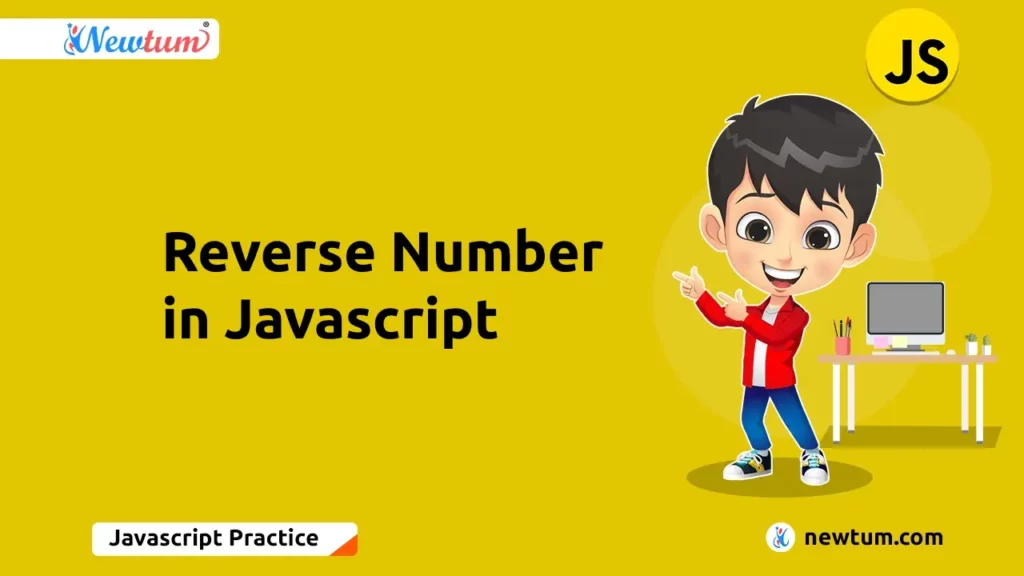
Reversing numbers is a fundamental operation in JavaScript programming, with various methods available to achieve this task. This blog ‘Reverse number in Javascript’ explores How to reverse number in Javascript with different techniques, highlighting their code implementation, explanation, and output demonstration.
Methods to reverse number in Javascript
Here are various techniques for reversing numbers in JavaScript, outlined below:
Table of Contents
Method 1: Reverse number in Javascript Using String Reverse
JavaScript provides a built-in method `reverse()` for arrays, which can also be applied to strings to reverse their order. Let’s delve into the code:
<!DOCTYPE html> <html> <head> <title>Reverse Number</title> <style> body{ font-family: Arial, sans-serif; text-align: center; } </style> </head> <body> <h1>Reverse Number</h1> <!-- create a form to take input from user --> <form> <label for="number">Enter a number:</label> <input type="number" id="number" name="number" required> <button type="submit">Reverse</button> </form> <script> // get the form element const form = document.querySelector('form'); // add event listener to the form on submit form.addEventListener('submit', (e) => { // prevent the default form submission e.preventDefault(); // get the input value from the form const number = document.getElementById('number').value; // convert the number to a string const stringNumber = number.toString(); // use the built-in reverse() method to reverse the string const reversedString = stringNumber.split('').reverse().join(''); // convert the reversed string back to a number const reversedNumber = parseInt(reversedString); // display the reversed number to the user alert(`The reversed number is: ${reversedNumber}`); }); </script> </body> </html>
Explanation of the code:
This HTML file contains a form to reverse a number entered by the user:
1. The `<form>` element allows users to input a number.
2. The `<script>` tag contains JavaScript code that listens for the form submission event.
3. When the form is submitted, the event listener prevents the default form submission behavior.
4. The input number is retrieved from the form and converted to a string.
5. The `split()`, `reverse()`, and `join()` methods are used to reverse the string.
6. The reversed string is then parsed into an integer using `parseInt()`.
7. Finally, an alert displays the reversed number to the user.
Discover more method Reverse number in Java using function and Reverse number in Java using while loop now!
Output:
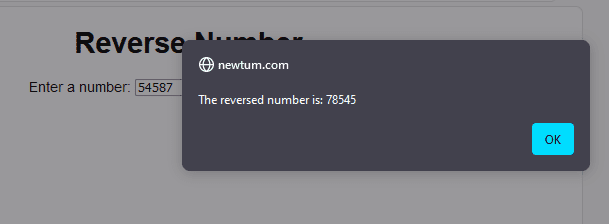
Method 2: Reverse number in Javascript Using Array Reduce() Method
Another approach involves utilizing the `reduce()` method of arrays to reverse the digits of a number. Let’s see how it’s implemented:
<!DOCTYPE html> <html> <head> <title>Reverse Number using Array Reduce() Method</title> </head> <body> <h1>Reverse Number</h1> <p>Enter a number to reverse:</p> <input type="number" id="numInput"> <button onclick="reverseNumber()">Reverse</button> <p id="result"></p> <script> // function to reverse the number function reverseNumber() { // get the input value from the user let num = document.getElementById("numInput").value; // convert the number to string and split it into an array of characters let numArray = num.toString().split(""); // use the reduce() method to reverse the array let reversedArray = numArray.reduce((acc, curr) => curr + acc, ""); // convert the reversed array back to a number let reversedNum = parseInt(reversedArray); // display the result on the webpage document.getElementById("result").innerHTML = "Reversed Number: " + reversedNum; } </script> </body> </html>
Explanation of the code:
This HTML file allows users to reverse a number using the Array `reduce()` method:
1. The `<input>` element allows users to input a number.
2. When the “Reverse” button is clicked, the `reverseNumber()` function is triggered.
3. Inside the function:
– The input number is retrieved from the input field.
