In this blog, we’ll teach you how to draw a rectangle in Python using Turtle graphics. Turtle graphics is like drawing with a virtual pen on your computer screen, making it easy for beginners to create shapes.
How to draw a rectangle in Python Using Turtle
below code of drawing rectangle pattern in python will help you to understand this concept in better way:
#import turtle import turtle t = turtle.Turtle() t.forward(200) t.right(90) t.forward(100) t.right(90) t.forward(200) t.right(90) t.forward(100) turtle.done()
Drawing shapes is a fundamental part of graphics programming, and Python’s Turtle module provides an easy way to draw shapes on the screen. In this tutorial, we will learn how to draw a rectangle in Python using the Turtle module.
To draw a rectangle in Python using the Turtle module, you need to use the forward() method to move the turtle forward, and the right() or left() method to turn the turtle. By repeating these two steps, you can create a rectangle with the desired dimensions. Additionally, you can use loops to simplify the process and make the code more concise.
Ready to explore more? Learn how to draw a circle in Python using turtle next!
Explanation
The given Python code uses the Turtle module to draw a rectangle. Here’s the explanation:
1. Importing the Turtle Module: The line import turtle
imports the Turtle module, which provides a simple way to draw graphics.
2. Creating a Turtle Object: The line t = turtle.Turtle()
creates a Turtle object named t
, which will be used to draw on the screen.
3. Drawing the Rectangle:
– t.forward(200)
: Moves the turtle forward by 200 units to draw the top side of the rectangle.
– t.right(90)
: Turns the turtle to the right by 90 degrees to change direction.
– t.forward(100)
: Moves the turtle forward by 100 units to draw the right side of the rectangle.
– t.right(90)
: Turns the turtle to the right by 90 degrees again.
– t.forward(200)
: Moves the turtle forward by 200 units to draw the bottom side of the rectangle.
– t.right(90)
: Turns the turtle to the right by 90 degrees.
– t.forward(100)
: Moves the turtle forward by 100 units to draw the left side of the rectangle.
4. Closing the Window: The line turtle.done()
keeps the window open until it is manually closed by the user.
This code uses the Turtle module’s methods to draw each side of the rectangle by moving the turtle forward and turning it at right angles. Finally, it closes the window to display the drawn rectangle.
Feeling creative? Try to draw Radha Krishna in Python using Turtle!
Output:
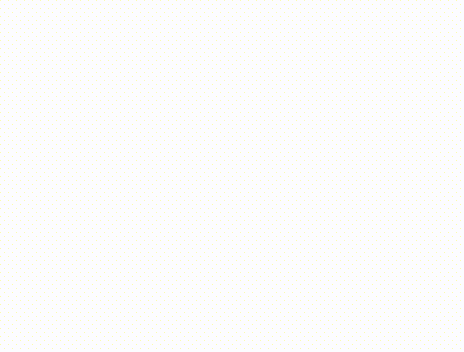
The output of the code is a rectangle drawn using Python and the Turtle module. The rectangle is drawn on a canvas, with the turtle moving forward and turning right to create the edges of the rectangle. The code is simple and easy to understand, making it a great starting point for beginners who want to learn about graphics programming in Python.
What are the other methods to Draw a rectangle in Python?
There are other methods to draw a rectangle in Python besides using the Turtle module. Here are a few examples:
- Using the graphics module: The graphics module is a simple graphics library that provides a way to create basic shapes such as rectangles, circles, and lines. To draw a rectangle using the graphics module, you can use the Rectangle class and the GraphWin object.
- Using the Pygame module: The Pygame module is a popular graphics library that provides a way to create complex games and animations using Python. To draw a rectangle in Python using Pygame, you can use the Rect class and the pygame.draw.rect() function.
Want to bring your favorite anime character to life? Learn how to draw Naruto in Python Using Turtle!
There are multiple ways to draw a rectangle in Python, each with its own advantages and disadvantages. The Turtle module provides a simple and beginner-friendly way to create shapes, while other libraries such as graphics and Pygame provide more advanced features for creating complex graphics and animations.
In this tutorial, we learned how to draw a rectangle in Python using the Turtle module. We explained each step of the code in detail, making it easy for beginners to understand. Drawing shapes using the Turtle module is a great way to learn about graphics programming in Python. We hope this tutorial was helpful and informative, and that you are now able to draw a rectangle in Python and the Turtle module.
FAQ about How to Draw a rectangle in Python using Turtle
Turtle graphics is a module in Python that allows users to create drawings and shapes using a turtle that moves around the screen.
To draw a rectangle in Python using Turtle, you can use the forward() method to move the turtle forward and the right() method to turn it.
Turtle graphics is beneficial for beginners as it provides a visual representation of code execution, making it easier to understand programming concepts.
Yes, besides rectangles, you can draw various shapes such as circles, triangles, and polygons using Turtle graphics in Python.
Besides using Turtle graphics, you can draw rectangles using other modules like graphics or Pygame in Python.
You can use the done() method from the Turtle module to keep the output window open after drawing a rectangle.