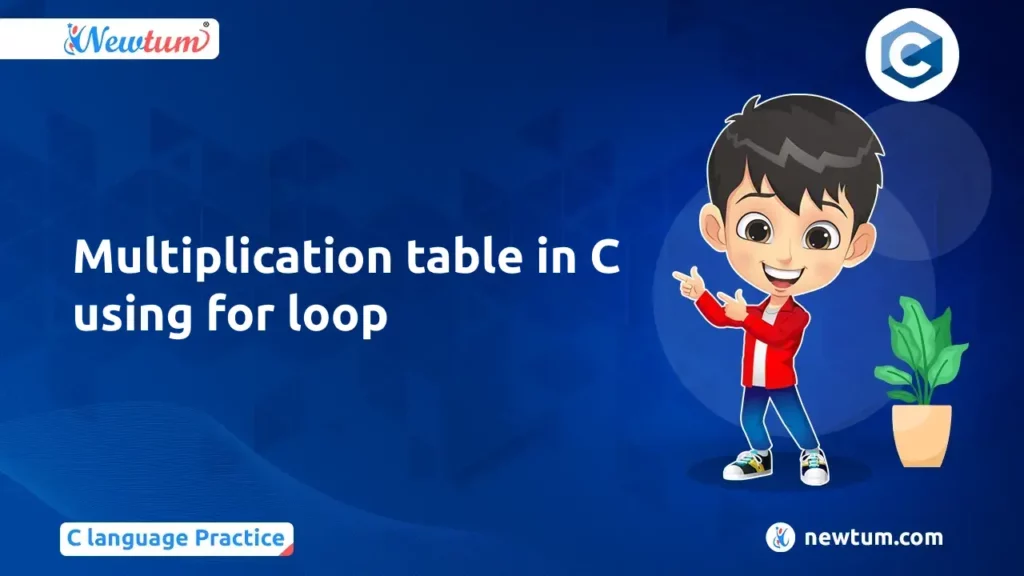
In this blog, we’ll explore how to generate multiplication tables in C using a for loop, providing a concise and efficient method to accomplish this task.
What are Multiplication Table in C using for Loop?
A multiplication table in C using a for loop is a program that generates and displays the multiplication table for a given number. It utilizes a for loop to iterate through a specified range of values (typically from 1 to 10) and computes the product of the given number with each value in the range. The resulting multiplication operations are then printed in a formatted manner to represent the multiplication table. This approach offers a concise and efficient method to generate multiplication tables in C programming, aiding in mathematical understanding and problem-solving skills.
Multiplication Table Program in C Using For Loop
The code for the C Program to generate Multiplication Table is given below:
// Multiplication table in C using for loop #include <stdio.h> int main(){ int n, i; printf("Enter a number: "); scanf("%d", &n); printf("Multiplication table of %d:\n ", n); for (i = 1; i <= 10; i++) printf("%d x %d = %d\n", n, i, n * i); return 0; }
Explanation of the code-
This C program generates a multiplication table for a specified number entered by the user using a for loop. Here’s an explanation of the code:
1. User Input: The program prompts the user to enter a number for which they want to generate the multiplication table using `printf()` function. The entered value is stored in the variable `n` using `scanf()` function.
2. For Loop: The program utilizes a `for` loop to iterate from 1 to 10.
Within the loop:
- The multiplication operation (`n * i`) is computed for each value of `i`.
- The result of the multiplication operation is printed in the format “n x i = n*i” using `printf()` function.
Output:
The multiplication table for the specified number is displayed in the console.
Enter a number: 24
Multiplication table of 24:
24 x 1 = 24
24 x 2 = 48
24 x 3 = 72
24 x 4 = 96
24 x 5 = 120
24 x 6 = 144
24 x 7 = 168
24 x 8 = 192
24 x 9 = 216
24 x 10 = 240
4. Return Statement: The `main()` function returns 0, indicating successful execution of the program.
This code effectively generates a multiplication table for a given number using a for loop, providing a clear and concise representation of the multiplication operations.
Real-world Applications and Extensions
Know the real-world applications and extensions of multiplication tables in C using for Loop given below:
A. Incorporating into Larger C Programs:
- Financial Applications: Calculate compound interest or amortization schedules by incorporating multiplication tables within financial software.
- Scientific Simulations: Implement algorithms that require repetitive mathematical computations, such as simulations in physics or chemistry.
- Data Analysis: Generate datasets with simulated data for testing algorithms or models in data analysis projects.
B. Educational Purposes:
- Interactive Learning Tools: Develop educational software to help students practice multiplication tables interactively, aiding in mathematical skill development.
- Classroom Activities: Design games or exercises that reinforce multiplication skills, enhancing engagement and understanding in the classroom.
- Curriculum Integration: Incorporate multiplication tables into lesson plans across various subjects like mathematics, science, and computer science to strengthen fundamental skills.
C. Exploring Other Applications in Programming:
- Pattern Recognition: Utilize multiplication tables to identify patterns and relationships in datasets or algorithms, aiding in data analysis and optimization.
- Algorithm Optimization: Leverage precomputed multiplication tables to optimize algorithms and reduce computational overhead in performance-critical applications.
- Code Optimization: Replace repetitive multiplication operations with table lookups for improved performance, especially in resource-constrained environments or embedded systems.
Mastering multiplication tables in C programming enhances functionality and efficiency in various domains, highlighting its versatility and importance in real-world applications like finance, science, education, and programming.
We hope our blog on “Multiplication table in C using for loop” helps you in strengthen your programming logic and mathematical skills and enhances problem-solving abilities and proficiency across various fields. Explore our courses on Newtum today for continuous growth and mastery.