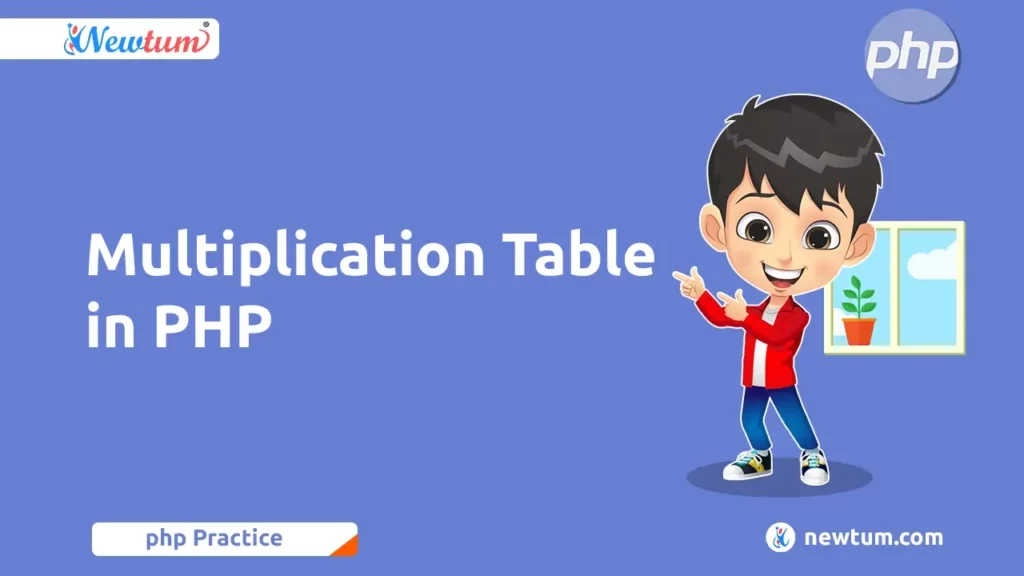
In this blog, we will explore creating a multiplication table in PHP, a server-side scripting language widely used for web development. Multiplication tables display the results of multiplying two numbers and are essential for understanding basic arithmetic operations.
Multiplication table in PHP- Code
A multiplication table in PHP is created using loops to generate a grid of multiplication results. It aids in learning arithmetic and programming concepts, making it a valuable educational tool. This code efficiently generates a multiplication table for the number 4 up to the 10th multiple in PHP:
<?php // Multiplication table in PHP $table = 4; $length = 10; $i = 1; echo "Multiplication table: $table \n"; for($i=1; $i<=$length; $i++) echo "$i * $table = ".$i * $table. "\n"; ?>
Explanation of the code:
Below is the explanation of the provided PHP code for generating a multiplication table:
1. Variable Initialization:
- `$table = 4;`: This line initializes a variable `$table` with the value 4, representing the number for which the multiplication table needs to be generated.
- `$length = 10;`: This line initializes a variable `$length` with the value 10, representing the length of the multiplication table.
2. Loop for Multiplication Table:
- `for($i=1; $i<=$length; $i++)`: This `for` loop iterates from 1 to the value of `$length`, which is 10 in this case.
- `echo “$i * $table = “.$i * $table. “\n”;`: Inside the loop, this line prints each multiplication expression, where `$i` represents the current iteration count, `$table` is the number for the multiplication table, and `$i * $table` calculates the product of the two numbers.
Output:
The output displays each multiplication expression on a new line, showing the multiplication table for the number 4 up to the 10th multiple:
Multiplication table: 4
1 * 4 = 4
2 * 4 = 8
3 * 4 = 12
4 * 4 = 16
5 * 4 = 20
6 * 4 = 24
7 * 4 = 28
8 * 4 = 32
9 * 4 = 36
10 * 4 = 40
Interactive Table Customization: Multiplication Table in PHP
A static multiplication table in PHP is informative, but what if we could make it interactive? Let’s explore how users can personalize their learning experience by providing input for various parameters.
User Input and Validation:
- Form and Input Fields: Create an HTML form with input fields for users to specify their desired table size, starting number, and range (if applicable).
- PHP Script: In your PHP script, use $_POST or $_GET(depending on the form submission method) to retrieve user-provided values.
- Validation: Implement basic validation to ensure valid input (e.g., checking for positive integers and handling non-numeric characters).
Customizing the Table based on Input:
- Table Size: Use the user-provided table size (number of rows and columns) as the loop conditions in nested for loops to determine the number of iterations and generate the appropriate table dimensions.
- Starting Number: Store the user’s chosen starting number in a variable. Modify the inner loop’s condition to iterate from the chosen starting number instead of the default 1.
- Specific Ranges: If users provide starting and ending values for rows and columns, adjust your loops to iterate within those specified ranges, displaying only the desired sub-section of the table.
Additional Customization Options:
- Formatting: Enhance the table’s appearance with CSS for spacing, alignment, and borders.
- Error Handling: Implement more robust error handling to catch various invalid user inputs and provide informative error messages.
- Advanced Features: Explore integrating user input with JavaScript for dynamic table generation and updates without full page reloads.
Integration with Database in Multiplication Table in PHP
Multiplication tables can be dynamically generated based on data stored in a database, offering flexibility and scalability in web applications. Here’s how this integration works:
1. Database Setup: Begin by creating a database schema to store the necessary data for generating multiplication tables. This schema typically includes tables to store user input parameters, such as table size and starting number, as well as the resulting multiplication table data.
2. Data Retrieval: Use server-side scripting languages like PHP to retrieve user input from the database and dynamically generate the multiplication table based on this data. SQL queries can be utilized to fetch relevant information from the database tables.
3. Dynamic Updates: With the integration in place, any changes to the user input parameters or underlying data in the database will reflect in real-time updates to the multiplication table displayed on the web application. This ensures that the table remains accurate and up-to-date.
4. Scalability: By storing multiplication table data in a database, web applications can handle large datasets efficiently and scale seamlessly as the application grows. This approach enables developers to accommodate diverse user needs and maintain performance across various usage scenarios.
In wrapping up, our journey through ‘Multiplication tables in PHP’ has highlighted how useful and important they are for learning and practical purposes. We urge you to try out the code we’ve provided on Newtum Compiler, where you can learn by doing. Explore Newtum‘s tutorials and courses to become a better coder. Happy Coding!
Multiplication table in PHP – FAQ
Answer: Yes, you can make it bigger or smaller based on what you need.
Answer: You just need to tell the program where to start counting from.
Answer: Absolutely, you can choose which part to display.
Answer: You can use a special trick with JavaScript to make it happen.
Answer: It helps handle big amounts of data more easily and makes the table work better.
Answer: Newtum has lessons and courses that can help you get better at it.