Python Program to Draw Square Design Using Turtle
import turtle wn = turtle.Screen() wn.setup(400,600) wn.bgcolor("white") s = turtle.Turtle() for i in range(4): s.begin_fill() s.forward(100) s.left(90) s.pencolor("red") s.forward(100) s.fillcolor("red") s.end_fill() for i in range(4): s.begin_fill() s.forward(80) s.left(90) s.pencolor("green") s.forward(80) s.fillcolor("green") s.end_fill() for i in range(4): s.begin_fill() s.forward(60) s.left(90) s.pencolor("yellow") s.forward(60) s.fillcolor("yellow") s.end_fill() for i in range(4): s.begin_fill() s.forward(40) s.left(90) s.pencolor('brown') s.forward(40) s.fillcolor("brown") s.end_fill() for i in range(4): s.begin_fill() s.forward(20) s.left(90) s.pencolor("black") s.forward(20) s.fillcolor("black") s.end_fill() turtle.done()
Introduction
Python is a popular high-level programming language known for its simplicity and ease of use. It is widely used in various fields, such as web development, data science, machine learning, and more. Python has a vast collection of libraries and modules that make it easy for developers to create complex applications with ease.
In this tutorial, we will learn how to draw a square in a square design in Python using turtle. The turtle module in Python provides a simple way to create graphics and shapes using a virtual turtle. We will be using the turtle module to create a design consisting of multiple squares, each square having a different color.
Explanation
1. Let’s start by importing the turtle module and creating a turtle object named “s”. We will also set up the screen and set the background color to white.
import turtle wn = turtle.Screen() wn.setup(400,600) wn.bgcolor("white") s = turtle.Turtle()
2. Next, we will use a for loop to draw multiple squares, each with a different color. For each square, we will use the begin_fill() method to start filling the square with color, then move forward by a distance of 100 pixels, turn left by 90 degrees, set the pen color to a specific color, move forward by another 100 pixels, set the fill color to the same color as the pen color, and finally, use the end_fill() method to fill the square with color.
for i in range(4): s.begin_fill() s.forward(100) s.left(90) s.pencolor("red") s.forward(100) s.fillcolor("red") s.end_fill()
3. We will repeat the above code for drawing four squares, each with a different color, with the size of each square decreasing by 20 pixels.
for i in range(4): s.begin_fill() s.forward(80) s.left(90) s.pencolor("green") s.forward(80) s.fillcolor("green") s.end_fill() for i in range(4): s.begin_fill() s.forward(60) s.left(90) s.pencolor("yellow") s.forward(60) s.fillcolor("yellow") s.end_fill() for i in range(4): s.begin_fill() s.forward(40) s.left(90) s.pencolor('brown') s.forward(40) s.fillcolor("brown") s.end_fill() for i in range(4): s.begin_fill() s.forward(20) s.left(90) s.pencolor("black") s.forward(20) s.fillcolor("black") s.end_fill()
Output:
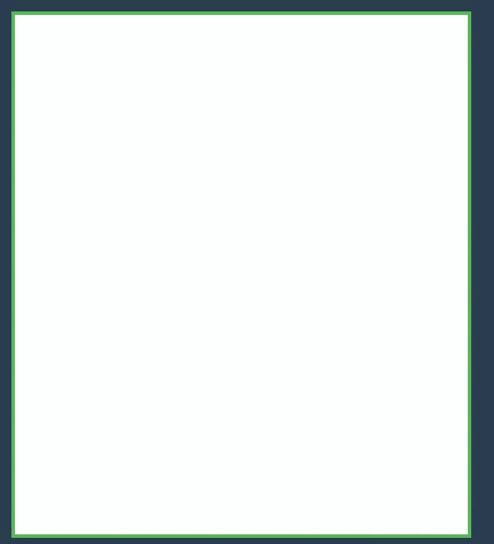
When you run the above code, a turtle graphics window will be opened with a square in a square design drawn in the center of the screen. The design will consist of multiple squares, each square having a different color. The size of each square will decrease by 20 pixels as we move from the outer square to the inner square. The use of different colors will make the design more colorful and visually appealing.
The square in square design created using the turtle module in Python has various practical and creative applications. Some of the common applications of drawing a square in square design using the turtle module are:
- Art and Graphics: The square in a square design in Python using turtle can be used in various art and graphic projects, such as creating logos, posters, or illustrations. The use of different colors and the size of each square decreasing as we move from the outer square to the inner square can create a visually appealing and dynamic design.
- Education and Science: Drawing a square in square design using the turtle module can be used in educational settings to teach geometry and mathematical concepts. The design can be used to teach concepts such as angles, degrees, and rotations. The visual representation of the square in square design can help students understand the concepts better and make learning more engaging.
- Games: The square in square design using turtle module can be incorporated into game development to create visually appealing and engaging games. The turtle module can be used to create game elements such as characters, backgrounds, and animations. The square in square design can be used to create game elements such as levels, obstacles, or patterns.
Conclusion
In this tutorial, we learned how to draw a square in a square design in Python using the turtle module. We used the turtle module to create graphics and shapes using a virtual turtle. We also learned how to use the begin_fill(), forward(), left(), pencolor(), fillcolor(), and end_fill() methods to draw and fill squares with different colors. With a little creativity, you can create all sorts of shapes and graphics using the turtle module in Python.