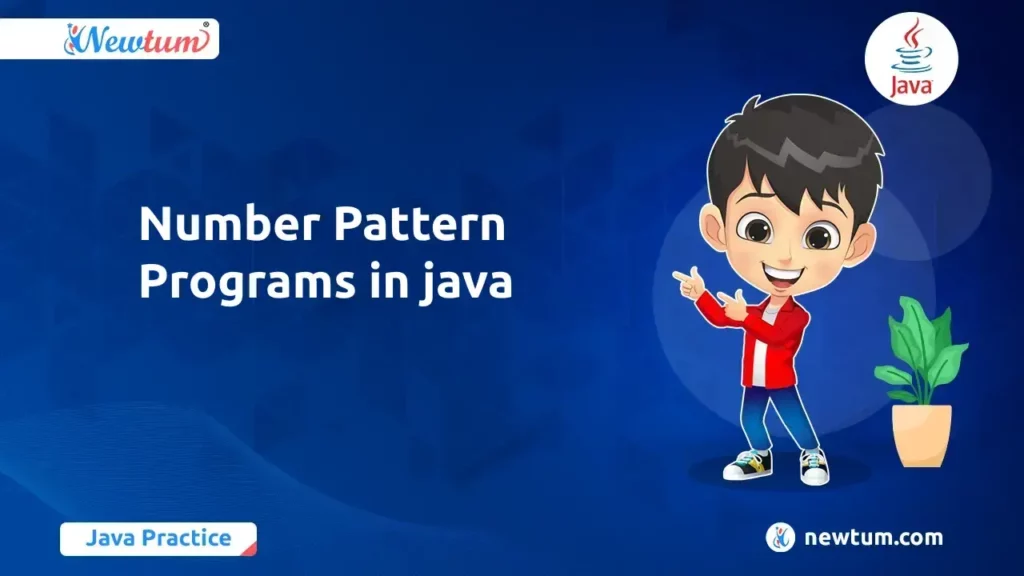
Welcome to our blog on Number Pattern Programs in Java! If you’ve ever marveled at the intricate designs made out of numbers, you’re in for a treat. In this blog, we’ll explore the fascinating world of creating mesmerizing patterns using simple Java code. Let’s dive in and unleash your creativity!
Number Pattern Programs in Java
Number Pattern Programs in Java involve creating various patterns using numbers, such as triangles, squares, or pyramids. These programs use loops and conditions to arrange numbers in specific shapes and sequences, let’s learn more about Number Pattern Program in Java through this code:
// Number Pattern Programs in Java import java.util.Scanner; public class numberPatternEx{ public static void main(String[] args){ int i, j, n; Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); n = sc.nextInt(); for (i = 1; i <= n; i++){ for (j = i; j >= 1; j--){ System.out.print(j+" "); } System.out.println(); } } }
Explanation of the Code:
1. The code starts by importing the `Scanner` class from the `java.util` package to facilitate user input.
2. The `main` method serves as the entry point of the program.
3. A `Scanner` object `sc` is created to read user input. The user is prompted to enter the number of rows for the pattern.
4. The outer loop (`for i`) manages the rows, iterating from 1 to `n`. The inner loop (`for j`) handles the elements in each row, iterating from `i` to 1. Within the inner loop, the value of `j` is printed with a space.
5. After each inner loop iteration, a new line is printed to move to the next row in the pattern.
6. Once all rows are printed, the pattern generation process completes, and the program execution ends.
Output:
Enter the number of rows: 7
1
2 1
3 2 1
4 3 2 1
5 4 3 2 1
6 5 4 3 2 1
7 6 5 4 3 2 1
Basic Number Patterns
Basic number patterns in Java involve using loops to create simple sequences of numbers often arranged in a pyramid or triangle shape.
