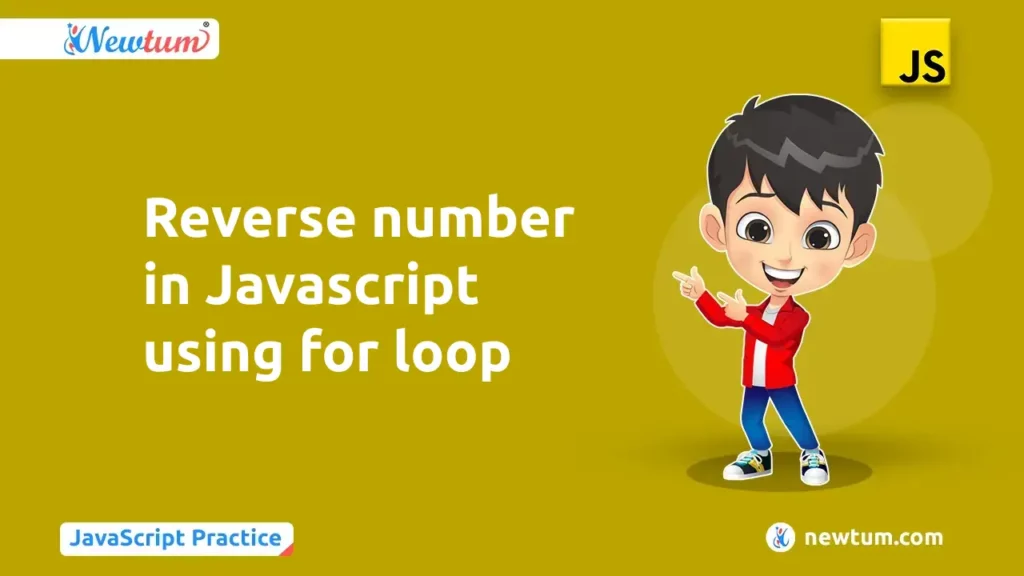
Our this blog is about ‘Reverse number in javascript using for loop where, we delve into the concept of reversing numbers. Reversing numbers holds significant importance in JavaScript programming, enabling tasks like data manipulation and algorithm design. We specifically focus on using for loops as a fundamental technique for number reversal, fostering a deeper understanding of JavaScript fundamentals.
Algorithm:
1. Get the input number from the user.
2. Initialize an empty string to store the reversed number.
3. Loop through the digits of the input number from the last digit to the first digit.
4. Append each digit to the reversed number string.
5. Display the reversed number on the webpage.
Sample code for Reverse number in Javascript using for loop:
<!DOCTYPE html> <html> <head> <title>Reverse Number</title> <style> body{ font-family: Arial, sans-serif; text-align: center; } </style> </head> <body> <h1>Reverse Number</h1> <form> <label for="number">Enter a number:</label> <input type="number" id="number" name="number"> <button type="button" onclick="reverseNumber()">Reverse</button> </form> <p id="result"></p> <script> // function to reverse the number function reverseNumber(){ // get the input value from the user let num = document.getElementById("number").value; // initialize an empty string to store the reversed number let reverse = ""; // loop through the number from the last digit to the first digit for(let i = num.length - 1; i >= 0; i--){ // add each digit to the reverse string reverse += num[i]; } // display the reversed number on the webpage document.getElementById("result").innerHTML = "Reversed number: " + reverse; } </script> </body> </html>
Discover more method Reverse number in Java using function and Reverse number in Java using while loop now!
Explanation of the Code:
The provided code is an HTML file containing the ‘Reverse number in JavaScript for loop’ number entered by the user:
1. The HTML structure includes a form with an input field to enter a number and a button to trigger the reversal process.
2. The JavaScript function `reverseNumber()` retrieves the input number.
3. It initializes an empty string `reverse` to store the reversed number.
4. It then iterates through the digits of the input number from the last digit to the first digit using a for loop.
5. In each iteration, it appends the current digit to the `reverse` string.
6. Finally, it updates the content of the paragraph element with the id “result” to display the reversed number on the webpage.
