In this tutorial, we are going to learn how to print Pascal Triangle in Python. There are different ways and programs that you can write to print a Pascal Triangle in Python. Let us explore these methods. Using the nCr Pascal Triangle Formula.
Python Program to Print Pascal Triangle
Here is what the implementation of this algorithm will look like.
n=int(input("Enter number of rows: ")) a=[] for i in range(n): a.append([]) a[i].append(1) for j in range(1,i): a[i].append(a[i-1][j-1]+a[i-1][j]) if(n!=0): a[i].append(1) for i in range(n): print(" "*(n-i),end=" ",sep=" ") for j in range(0,i+1): print('{0:6}'.format(a[i][j]),end=" ",sep=" ") print()
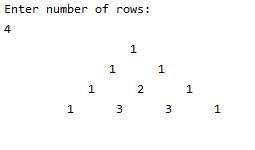
Watch your YouTube video next!
What is a Pascal Triangle? & It’s Formula
A Pascal’s Triangle is a triangular arrangement of numbers that gives you the coefficients for expanding any binomial expression. The most common applications of Pascal’s triangle include probability and algebra. So, if you were looking to find the probability of getting heads or tails when tossing a coin, you can use a Pascal Triangle to get your answer.
The key property of a Pascal Triangle is that the sum of each of the adjacent two numbers of the previous row is the number that lies just below in the second row. The Pascal Triangle is a triangular pattern based on the nCr formula. It can be mathematically represented as shown below:
Using the nCr formula
A combination is the selection of elements from a group of elements where the order of these elements does not matter. Suppose you have six books on your bookshelf and you want to find out how many different combinations are possible to select any 3 books from the six with the order of the books not mattering; you would use the formula nCr, i.e. 6C3 ways. You can further represent this using the Pascal Triangle.
Let us figure out the algorithm for this method. The following are some points to consider:
- Define the input for the program and assign it a variable (n).
- There are two iterations in the loop – the outer one and the inner one. Let us call the outer iteration i and the inner one j.
- The outer loop runs for n number of times while the inner one runs from o to n-1.
- We use “ “ to account for blank spaces, and // is used to close the loop.
- Every new line is marked off in the program using \n
Time Complexity with the O(N2) Formula
The second method to print a Pascal Triangle in Python is to follow the binomial coefficient concept. Here, the binomial coefficient for an ith entry in a line is C(line, i).
Let us figure out the algorithm behind this method. The key principle behind this method of printing a Pascal Triangle in Python is to calculate the value of the binomial coefficient using C(line, i-1). So, the underlying algorithm for this is as shown:
C(line, i) = C(line, i-1) * (line – i + 1)/i
Based on this algorithm, the implementation of this logic will be:
# input n n = int(input("Enter the number of rows:")) # iterarte upto n for i in range(n): # adjust space print(' '*(n-i), end='') # compute power of 11 print(' '.join(map(str, str(11**i))))
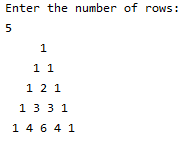