A. Print Triangle Number Pattern in Java
Check out the code below for a clearer understanding of Triangle Patterns in Java:
//importing necessary packages import java.util.*; //defining class public class Main { public static void main(String[] args) { //creating scanner object to take user input Scanner input = new Scanner(System.in); //prompting user to enter the number of rows for the triangle System.out.println("Enter the number of rows for the triangle: "); //storing user input in a variable int rows = input.nextInt(); //outer loop to iterate through each row for(int i=1; i<=rows; i++){ //inner loop to print the numbers in each row for(int j=1; j<=i; j++){ //printing the numbers in ascending order System.out.print(j + " "); } //printing a new line after each row is printed System.out.println(); } //closing the scanner object input.close(); } }
Explanation of the code:
1. User Input: The program prompts the user to enter the number of rows for the triangle.
2. Pattern Generation**: Using nested loops, the program generates and prints a number triangle. The outer loop controls the number of rows, and the inner loops print the numbers in each row in ascending order.
3. Printing Numbers: Inside the inner loop, numbers are printed in ascending order from 1 to the current row number.
4. Output: The completed number triangle is printed to the console.
5. Resource Management: Finally, the scanner object is closed to release system resources.
Output:
Enter the number of rows for the triangle: 5
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
B. Creating Pyramid Pattern in Java by Number
Take a look at the code provided to grasp Pyramid number pattern in Java more effectively.
public class Main { public static void main(String[] args) { int n = 5; for (int i = 0; i < n; i++) { for (int j = 0; j < n - i; j++) { System.out.print(" "); } for (int k = 0; k <= i; k++) { System.out.print(k + 1); } for (int l = i - 1; l >= 0; l--) { System.out.print(l + 1); } System.out.println(); } } }
Explanation of the code:
1. Outer Loop: The outer loop iterates over each row of the pattern, from 0 to n – 1, where n is the specified number of rows.
2. Leading Spaces: Within each row, an inner loop prints leading spaces to create a pyramid shape, with the number of spaces decreasing with each row.
3. Ascending Numbers: Another inner loop prints ascending numbers from 1 to i + 1, where i represents the current row number.
4. Descending Numbers After printing ascending numbers, a third loop prints descending numbers from i – 1 to 0, forming the bottom half of the pyramid.
5. Output: The completed pattern is printed to the console.
Output:
1
121
12321
1234321
123454321
C. Square number pattern in Java
The following code demonstrates square number patterns in Java, aiding comprehension.
//importing the necessary packages import java.util.*; //defining the class public class Main { public static void main(String[] args) { //creating a Scanner object to take user input Scanner input = new Scanner(System.in); //prompting the user to enter the number of rows for the pattern System.out.print("Enter the number of rows: "); //storing the user input in a variable int rows = input.nextInt(); //using nested for loops to print the pattern for(int i=1; i<=rows; i++){ for(int j=1; j<=rows; j++){ //printing the square number at each position System.out.print(i*j + " "); } //moving to the next line after each row is printed System.out.println(); } } }
Explanation of the code:
1. Importing Packages: The code imports the `java.util` package to access the Scanner class for user input.
2. Main Class: The Main class is defined as the entry point of the program.
3. User Input: A Scanner object is created to read user input for the number of rows in the pattern.
4. Nested Loops: Two nested for loops are used to iterate through each row and column of the pattern grid.
5. Printing Pattern: The product of row number (i) and column number (j) is printed at each position to create a square number pattern.
6. Output: The completed pattern is printed to the console, with each row separated by a new line.
Output:
Enter the number of rows: 3
1 2 3
2 4 6
3 6 9
Advanced Number Patterns in Java
Advanced Number Patterns in Java involve intricate geometric shapes and designs generated using complex algorithms and nested loops.
A. Diamond Patterns
Review the code below to enhance your understanding of Diamond Patterns in Java.
public class Main { public static void main(String[] args) { int n = 5; for (int i = 1; i <= n; i++) { for (int j = i; j < n; j++) { System.out.print(" "); } for (int k = 1; k < (i * 2); k++) { System.out.print(k); } System.out.println(); } for (int i = n - 1; i >= 1; i--) { for (int j = n; j > i; j--) { System.out.print(" "); } for (int k = 1; k < (i * 2); k++) { System.out.print(k); } System.out.println(); } } }
Explanation of the code:
1. Main Class and Method: The code defines a Main class with a main method as the entry point for the program.
2. Outer Loop for Rows: It starts an outer loop to iterate over each row of the pattern.
3. Leading Spaces: Within each row, a loop prints leading spaces based on the row number.
4. Printing Numbers: Another loop prints the numbers in increasing order up to (i * 2) for each row.
5. Output: After each row, a new line is printed.
6. Lower Half of the Diamond: Similar logic is used to print the lower half of the diamond with decreasing row numbers.
Output:
1
123
12345
1234567
123456789
1234567
12345
123
1
B. Pascal’s Triangle
Examining the code below can improve your grasp of Pascal’s Triangle Patterns in Java.
