Python Program to Draw a Indian Flag Using Turtle
# Draw Indian Flag in Python using Turtle # import turtle import turtle from turtle import * # screen for output screen = turtle.Screen() # Defining a turtle Instance t = turtle.Turtle() speed(1) # initially penup() t.penup() t.goto(-200, 125) t.pendown() # Orange Rectangle #white rectangle t.color("orange") t.begin_fill() t.forward(400) t.right(90) t.forward(84) t.right(90) t.forward(400) t.end_fill() t.left(90) t.forward(84) # Green Rectangle t.color("green") t.begin_fill() t.forward(84) t.left(90) t.forward(400) t.left(90) t.forward(84) t.end_fill() # Big Blue Circle t.penup() t.goto(35, 0) t.pendown() t.color("navy") t.begin_fill() t.circle(35) t.end_fill() # Big White Circle t.penup() t.goto(30, 0) t.pendown() t.color("white") t.begin_fill() t.circle(30) t.end_fill() #Mini Blue Circles of Flag t.penup() t.goto(-27, -4) t.pendown() t.color("navy") for i in range(24): t.begin_fill() t.circle(2) t.end_fill() t.penup() t.forward(7) t.right(15) t.pendown() # Small Blue Circle t.color("navy") t.penup() t.goto(10, 0) t.pendown() t.begin_fill() t.circle(10) t.end_fill() #The spokes of India Flag t.penup() t.goto(0, 0) t.pendown() t.pensize(1) for i in range(24): t.forward(30) t.backward(30) t.left(15) #for stick of the flag t.color("Brown") t.pensize(10) t.penup() t.goto(-200,125) t.right(180) t.pendown() t.forward(800) #to hold the #output window turtle.done()
Introduction
Python is a popular programming language that is widely used for web development, scientific computing, data analysis, artificial intelligence, and more. It is a versatile language that is easy to learn and read, making it a great choice for beginners and experts alike. In this tutorial, we will learn how to draw the Indian flag using Python and the Turtle module.
The Indian flag is a symbol of the country’s independence and national pride. It consists of three horizontal stripes of saffron, white, and green, with a blue wheel in the center. Drawing the Indian flag in Python using the Turtle module is a great way to learn about graphics programming and the use of loops and methods in Python.
Explanation
The code above demonstrates how to draw the Indian flag using Python and the Turtle module. Here is a detailed step-by-step explanation of the code:
1. First, we import the turtle module and the turtle class.
import turtle from turtle import
2. We create a turtle object named “t” and set the speed to 1.
t = turtle.Turtle() speed(1)
3. We define the turtle screen object and create a screen to display the output.
screen = turtle.Screen()
We use the penup() method to lift the pen off the canvas and goto() method to move the turtle to the starting position.
t.penup() t.goto(-200, 125) t.pendown()
4. We use the color() method to set the color and begin_fill() method to start filling the rectangle.
t.color("orange") t.begin_fill()
5. We use the forward() and right() methods to draw the rectangle and end_fill() method to fill the color.
t.forward(400) t.right(90) t.forward(84) t.right(90) t.forward(400) t.end_fill()
We repeat steps 5 and 6 to draw the green rectangle.
t.color("green") t.begin_fill() t.forward(84) t.left(90) t.forward(400) t.left(90) t.forward(84) t.end_fill()
6. We use the penup() method to lift the pen off the canvas and goto() method to move the turtle to the starting position of the big blue circle.
t.penup() t.goto(35, 0) t.pendown()
7. We use the color() method to set the color and begin_fill() method to start filling the blue circle.
t.color("navy") t.begin_fill()
8. We use the circle() method to draw the big blue circle and end_fill() method to fill the color.
t.circle(35) t.end_fill()
9. We repeat steps 8-10 to draw the big white circle.
t.penup() t.goto(30, 0) t.pendown() t.color("white") t.begin_fill() t.circle(30) t.end_fill()
10. We use the penup() method to lift the pen off the canvas and goto() method to move the turtle to the starting position of the mini blue circles.
t.penup() t.goto(-27, -4) t.pendown() t.color("navy")
11. We use the for loop to draw the mini blue circles and use the penup() and pendown() methods to lift and lower the pen between circles.
for i in range(24): t.begin_fill() t.circle(2) t.end_fill() t.penup() t.forward(7) t.right(15) t.pendown()
12. We use the penup() method to lift the pen off the canvas and goto() method to move the turtle to the starting position of the small blue circle.
t.penup() t.goto(10, 0) t.pendown() t.color("navy")
13. We use the begin_fill() and end_fill() methods to fill the small blue circle.
t.begin_fill() t.circle(10) t.end_fill()
14. We use the penup() method to lift the pen off the canvas and goto() method to move the turtle to the starting position of the spokes of the flag.
t.penup() t.goto(0, 0) t.pendown()
15. We use the for loop to draw the spokes of the flag and use the forward(), backward(), and left() methods to move the turtle.
for i in range(24): t.forward(30) t.backward(30) t.left(15)
16. We use the color() method to set the color and pensize() method to set the size of the stick of the flag.
t.color("brown") t.pensize(10)
17. We use the penup() method to lift the pen off the canvas and goto() method to move the turtle to the starting position of the stick.
t.penup() t.goto(-200, 125) t.right(180) t.pendown() t.forward(800)
18. Finally, we use the done() method to keep the output window open.
turtle.done()
Output:
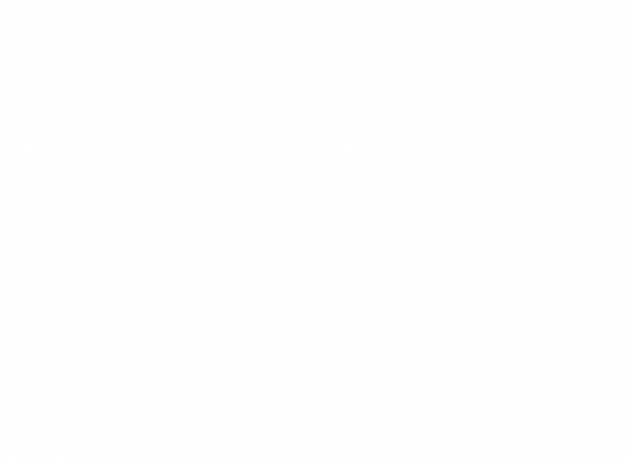
The output of the code is the Indian flag drawn using Python and the Turtle module. The flag consists of three horizontal stripes of saffron, white, and green, with a blue wheel in the center. The code is written in a step-by-step manner, making it easy to understand and follow along. The use of loops and methods in the code demonstrates the power and versatility of the Python programming language.
Conclusion
In this tutorial, we learned how to draw the Indian flag using Python and the Turtle module. We explained each step of the code in detail, making it easy for beginners to understand. Drawing the Indian flag in Python using the Turtle module is a great way to learn about graphics programming and the use of loops and methods in Python. We hope this tutorial was helpful and informative.