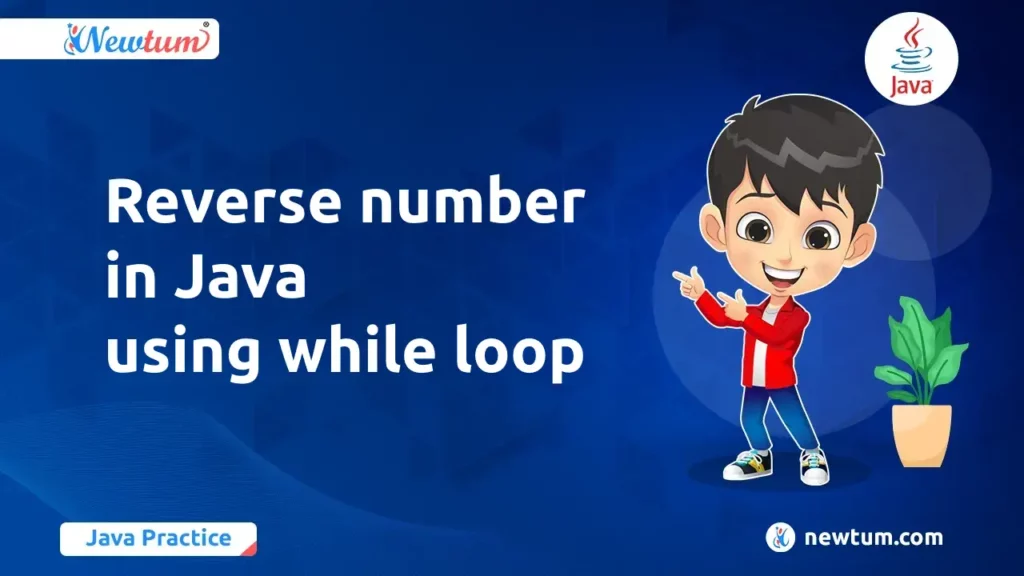
Our aim in this blog is to offer a thorough explanation of how to reverse number in Java using while loop technique In Java, flipping a number around is a regular job. It means putting the digits of a number in the opposite order. This helps in cryptography, math, and data work. Learning to do this with a while loop is important for Java coders. It helps them get better at solving problems and understanding loops.
Basic Algorithm:
To reverse a number using a while loop, follow these steps:
– Initialize variables to store the original and reversed numbers.
– Extract the last digit of the original number and append it to the reversed number.
– Remove the last digit from the original number.
– Repeat the process until the original number becomes zero.
Sample Code- Reverse number in Java using while loop
public class ReverseNumber { public static void main(String[] args) { int num = 1234; // Input number int reversedNum = 0; while (num != 0) { int digit = num % 10; reversedNum = reversedNum * 10 + digit; num /= 10; } System.out.println("Reversed Number: " + reversedNum); } }
Explanation of the Code:
– Initialize variables `num` and `reversedNum` to store the original and reversed numbers, respectively.
– Inside the while loop, extract the last digit of `num` using the modulus operator `%`.
– Append the extracted digit to `reversedNum` by multiplying it by 10 and adding the extracted digit.
– Remove the last digit from `num` by integer division `/`.
– Repeat the process until `num` becomes zero.
Output:
Reversed Number: 4321
Dive into the world of Java programming and master the reverse number in java using for loop in a snap!
Handling Edge Cases- Reverse number in Java using while loop:
- Handling Negative Numbers:
Use `Math.abs()` to obtain the absolute value of negative numbers, ensuring consistent processing regardless of input sign.
- Zero and Large Numbers:
Set the loop termination condition appropriately to handle scenarios involving zero or large numbers.
- Validation and Error Handling:
Implement input validation to prevent invalid inputs, such as non-numeric characters or excessively large numbers.
- Robust Error Messages:
Provide informative error messages to guide users on correct input formats and ranges.
- Testing with Boundary Values:
Conduct thorough testing with boundary values, including zero, negative numbers, and the maximum range, to ensure the program’s robustness and correctness.
Learn How to Reverse a Number in Python Using Recursion Here!
Benefits and Applications of Reversing Numbers in Java
From the following section, you’ll be able to learn about the benefits and applications of reverse number in Java using while loops:
Enhanced Problem-Solving Skills:
Reversing numbers fosters analytical thinking and problem-solving abilities, crucial in various programming scenarios.
Strengthened Understanding of Loop Constructs:
Mastery of reversing numbers improves comprehension of loop constructs like while loops, contributing to more efficient and effective code implementation.
Applications in Cryptography:
Understanding number reversal is vital in cryptography for tasks like encryption and decryption, where manipulating numerical data is fundamental.
Mathematical Calculations:
Reversed numbers play a role in mathematical calculations, such as finding palindromic numbers or determining factors and multiples.
Data Manipulation:
Reversing numbers is essential in data manipulation tasks like sorting algorithms, where rearranging elements in a sequence is necessary for analysis or presentation.
Interview Questions
Check out below given interview questions about reverse number in Java using while loop:
1. How can you reverse number in Java using while loop?
Answer: Iterate through the digits of the number by continuously dividing by 10 and extracting the remainder until the number becomes zero.
2. What’s the significance of using a while loop in reversing a number?
Answer: While loops are effective for iterating through digits until all are processed, ensuring flexibility for varying input lengths.
3. How do you handle negative numbers when reversing them?
Answer: Use Math.abs() to convert negative numbers to positive before reversing.
4. What’s the approach to handle zero or large numbers when reversing?
Answer: Ensure the loop termination condition is properly set to handle zero and prevent infinite looping for large numbers.
5. Can you explain how to reverse a number without using additional storage?
Answer: By using mathematical operations like modulo and division, digits can be extracted and concatenated in reverse order without extra storage.
Within the confines of this blog ‘reverse number in Java using while loop’, we aim to provide a comprehensive understanding of reversing numbers in Java, utilizing the while loop approach. It emphasizing its educational and practical significance. Aspiring programmers can further enhance their skills through the wide array of tutorials and courses available on Newtum. Keep coding, stay curious, and enjoy the journey of continuous learning and growth!