//importing necessary libraries import java.util.Scanner; //defining class public class Main { //main method public static void main(String[] args) { //creating scanner object to take user input Scanner input = new Scanner(System.in); //prompting user to enter the number of rows for Pascal's Triangle System.out.print("Enter the number of rows for Pascal's Triangle: "); //storing user input in a variable int rows = input.nextInt(); //creating a nested for loop to print the triangle for (int i = 0; i < rows; i++) { //printing spaces to create a pyramid shape for (int j = 0; j < rows - i; j++) { System.out.print(" "); } //initializing a variable to store the value of each element in the triangle int num = 1; //printing the elements in each row for (int k = 0; k <= i; k++) { //printing the value of each element System.out.print(num + " "); //updating the value of num for the next element num = num * (i - k) / (k + 1); } //printing a new line after each row System.out.println(); } } }
Explanation of the code:
1. User Input: The program prompts the user to enter the number of rows for Pascal’s Triangle and stores the input in an integer variable.
2. Pattern Generation: Using nested loops, the program generates and prints Pascal’s Triangle. The outer loop controls the number of rows, and the inner loops print the spaces and elements in each row to create the triangle shape.
3. Space Printing: Inside the outer loop, spaces are printed to create a pyramid shape for the triangle.
4. Element Calculation: Within the inner loop, each element’s value is calculated using the binomial coefficient formula.
5. The completed Pascal’s Triangle is printed to the console.
Output:
Enter the number of rows for Pascal's Triangle: 4
1
1 1
1 2 1
1 3 3 1
C. Floyd’s Triangle Pattern
Below mentioned code of Floyd’s triangle Pattern will help know this concept thoroughly:
//importing necessary packages import java.util.Scanner; //defining class public class Main { public static void main(String[] args) { //creating scanner object to take user input Scanner input = new Scanner(System.in); //prompting user to enter the desired pattern name System.out.println("Enter the pattern name: "); //storing user input in a string variable String patternName = input.nextLine(); //printing the pattern name entered by the user System.out.println("Pattern Name: " + patternName); //defining variables for number of rows and current number int rows, currentNum = 1; //prompting user to enter the number of rows for the pattern System.out.println("Enter the number of rows: "); //storing user input in rows variable rows = input.nextInt(); //loop to print the pattern for(int i = 1; i <= rows; i++){ //loop to print the numbers in each row for(int j = 1; j <= i; j++){ //printing the current number and incrementing it System.out.print(currentNum + " "); currentNum++; } //printing a new line after each row System.out.println(); } } }
Explanation of the code:
1. The program starts by importing the necessary packages and defining the main class. It prompts the user to enter the desired pattern name and stores it in a string variable.
2. The entered pattern name is printed back to the user for confirmation.
3. The program prompts the user to enter the number of rows for the pattern and stores the input in an integer variable.
4. Using nested loops, the program generates and prints the pattern based on the number of rows entered by the user. The outer loop controls the number of rows, while the inner loop prints the numbers in each row.
5. The pattern is printed to the console, with each row separated by a new line.
Output:
Enter the pattern name: Number
Pattern Name: Number
Enter the number of rows: 7
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
16 17 18 19 20 21
22 23 24 25 26 27 28
In this blog, we’ve explored the educational and practical significance of Number Pattern Programs in Java, showcasing their role in strengthening programming skills. We encourage readers to experiment with the provided code on the Newtum Compiler, fostering hands-on learning. Explore Newtum tutorials and courses for further programming insights and continue your coding journey with enthusiasm and dedication. Happy coding!