The Odd Even program in C# distinguishes between numbers divisible by 2 (even) and those leaving a remainder of 1 (odd). This foundational concept enhances programming skills by teaching basic arithmetic and conditional logic. Mastering this program sets the stage for more complex algorithms and improves problem-solving abilities in C#.In this blog, we’ll explore various aspect of the Odd Even Program in C#
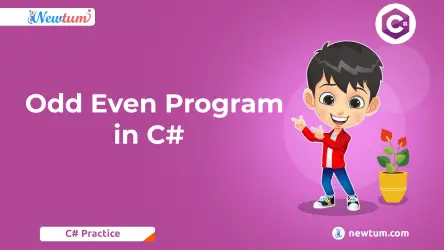
What are Odd and Even Numbers?
Odd and even numbers are fundamental concepts in mathematics and programming:
- Definition: Even numbers are divisible by 2 without leaving a remainder, while odd numbers leave a remainder of 1 when divided by 2.
- Modulus Operator (%): In C#, the modulus operator (%) returns the remainder of a division operation. It’s commonly used to check if a number is even or odd.
Setting Up C# Development Environment
Preparing your environment is essential for coding in C#:
- Installing Visual Studio: Download and install Visual Studio, a robust IDE for C# development.
- Configuring a C# Project: Create a new project in Visual Studio or Visual Studio Code, setting up necessary dependencies and configurations suitable for beginners.
Code of Odd Even Program in C#
// Odd even program in C# using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace Newtum { class oddEvenEx { static void Main(string[] args) { int num; Console.WriteLine("Enter the number to check odd or even"); num = int.Parse(Console.ReadLine()); if (num % 2 == 0) { Console.WriteLine("Number is Even"); } else { Console.WriteLine("Number is odd"); } Console.ReadKey(); } } }
Explanation of the Code:
- Namespace and Class Definition:
- namespace Newtum: Defines a namespace named Newtum to organize the classes.
- class oddEvenEx: Defines a class named oddEvenEx inside the Newtum namespace.
- Main Method (static void Main(string[] args)):
- Entry point of the program where execution begins.
- int num;: Declares an integer variable num to store the user input.
- User Input:
- Console.WriteLine(“Enter the number to check odd or even”);: Displays a prompt to enter a number.
- num = int.Parse(Console.ReadLine());: Reads the user input from the console as a string (Console.ReadLine()) and converts it to an integer (int.Parse()).
- Odd-Even Check:
- if (num % 2 == 0): Checks if the number num is even by using the modulus operator %. If num % 2 equals 0, the number is even (num is divisible by 2).
- Console.WriteLine(“Number is Even”);: Prints “Number is Even” to the console if the condition is true.
- Else Statement:
- else: Executes if the condition in the if statement is false (i.e., num is odd).
- Console.WriteLine(“Number is odd”);: Prints “Number is odd” to the console if the number is not even.
- Console.ReadKey():
- Pauses the program execution by waiting for the user to press any key before the console window closes. This keeps the console window open so the user can see the output.
Output:
Enter the number to check odd or even 895
Number is odd
Exploring Conditional Statements
Use of if…else Statement for Odd Even Check
The provided C# code snippet demonstrates the use of conditional statements to determine if a number is odd or even.
using System; namespace Newtum { class oddEvenEx { static void Main(string[] args) { int num; Console.WriteLine("Enter the number to check odd or even"); num = int.Parse(Console.ReadLine()); if (num % 2 == 0) { Console.WriteLine("Number is Even"); } else { Console.WriteLine("Number is odd"); } Console.ReadKey(); } } }
Explanation:
- Conditional Check (if…else statement):
- if (num % 2 == 0): Checks if the remainder of num divided by 2 is zero.
- If true, it means num is even, and it prints “Number is Even”.
- else: Executes if the condition (num % 2 == 0) is false.
- Prints “Number is odd” indicating that num is odd.
- if (num % 2 == 0): Checks if the remainder of num divided by 2 is zero.
Handling Edge Cases and User Input Validation
Edge Cases:
- The program does not handle edge cases explicitly, such as non-integer inputs or negative numbers. It assumes the user will enter a valid integer.
User Input Validation:
- int.Parse(Console.ReadLine()): Converts user input from string to integer. If the input cannot be parsed as an integer, it will throw a FormatException.
- Additional validation logic can be added to handle non-numeric inputs or negative numbers to provide a better user experience and prevent runtime errors.
Real-World Applications of Odd Even Checks
Beyond scheduling tasks, traffic management, and data partitioning in databases, the Odd Even logic in C# finds application in various other practical scenarios:
- Resource Allocation in Distributed Systems:
In distributed computing environments, resources such as processing nodes or storage units can be allocated based on odd or even criteria. This allocation strategy helps balance workload distribution and enhances system resilience by ensuring even utilization of resources across nodes.
- Game Development and Animation Frames:
Game developers use Odd Even logic in C# to manage animation frames efficiently. For instance, alternating frame updates can be synchronized with odd or even frame counts to create smooth animations and optimize rendering performance.
- Financial Transaction Processing:
Financial applications often partition transaction data based on odd or even criteria. C# programs can segregate transactions into separate batches or processes, ensuring that operations like auditing, reconciliation, or reporting are handled effectively and efficiently.
- User Interface and Interaction Design:
In C# applications, you can dynamically style or interact with user interface elements based on odd or even user interactions or system states.For example, alternating background colors or alternating behavior based on odd or even user actions can enhance user experience and usability.
- Load Balancing and Server Management:
C# applications in server environments can utilize Odd Even logic to distribute incoming requests across multiple servers or processes. By categorizing requests as odd or even, load balancing algorithms can evenly distribute traffic, optimize resource usage, and improve overall system performance.
In conclusion, this blog covered the implementation of the Odd Even Program in C#, highlighting its educational and practical value. Experimenting with such programs fosters hands-on learning. Explore more programming resources on Newtum, including blogs and courses in Java, HTML, C, and more. Happy coding!
Odd Even Program in C# – FAQs
The modulus operator (%) returns the remainder of a division operation, which helps in determining if a number is even (remainder 0) or odd (remainder 1).
The provided code does not handle non-integer inputs and will throw an exception if such input is entered.
The program will correctly identify negative numbers as odd or even based on their divisibility by 2.
It pauses the program execution, allowing the user to see the output before the console window closes.
By adding try-catch blocks and additional checks to handle non-numeric and out-of-range inputs, you can improve input validation.