shapes using Turtle introduces fundamental concepts such as loops, conditionals, and functions, which are essential for any programming language.
- Fun and Interactive: Drawing squares using Turtle is a fun and interactive way to learn about programming. It allows beginners to experiment with code and see the results in real time.
Starting with drawing a square in Python using Turtle is an excellent way for beginners to learn about graphics programming, understand fundamental concepts, and have fun while learning. It is a great way to build a solid foundation for more complex graphics programming projects.
Drawing shapes is a fundamental part of graphics programming, and Python’s Turtle module provides an easy way to draw shapes on the screen. The Turtle module is a beginner-friendly tool for graphics programming that uses a turtle metaphor to enable users to draw shapes on the screen. In this tutorial, we will learn how to draw a square in Python using the Turtle module.
Explanation
To draw a square in Python using Turtle, follow these steps:
1. Import the turtle module.
import turtle
2. Create a turtle object.
t = turtle.Turtle()
3. Use the forward() method to move the turtle forward, and the right() or left() method to turn the turtle.
t.forward(100) t.right(90) t.forward(100) t.right(90) t.forward(100) t.right(90) t.forward(100)
4. Use the done() method to keep the output window open.
turtle.done()
The code above creates a turtle object named “t”, uses the forward() method to move the turtle forward by 100 units, and then uses the right() method to turn the turtle by 90 degrees. It repeats this process four times, creating a square. Finally, it uses the done() method to keep the output window open.
Output:
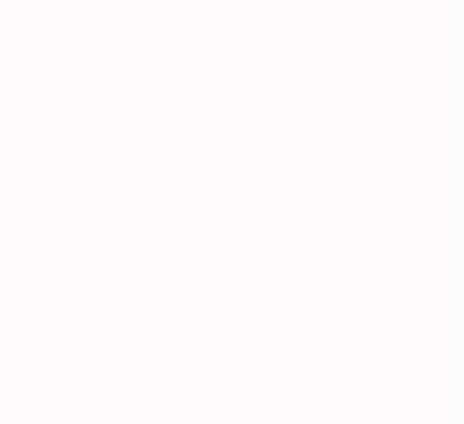
The output of the code is a square drawn using Python and the Turtle module. The square is drawn on a canvas, with the turtle moving forward and turning right to create the edges of the square. The code is simple and easy to understand, making it a great starting point for beginners who want to learn about graphics programming in Python.
In this tutorial, we learned how to draw a square in Python using the Turtle module. We explained each step of the code in detail, making it easy for beginners to understand. Drawing shapes using the Turtle module is a great way to learn about graphics programming in Python. We hope this tutorial was helpful and informative, and that you are now able to draw squares using Python and the Turtle module.