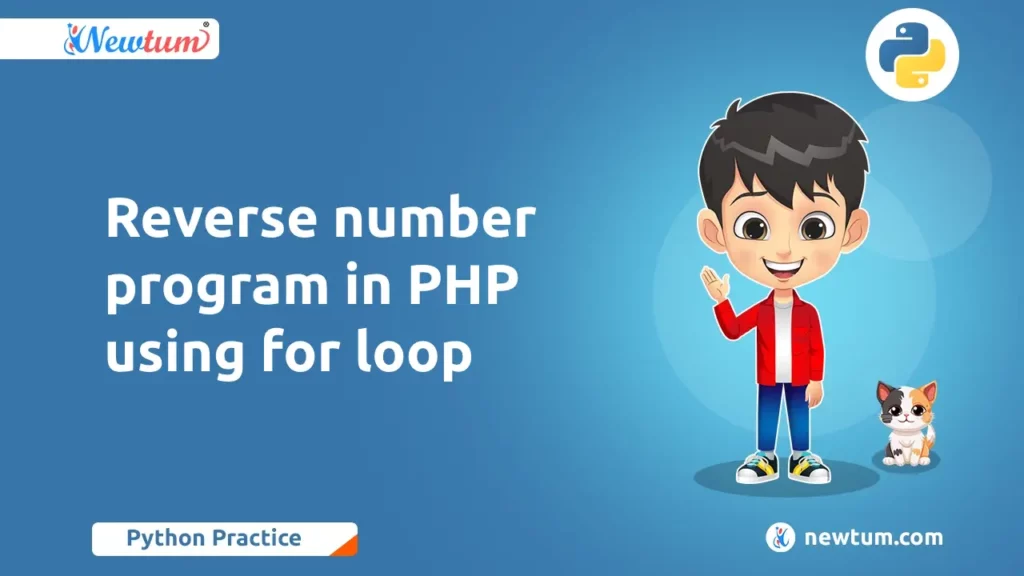
In the world of programming, reversing numbers is a common task that often arises in various applications. In this blog, we’ll delve into the concept of reverse number program in PHP using for loop. We’ll explore the step-by-step process, provide a sample code snippet, explain how it works, and discuss its practical applications.
Reverse number Program in PHP using a for loop – Algorithm
The algorithm for reversing numbers in PHP using a for loop involves several steps:
1. Initialization: Start by initializing an empty string to store the reversed number.
2. Convert to String: Convert the input number to a string to iterate through its digits.
3. Iterate Through Digits: Use a for loop to iterate through each digit of the input number in reverse order, starting from the last digit.
4. Append Digits: Within the loop, append each digit to the reversed number string.
5. Convert Back to Integer: Convert the reversed number string back to an integer.
Using loop control variables and array indexing allows us to access each digit of the input number efficiently, systematically facilitating the reversal process.
Code: Reverse number program in PHP using for Loop
<?php // Get user input echo "Enter a number: "; $userInput = fgets(STDIN); $number = (int)trim($userInput); // Convert input to integer and remove whitespace // Initialize variables for reversal $reversedNumber = 0; $numberOfDigits = strlen((string)$number); // Get number of digits (converted to string) // Reverse the number using a for loop for ($i = $numberOfDigits - 1; $i >= 0; $i--) { $lastDigit = $number % 10; $reversedNumber = $reversedNumber * 10 + $lastDigit; $number = (int)($number / 10); } // Print the result echo "The reversed number is: " . $reversedNumber . PHP_EOL; ?>
Explanation of Code:
- User Input: Prompts the user to enter a number. Reads the user input using fgets(STDIN). Converts the input to an integer using (int) and removes leading/trailing spaces with trim.
- Reverse Variables: Initializes reversedNumber to store the reversed number. numberOfDigits stores the number of digits in the original number. We achieve this by converting the number to a string using (string) and then using strlen to get the string length.
- For Loop: The loop iterates $numberOfDigits times, starting from the last digit position ($numberOfDigits – 1). In each iteration:
- Extracts the last digit using the modulo operator (%).
- Appends the last digit to the $reversedNumber number, multiplied by 10 to shift it to the appropriate position.
- Updates the original number by removing the last digit using integer division (/) and casting it to an integer ((int)) to remove any decimal part.
- Print Result: Prints the reversed number using string concatenation.
This approach uses a for loop to iterate through each digit of the number based on its length, achieving the reversal process.
Explore the fascinating world of Reverse number program in PHP using while loop, Now!
Output:
Original number: 13579
Reversed number: 97531
Reverse number in PHP using for loop- Practical Application
The practical applications of reversing numbers in PHP using a for loop are vast and varied, spanning across different fields:
1. Data Processing: In data analysis tasks, reversing numbers can help interpret data in reverse chronological or sequential order, aiding in trend analysis and historical data visualization.
2. Cryptography: Reversing numbers can be utilized in encoding and decoding algorithms, enhancing data security and confidentiality in cryptographic applications.
3. Mathematical Computations: Reverse numbers are essential in various mathematical computations and algorithmic challenges, facilitating efficient problem-solving in mathematical domains.
4. User Interface Design: Reversing numbers can enhance user interface elements like countdown timers, progress bars, and other interactive components, improving user experience and engagement.
5. Game Development: In game development, reversing numbers can be used for implementing game mechanics such as scoring systems, countdown timers, and level progression indicators, adding depth and complexity to gameplay.
Overall, the ability to reverse number program in PHP using a for loop finds extensive applications across diverse real-world scenarios.
Dive into the world of Javascript programming and master the Reverse number in javascript using for loop in a snap!
Reverse Number in PHP Using For Loop: Benefits
Using a for loop for reversing numbers in PHP offers several advantages over other methods:
1. Controlled Iteration: With a for loop, you have precise control over the number of iterations, making it suitable for tasks where a specific number of iterations is required.
2. Simplicity and Readability: The syntax of a for loop is concise and intuitive, making the code easier to understand and maintain compared to other looping constructs.
3. Efficiency: For loops are generally more efficient than other looping constructs like while and do-while loops, as they combine loop initialization, condition checking, and iteration in a single line.
4. Ease of Integration: For loops seamlessly integrate with other PHP code, making them versatile and suitable for a wide range of applications.
5. Performance: In many cases, for loops offer better performance compared to built-in functions for reversing numbers, especially when dealing with large datasets.
6. Flexibility: For loops allow for customization and adaptation to different scenarios, providing developers with greater flexibility in implementing complex algorithms or logic.
Overall, the for loop stands out as a reliable and efficient choice for reversing numbers in PHP, offering simplicity, readability, performance, and flexibility unmatched by other methods.
In conclusion, exploring the reverse number program in PHP using a for loop enhances problem-solving skills and understanding of loop constructs. Experimentation is key to mastering programming, and resources like Newtum offer comprehensive tutorials to support your learning journey. Keep coding and enjoy the endless possibilities of PHP programming!
Reverse Number Program in PHP using for Loop- FAQ
Answer: The reverse number program in PHP using for loop offers controlled iteration, simplicity, efficiency, and flexibility, making it a preferred choice for reversing numbers.
Answer: Using a for loop allows precise control over the number of iterations, ensuring efficient and customizable reversal of numbers in PHP.
Answer: You can simply copy and paste the provided sample code into your PHP project, ensuring proper variable initialization and loop control.
Answer: Yes, the sample code can be easily modified to reverse numbers with any number of digits by adjusting the loop control variable and initialization.
Answer: Yes, the for loop offers good performance even with large datasets, making it suitable for handling extensive numerical processing tasks efficiently.