– The number is converted to a string and split into an array of characters.
– The `reduce()` method is used to reverse the array by concatenating each element with the accumulator in reverse order.
– The reversed array is then parsed back into a number.
– Finally, the reversed number is displayed on the webpage.
Output:
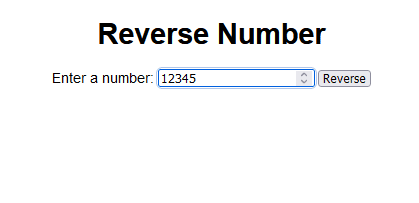
Reversed Number: 54321
Method 3: Reverse Number in Javascript Using String Iteration
In this method, we iterate over the string representation of the number and build the reversed string iteratively. Let’s examine the code:
<!DOCTYPE html> <html> <head> <title>Reverse Number</title> <style> body{ font-family: Arial, sans-serif; text-align: center; } </style> </head> <body> <h1>Reverse Number</h1> <!-- create a form to take input from user --> <form> <label for="number">Enter a number:</label> <input type="number" id="number" name="number" required> <button type="submit">Reverse</button> </form> <script> // get the form element var form = document.querySelector("form"); // add event listener to the form on submit form.addEventListener("submit", function(event){ // prevent the default form submission event.preventDefault(); // get the input value from the form var number = document.getElementById("number").value; // convert the number to a string var numberString = number.toString(); // create an empty string to store the reversed number var reversedNumber = ""; // loop through the string from the last character to the first for(var i = numberString.length - 1; i >= 0; i--){ // add each character to the reversed number string reversedNumber += numberString[i]; } // display the reversed number on the page document.write("<p>The reversed number is: " + reversedNumber + "</p>"); }); </script> </body> </html>
Explanation of the code:
This HTML file contains a form where users can input a number. When the form is submitted, the JavaScript code executes. Below is the explanation of the code:
1. The form submission event is captured using an event listener.
2. The default form submission behavior is prevented to handle the submission via JavaScript.
3. The input number is retrieved and converted to a string.
4. An empty string is initialized to store the reversed number.
5. Using a for loop, each character of the string is appended to the reversed number string in reverse order.
6. The reversed number is then displayed on the webpage using `document.write()`.
Output:
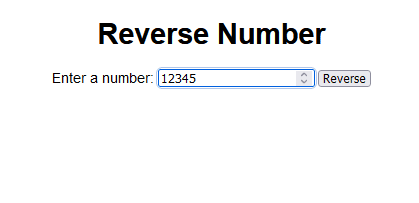
Reversed Number: 54321
Method 4: Using Recursion
Recursion offers an elegant solution for reversing numbers by breaking down the problem into smaller subproblems. Let’s explore the code:
<!DOCTYPE html> <html> <head> <title>Reverse Number Using Recursion</title> <style> body{ font-family: sans-serif; text-align: center; } h1{ margin-top: 50px; } input{ padding: 10px; font-size: 16px; } button{ padding: 10px 20px; font-size: 16px; margin-top: 20px; } </style> </head> <body> <h1>Reverse Number Using Recursion</h1> <!-- create a form to take input from user --> <form> <label for="number">Enter a number:</label> <input type="number" id="number" required> <button type="button" onclick="reverseNumber()">Reverse</button> </form> <!-- create a div to display the result --> <div id="result"></div> <script> // function to reverse the number using recursion function reverseNumber(){ // get the input value from user let num = document.getElementById("number").value; // call the recursive function and store the result in a variable let reversedNum = reverse(num); // display the result in the div document.getElementById("result").innerHTML = "Reversed number: " + reversedNum; } // recursive function to reverse the number function reverse(num){ // base case: if the number has only one digit, return the number if(num < 10){ return num; } // recursive case: call the function again with the number divided by 10 and add the remainder to the end else{ return (num % 10) + "" + reverse(Math.floor(num/10)); } } </script> </body> </html>
Explanation of the code:
This HTML file includes a form for users to input a number. When the form is submitted, the `reverseNumber()` function is called. Here’s the explanation:
1. The user inputs a number, triggering the `reverseNumber()` function onclick.
2. The input number is retrieved.
3. The `reverse()` function is called recursively to reverse the number.
4. In each recursive call, the last digit of the number is extracted and added to the reversed number string.
5. The reversed number is displayed in a div element on the webpage.
Output:
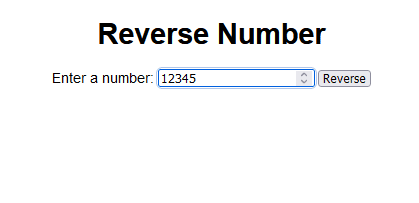
Reversed Number: 54321
In conclusion, exploring the various methods to reverse number in JavaScript, including string reversal, array reduce(), string iteration, and recursion-based approaches, enhances problem-solving skills. Newtum offers a wide range of tutorials and courses in programming languages, fostering continuous learning and growth. Happy coding!