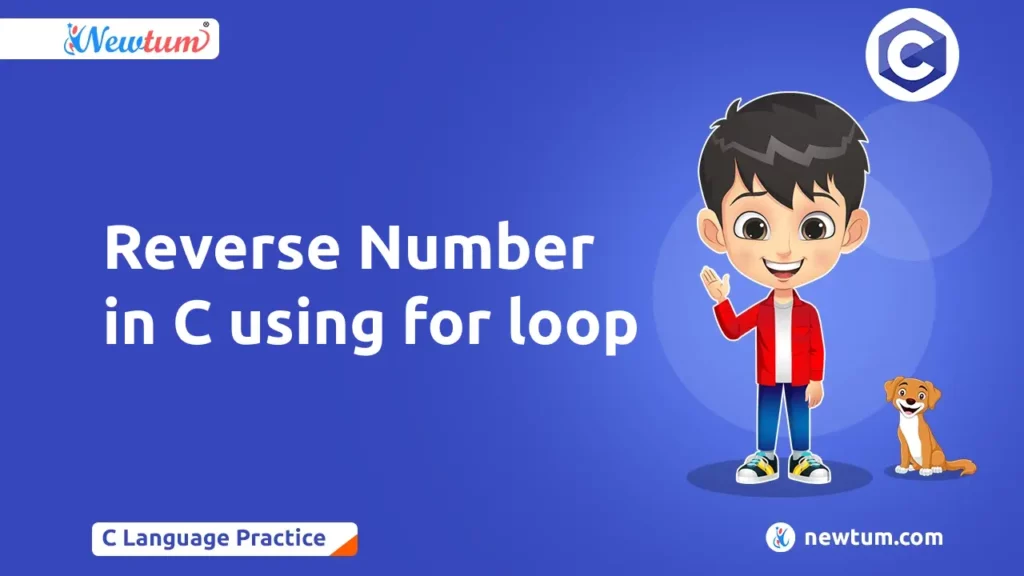
In this blog, we will learn how to reverse number in C using a for loop. From the basic concept of reverse number to other factors related to the topic. we will be understanding how to reverse numbers is a fundamental skill in programming, and mastering it opens up opportunities for tackling more complex problems.
How to reverse a number in C?
Reverse a number means to rearrange the number in the opposite order. In C programming, we can achieve this using a variety of techniques, one of which involves using a for loop for iterative reversal.
Learn How to Reverse a Number in Python Using Recursion Here!
C programming to reverse a number using for loop – Code
#include <stdio.h> int main() { int num, reversedNum = 0, remainder; // Input the number from the user printf("Enter an integer: "); scanf("%d", &num); // Reversing the number using a for loop for (; num != 0; num /= 10) { remainder = num % 10; reversedNum = reversedNum * 10 + remainder; } // Print the reversed number printf("Reversed number: %d\n", reversedNum); return 0; }
Explanation of the code:
This C program is designed to reverse a given integer entered by the user. Here’s how it works:
1. Input: The program prompts the user to enter an integer using `printf()` and `scanf()` functions.
2. Reversal: Inside the for loop, the number is reversed by repeatedly extracting the last digit of the number using the modulus operator `%`, and adding it to the `reversedNum` variable after multiplying `reversedNum` by 10. This process continues until the original number becomes zero.
3. Printing: Finally, the program prints the reversed number using `printf()`.
4. Loop Termination: The loop continues until `num` becomes zero, ensuring that all digits of the original number are processed.
Output:
Enter an integer: 123456789
Reversed number: 987654321
Check out our blog on Reverse a Number in Python Using String Slicing here!
Applications of Reverse Number in Real-world Scenarios
We’ll discuss common errors that may arise during implementation and how to debug them effectively.
1. Cryptographic Operations:
Reversing numbers are frequently used in cryptography for encryption and decryption algorithms, serving as keys or seeds for generating cryptographic hashes or pseudorandom numbers, thereby enhancing security.
2. Data Validation and Verification:
Reversing numbers aids in data validation and verification processes, such as detecting errors during input or transmission of credit card or identification numbers.
3. Data Structures and Algorithms:
Reversing numbers is a crucial operation in data structures and algorithms, particularly in linked lists, stacks, and queues, which are vital in computer science and programming.
4. Numeric Palindrome Identification:
Palindromes, which read the same forwards and backwards, are used to identify valid dates, symmetric patterns, or numeric codes in systems where palindromes hold significance.
5. Numerical Analysis and Optimization:
Reverse numbers are utilized in numerical analysis for tasks like root finding and optimization, often used in algorithms like Newton’s method or gradient descent for convergent solutions.
6. Mathematical Operations and Conversions:
Reversing numbers aids in mathematical operations and conversions, such as converting binary to decimal and performing arithmetic operations on digits.
7. Text Processing and String Manipulation:
Reversing numbers is a crucial part of string manipulation operations in text processing tasks, particularly when it comes to formatting or parsing data in text-based files or documents.
8. Game Development and Puzzle Solving:
Reversing numbers is utilized in game development and puzzle-solving scenarios, enhancing game mechanics, puzzle challenges, and cryptographic puzzles for players to solve.
We hope that our blog on ‘reverse numbers in C using for loop’ offers many benefits. Try out the provided code on Newtum Compiler to practice and learn. Newtum also offers easy-to-follow tutorials and courses to help you improve your programming skills. Keep practicing and enjoy your coding journey!
Reverse number in C using for Loop – FAQ
Answer: A reverse number in C is the number obtained by rearranging the digits of a given integer in the opposite order.
Answer: Reversing numbers is essential for tasks like cryptography, data validation, and various algorithms, making it a fundamental skill in programming.
Answer: The code iterates through each digit of the input number using a for loop, extracting digits and appending them in reverse order to form the reversed number.
Answer: Applications include cryptography, data verification, mathematical operations, game development, and text processing.
Answer: You can use the code on the Newtum Compiler to execute and modify it, allowing for hands-on learning and experimentation.