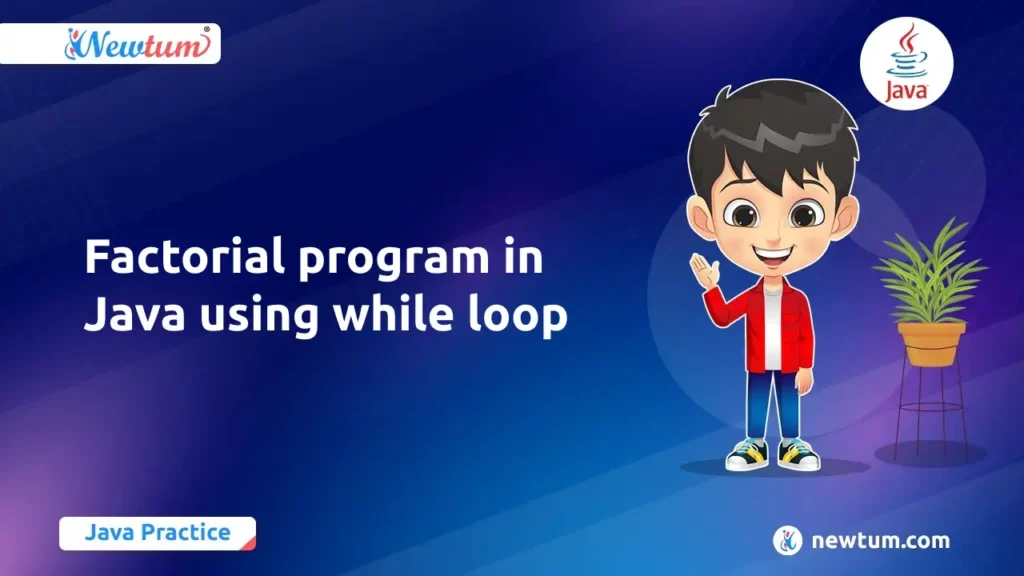
Factorials are math operations that involve multiplying a series of numbers one after another. They’re super helpful in math and coding for things like figuring out combinations or chances. In Java, a while loop is like a tool that repeats a task as long as a condition stays true. It’s great for doing the same thing over and over again until we’re done. With Java’s while loop, we can easily calculate factorials by doing the multiplication step by step. In this blog, we will see how it works!
Java Program to find factorial of a number using while loop
If we want to find out what the factorial of 4 is, we can use the following code to get the answer:
// Factorial program in java using while loop import java.util.Scanner; public class factorialEx { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number: "); int number = sc.nextInt(); long fact = 1; int i = 1; while(i<=number) { fact = fact * i; i++; } System.out.println("Factorial of "+number+" is: "+fact); } }
Explanation of the code-
Here’s a step-by-step explanation of the Java program:
1. Import Scanner Class: Import the `Scanner` class from the `java.util` package to allow user input.
2. Define the Main Class: Define the main class `factorialEx`.
3. Define the Main Method: Define the main method `main(String[] args)`.
4. Create a Scanner Object: Create a `Scanner` object named `sc` to read user input from the console.
5. Prompt for Input: Display a message prompting the user to enter a number.
6. Read User Input: Use the `nextInt()` method of the `Scanner` class to read an integer input from the user and store it in the `number` variable.
7. Initialize Variables: Initialize variables `fact` and `i`. Set `fact` to 1 and `i` to 1.
8. Calculate Factorial: Use a `while` loop to iterate from 1 to `number`. Multiply `fact` by `i` in each iteration and increment `i` by 1.
9. Display Result: Once the loop terminates, print the factorial of the input number using `System.out.println()`.
This program calculates the factorial of a number entered by the user using a `while` loop. It iterates through each number from 1 to the input number, multiplying them to compute the factorial. Finally, it prints the result to the console.
Output:
Enter the number: 6
Factorial of 6 is: 720
Importance of Factorials
Factorials hold significant importance in both mathematics and programming due to their versatile applications. Here’s why they are essential:
1. Combinatorics: Factorials are crucial in combinatorial mathematics, where they represent the number of permutations and combinations of objects. They help solve problems related to arrangements and selections.