Output:
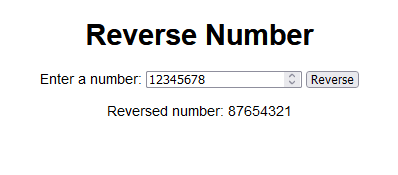
Performance Analysis and Optimization Techniques
Understand Performance Analysis and learn optimization techniques for the reverse number in Javascript using for loop:
A. Evaluating Time Complexity:
1. Analysis: Assess the time complexity of the algorithm, considering the number of iterations required for reversing a number.
2. For Loop Complexity: As the for loop iterates through each digit of the number once, the time complexity is O(n), where n is the number of digits in the input number.
B. Identifying Bottlenecks:
1. String Concatenation: Concatenating strings within the loop may lead to performance overhead, especially for large numbers.
2. String Length Calculation: Calculating the length of the input number string in each iteration can impact performance.
3. DOM Manipulation: Frequent DOM updates for displaying the reversed number may slow down the execution.
C. Optimization Strategies:
1. Preallocation of String: Instead of concatenating strings in each iteration, preallocate a fixed-size array and fill it with reversed digits.
2. Use StringBuilder: In JavaScript, use Array.prototype.push to append characters to an array and then join them using Array.prototype.join for better performance.
3. Avoid Redundant Calculations: Store the length of the input number string in a variable outside the loop to avoid redundant calculations.
4. Batch DOM Updates: Instead of updating the DOM in each iteration, batch the updates and perform them once after the loop completes.
By implementing these optimization strategies, the performance of the reverse number function can be significantly improved, enhancing the user experience and reducing computational overhead.
Check out our blog on Reverse number in javascript here!
Benefits and Applications
Check out below mentioned benefits and applications of Reverse Number in JavaScript Using For Loop
A. Enhanced Problem-Solving Skills:
1. Analytical Thinking: Reversing numbers using a for loop enhances analytical thinking and problem-solving abilities.
2. Algorithmic Understanding: Understanding the logic behind reversing numbers strengthens algorithmic understanding, aiding in tackling complex problems.
B. Real-World Applications:
1. Data Manipulation: Reversing numbers is crucial in data manipulation tasks such as sorting algorithms, where rearranging elements is necessary.
2. User Input Processing: In applications like form validation, reversing user input can be useful for verification and error checking.
C. Importance in Algorithm Design:
1. Pattern Recognition: Reversing numbers helps in recognizing patterns and relationships in data, essential for developing efficient algorithms.
2. Optimization Techniques: Understanding reverse number algorithms is foundational for optimizing other algorithms that involve numerical manipulation.
By recognizing the benefits and applications of reversing numbers in JavaScript using a for loop, developers can enhance their problem-solving skills, design more efficient algorithms, and apply these concepts to real-world scenarios effectively.
In this blog of ‘Reverse number in Javascript using for loop’, we explored the process of reversing numbers in JavaScript using for loop, emphasizing its educational and practical significance. Newtum offers a wide range of programming language tutorials and courses, catering to diverse learning needs. Keep coding, stay curious, and enjoy your journey of continuous learning and growth!
FAQs about Reverse Number in Javascript using for loop
Answer: Iterate through the digits of the number from the last digit to the first and append each digit to a new string to reverse the number.
Answer: The time complexity is O(n), where n is the number of digits in the input number.
Answer: Yes, the method works for negative numbers by treating them as strings and reversing their digits.
Answer: Optimize by preallocating a fixed-size array, avoiding redundant calculations, and batching DOM updates for better performance.
Answer: Yes, you can explore other methods like recursion or built-in JavaScript functions like split(), reverse(), and join().
Answer: While the method works for large numbers, performance may degrade due to string manipulation and DOM updates, impacting user experience.