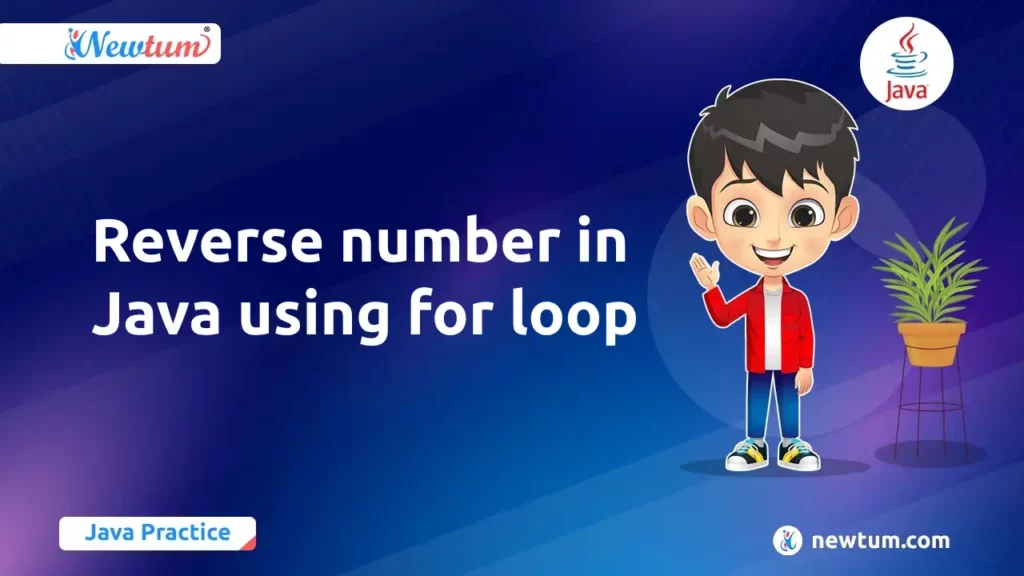
In programming, reversing numbers is a fundamental operation, and understanding how to achieve it efficiently can significantly enhance one’s coding skills. In this blog, we’ll explore the concept of reverse numbers in Java using a for loop, providing a step-by-step guide and highlighting its relevance in various programming tasks.
Sample Code for reverse number in Java using for loop
Check out code snippets below to understand reverse number program in Java using for loop:
#include <stdio.h> //include standard input/output library int main() { //main function int num, reversed = 0; //declare variables for input and reversed number printf("Enter a number: "); //prompt user to enter a number scanf("%d", &num); //read input from user and store in num variable for(int i = num; i != 0; i /= 10) { //for loop to reverse the number int digit = i % 10; //get the last digit of the number reversed = reversed * 10 + digit; //add the digit to the reversed number } printf("Reversed number: %d", reversed); //print the reversed number return 0; //end program }
Explore the fascinating world of reverse number in C using for loop Check out!
Explanation of the Code:
This C program uses a for loop to reverse a given integer entered by the user. Here’s a step-by-step explanation of how it works:
1. The `#include <stdio.h>` directive includes the standard input/output library.
2. The `main()` function serves as the entry point for the program.
3. The statement declares two integer variables num and reversed to store the input number and the reversed number, respectively, and initially sets reversed to 0.
4. The `printf()` function prompts the user to enter a number, and the `scanf()` function reads the input and stores it in the `num` variable.
5. The for loop is used to reverse the number. It initializes `i` with the value of `num` and continues looping as long as `i` is not equal to 0. In each iteration, the code divides ‘i’ by 10 to move to the next digit.
6. Inside the loop, the last digit of `i` is extracted using the modulus operator `%` and stored in the `digit` variable.
7. The reversed number (`reversed`) is calculated by multiplying the existing value of `reversed` by 10 and adding the extracted digit.
8. Finally, the reversed number is printed using the `printf()` function.
9. The `return 0;` statement indicates the successful execution of the program and terminates it.
Output:
Enter a number: 123456789
Reversed number: 987654321
Domains in which reverse number applied
Reversing numbers finds utility across diverse domains, pivotal for various practical applications:
1. Cryptography
2. Data Validation.
3. Numerical Analysis
4. Text Processing
5. Game Development
Learn How to reverse number in C using while loop Here!
Comparison of methods in reverse number:
Reverse number in Java using for loop and other available methods are presented to understand properly:
Methods | Description | Advantages | Disadvantage |
For Loop | Utilizes a loop structure with a predetermined number of iterations, iterating over each digit of the number | Simple and concise implementation | May be less flexible than the while loop method for certain scenarios |
While Loop | Similar to the for loop method, but employs a different loop structure where the condition for termination is explicitly defined within the loop. | Offers flexibility in defining termination conditions making it suitable for scenarios with dynamic requirements. | Requires explicit management of loop control variables potentially leading to more verbose code compared to the for loop method |
Function | It involves encapsulating the logic for reversing a number within a reusable function, providing modularity and facilitating modular design and maintenance. | Advantages Promotes code reusability and abstraction, facilitating modular design and maintenance. | May introduce overhead due to function call overhead, particularly in performance-critical applications. |
In this blog, we explored the reverse number concept in Java using a for loop, discussing its significance in programming. Utilize Newtum’s tutorials and courses to enhance your skills further. Keep coding, stay curious, and continue learning to achieve success in your programming journey. Happy coding with Newtum!
Reverse number in Java using for loop- FAQ
Answer: Reversing a number helps in tasks like cryptography, data validation, and numerical computations.
Answer: The for loop method offers a concise and structured approach compared to other methods, making it easy to implement and understand.
Answer: Yes, the for loop method can handle negative numbers effectively by reversing their absolute values and then adjusting the sign.
Answer: The for loop provides a simple and efficient way to iterate over the digits of a number, making it suitable for reversing operations.
Answer: One limitation is that for loops may have fixed iteration counts, which may not be suitable for all scenarios, especially when dealing with dynamic input sizes.