2. Probability: Factorials are used in probability theory to calculate the number of outcomes in an experiment. They play a vital role in determining the probability of events occurring.
3. Mathematical Series: Factorials are integral to various mathematical series, such as Taylor series and binomial expansions. They facilitate the representation and manipulation of these series.
4. Algorithm Design: In programming, factorials are utilized in algorithm design, particularly in recursive algorithms and dynamic programming. They enable efficient solutions to problems involving repeated calculations or permutations.
5. Recursive Functions: Factorials serve as classic examples in teaching recursive function implementation. Recursive factorial functions demonstrate the concept of function calling itself, aiding in understanding recursion.
6. Efficiency Optimization: In some programming tasks, knowing how to calculate factorials efficiently can optimize performance. For example, in problems requiring factorial computation, understanding efficient factorial algorithms can lead to faster execution times.
Advantages of Using While Loop
Using a while loop for factorial calculation offers several advantages, making it a suitable choice for such tasks:
1. Dynamic Control: While loops provide dynamic control over iterations based on a condition. In factorial calculation, the loop continues until reaching the desired number, accommodating varying input sizes.
2. Variable Initialization: While loops allow flexible initialization of loop variables, enabling precise control over the starting point of calculations. This flexibility is beneficial for factorial computation, where the loop starts from 1.
3. Incremental Approach: While loops follow an incremental approach, incrementing the loop variable with each iteration. This aligns with factorial calculation, where consecutive numbers are multiplied together step by step.
4. Conditional Termination: While loops terminate based on a specified condition, such as reaching the desired number. In factorial calculation, the loop stops when reaching the input number, ensuring accurate computation without unnecessary iterations.
5. Clear Logic: While loops offer clear and concise logic for iterative tasks, enhancing code readability. This clarity is advantageous for factorial calculation, as it simplifies understanding the iterative process involved in multiplying consecutive numbers.
This was brief about different methods of calculating the Factorial program, Now excel each method such as factorial program in c using for loop, factorial program in c using function and factorial program in c using while loop.
Tips for Better Implementation of Code
To ensure efficient and readable code when implementing factorial calculation using a while loop, consider the following tips:
1. Use Descriptive Variable Names: Choose meaningful variable names like `number` instead of generic names like `n`.
2. Initialize Variables Properly: Initialize loop variables before the loop to ensure predictable behavior and prevent runtime errors.
3. Handle Edge Cases: Include error handling for edge cases like negative numbers or zero.
4. Optimize Loop Conditions: Optimize loop conditions to minimize iterations, e.g., stop loop if factorial exceeds data type limit.
5. Provide Clear Comments: Add comments for clarity, especially in complex or edge cases, aiding understanding and future edits.
6. Test with Various Inputs: Test the code with different input values, including boundary cases and extreme values, to ensure correctness and robustness.
7. Follow Code Style Guidelines: Adhere to coding conventions and style guidelines to maintain consistency and improve code readability.
In conclusion, we explored factorial computation in Java using a while loop, emphasizing its significance in mathematics and programming. We hope that our blog on ‘Factorial Program in Java using while loop’ helped you in understanding the Factorial Number Concept in Java. For more concepts and courses check our Newtum website and broaden your programming expertise.
Factorial program in Java using while loop- FAQ
Answer- The Java program iterates through numbers from 1 to the input number using a while loop, multiplying them to compute the factorial.
Answer- The while loop offers flexibility for scenarios where the number of iterations is not predetermined, making it suitable for factorial calculation.
Answer- Input validation ensures that the user-provided input is valid and within an acceptable range before starting the factorial calculation using the while loop.
Answer- Yes, the while loop efficiently computes factorials for large input values without causing stack overflow issues or performance degradation.
Answer- Understanding the while loop’s structure helps in comprehending how each iteration contributes to the factorial calculation, facilitating better code comprehension and debugging.