Python Program to Draw Circular Design Using Turtle
import turtle ninja = turtle.Turtle() ninja.speed(10) for i in range(180): ninja.color("red") ninja.forward(100) ninja.right(30) ninja.color("blue") ninja.forward(20) ninja.left(60) ninja.color("green") ninja.forward(50) ninja.right(30) ninja.penup() ninja.setposition(0, 0) ninja.pendown() ninja.right(2) turtle.done()
Introduction
Python is a popular high-level programming language known for its simplicity and ease of use. It is widely used in various fields, such as web development, data science, machine learning, and more. Python has a vast collection of libraries and modules that make it easy for developers to create complex applications with ease.
In this tutorial, we will learn how to draw a circular design in Python using turtle. The turtle module in Python provides a simple way to create graphics and shapes using a virtual turtle. We will be using the turtle module to create a circular design consisting of multiple colors and shapes.
Explanation
1. Let’s start by importing the turtle module and creating a turtle object named “ninja”. We will also set the speed of the turtle to 10 so that the design is drawn quickly.
import turtle ninja = turtle.Turtle() ninja.speed(10)
2. Next, we will use a for loop to draw the circular design. The for loop will iterate 180 times, and with each iteration, the turtle will move forward by 100 pixels, turn right by 30 degrees, move forward by 20 pixels, turn left by 60 degrees, move forward by 50 pixels, and then turn right by 30 degrees.
for i in range(180): ninja.color("red") ninja.forward(100) ninja.right(30) ninja.color("blue") ninja.forward(20) ninja.left(60) ninja.color("green") ninja.forward(50) ninja.right(30) ninja.penup() ninja.setposition(0, 0) ninja.pendown() ninja.right(2)
The above code will draw lines and shapes using different colors, creating a circular design. After drawing each shape, the turtle will reset its position to the center of the screen and turn right by 2 degrees before drawing the next shape. This will create a spiral-like effect and make the design more interesting.
Output:
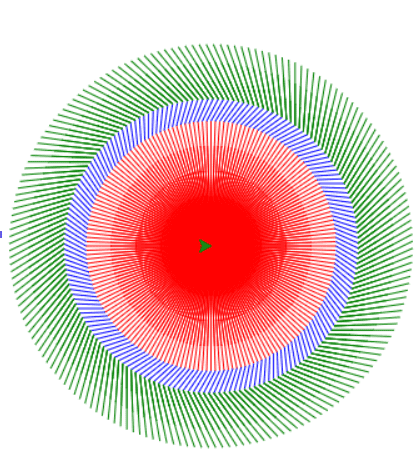
When you run the above code, a turtle graphics window will be opened with a circular design drawn in the center of the screen. The circular design will consist of multiple shapes and colors, creating a beautiful and intricate pattern. The design will have a spiral-like effect, with each shape being slightly different from the previous one.
Drawing circular designs using turtle in Python can have various practical and creative applications. Some of the common uses of drawing circular designs using the turtle module are:
- Art and Graphics: Circular designs drawn using the turtle module can be used in various art and graphic projects, such as creating logos, posters, or illustrations. The flexibility of the turtle module allows for creating intricate and complex designs, making it an excellent tool for graphic designers and artists.
- Educational Purposes: Drawing circular designs using the turtle module can be used in educational settings to teach geometry, trigonometry, and other mathematical concepts. The visual representation of the circular designs can help students understand the concepts better and make learning more engaging.
- Games: Circular designs can be incorporated into game development to create visually appealing and engaging games. The turtle module can be used to create game elements such as characters, backgrounds, and animations.
- Web Development: Circular designs can be used in web development to create visually stunning websites and user interfaces. The turtle module can be used to create graphics and animations that can be integrated into web development projects.
- Engineering and Architecture: Circular designs can be used in engineering and architecture to create blueprints and designs for various structures. The turtle module can be used to create 2D designs that can be used as a base for creating 3D models.
Conclusion
In this tutorial, we learned how to draw a circular design in Python using turtle. We used the turtle module to create graphics and shapes using a virtual turtle. We also learned how to use a for loop to repeat a set of instructions multiple times. With a little creativity, you can create all sorts of shapes and graphics using the turtle module in Python.
Happy Coding!!