Python Program to Draw Spiraling Star Using Turtle
import turtle import random #Module for choosing random colors spiral = turtle.Turtle() colours = ["red", "blue", "black"] #List of colors to use randomly for i in range(40): spiral.color(random.choice(colours)) #Choosing the random colors from the colours list spiral.forward(i * 10) spiral.right(144) turtle.done()
Introduction
Python is a popular high-level programming language known for its simplicity and ease of use. The turtle module finds extensive application in numerous fields, including web development, data science, machine learning, and various other domains.Python has a vast collection of libraries and modules that make it easy for developers to create complex applications with ease.
In this tutorial, we will learn how to draw a spiraling star in Python using a turtle. The turtle module in Python provides a simple way to create graphics and shapes using a virtual turtle. We will be using the turtle module to create a spiraling star consisting of multiple colors.
Explanation
1. Let’s start by importing the turtle module and creating a turtle object named “spiral”. We will also import the random module for choosing random colors and create a list of colors to use randomly.
import turtle import random spiral = turtle.Turtle() colours = ["red", "blue", "black"]
2. Next, we will use a for loop to draw the spiraling star. The for loop will iterate 40 times, and with each iteration, the turtle will choose a random color from the colors list, move forward by a distance equal to the current iteration number multiplied by 10, and turn right by 144 degrees.
for i in range(40): spiral.color(random.choice(colours)) spiral.forward(i * 10) spiral.right(144)
The above code will draw lines using different colors, creating a spiraling star. After drawing each line, the turtle will turn right by 144 degrees before drawing the next line. This will create a star-like effect and make the design more interesting. The random.choice() function is used to choose a random color from the colors list, making the design more colorful and dynamic.
Output:
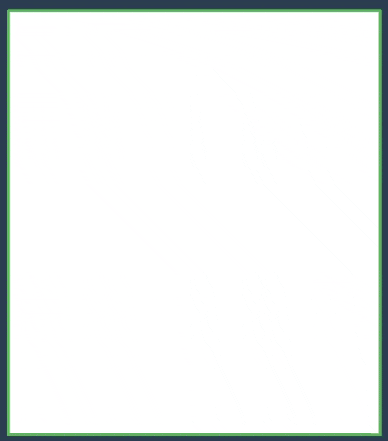
When executing the above code, a turtle graphics window will open, displaying a spiraling star drawn at the center of the screen. The spiraling star will consist of multiple lines, each line being a different color. The star will have a spiral-like effect, with each line being slightly different from the previous one. The random colors will make the design more colorful and dynamic.
Drawing a spiraling star in Python using turtle has various practical and creative applications. Some of the common applications of drawing spiraling stars using the turtle module are:
- Art and Graphics: Drawing spiraling stars using the turtle module can be used in various art and graphic projects, such as creating logos, posters, or illustrations. The flexibility of the turtle module allows for creating intricate and complex designs, making it an excellent tool for graphic designers and artists.
- Education and Science: Drawing spiraling stars using the turtle module can be used in educational settings to teach geometry, trigonometry, and other mathematical concepts. The spiraling star can serve as an educational tool for teaching concepts such as angles, degrees, and rotations. The visual representation of the spiraling star can help students understand the concepts better and make learning more engaging.
- Games: Spiraling stars can be incorporated into game development to create visually appealing and engaging games. The turtle module can be used to create game elements such as characters, backgrounds, and animations. The spiraling star created using turtle graphics can serve as game elements, such as power-ups, bonuses, or collectibles.
Conclusion
In this tutorial, we learned how to draw a spiraling star in Python using turtle. We used the turtle module to create graphics and shapes using a virtual turtle. We also learned how to use the random module to choose random colors from a list. With a little creativity, you can create all sorts of shapes and graphics using the turtle module in Python. The turtle module is an excellent tool for beginners to learn the basics of programming and computer graphics.