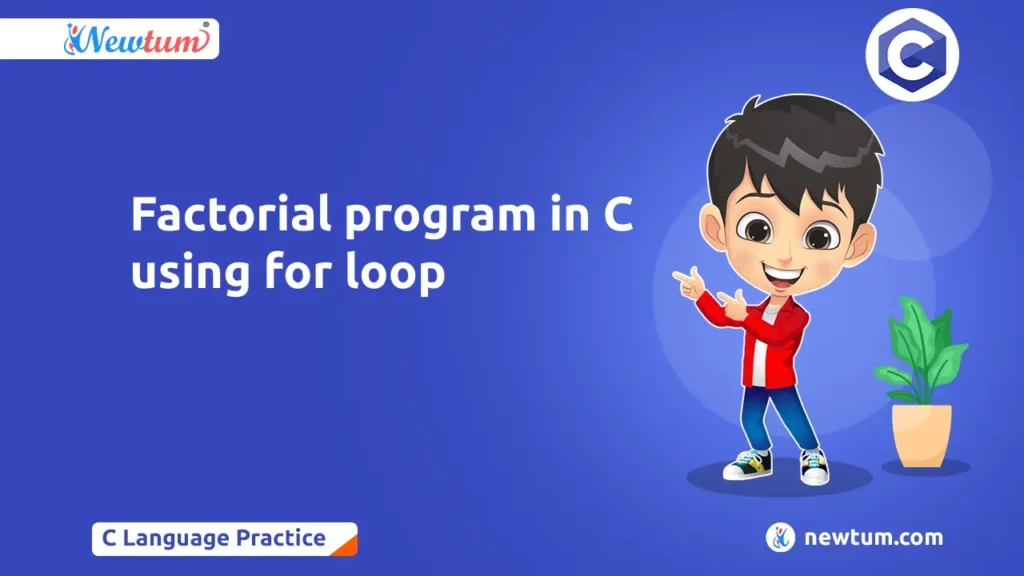
While C programming offers various ways to calculate factorials, like functions and while loops, this blog will specifically explore using for loops. Excelling this method will lay a strong foundation for understanding factorials in C. For loops provide a clear, step-by-step approach, making it easier to grasp the concept and build more complex programs in the future. Let’s dive in and discover how to calculate factorials using for loops in C!
Factorial Program in C using for loop
// Factorial program in C using for loop #include<stdio.h> int main(){ int i,f=1,num; printf("Enter a number: "); scanf("%d",&num); for(i=1;i<=num;i++) f=f*i; printf("Factorial of %d is: %d",num,f); return 0; }
Learn the factorial number in php here!
Explanation of the code:
1. Include Header File: The `#include<stdio.h>` directive adds the standard input/output library, enabling functions like `printf()` and `scanf()`.
2. Main Function Definition: The `int main()` function serves as the program’s entry point.
3. Variable Declaration: Three variables are declared: `i`, `f`, and `num`. `i` is the loop control variable, `f` stores the factorial result initialized to 1, and `num` stores the user-input number.
4. User Input: `printf()` prompts the user for input, and `scanf()` reads the input and stores it in `num`.
5. For Loop: The factorial is calculated using a `for` loop. Initialization: `i` starts at 1. Condition: Loop continues while `i` is less than or equal to `num`. Iteration: `i` increments by 1. The factorial `f` is calculated by multiplying with `i`.
6. Output: `printf()` displays the factorial of the entered number (`num`) along with the result (`f`). The program ends by returning 0.
Output:
Enter a number: 10
Factorial of 10 is: 3628800
Learn the same concept in different programming languages such as the factorial of a number in C#
Importance of Factorial and Why in C Programming?
Factorials (written as n!), multiply all positive numbers less than or equal to n. They’re crucial in mathematics for counting arrangements (permutations) and selections (combinations) – imagine counting unique ways to order pizzas or pick a lottery ticket! C programming, a widely used and versatile language, lets you write programs that solve these problems efficiently. Understanding factorials in C enables you to unlock a powerful tool for various mathematical tasks.
Factorial Program in C using for Loop.
Understanding Factorials in C
- How to calculate factorial manually (Example: 4! = 4 3 2 * 1)
Calculating factorials manually involves multiplying all positive integers up to a given number. For example, to find 4 factorial (4!), we multiply 4 × 3 × 2 × 1, which equals 24.
- Challenges of manual calculation for large numbers
However, manual factorial calculation becomes challenging for large numbers due to the exponential increase in the number of multiplications required. For instance, calculating the factorial of 100 (100!) would involve multiplying 100 numbers, resulting in an enormous computation task prone to errors and inefficiencies. This highlights the necessity for efficient computational methods, such as those implemented in programming languages like C, to handle factorial calculations effectively, especially for large numbers.
What is a Loop?
The for loop is a fundamental building block in C programming, allowing you to execute a block of code repeatedly until a certain condition is met. Below we will understand a breakdown of its structure and functionality:
Basic Structure:
for (initialization; condition; increment/decrement) { // Code to be executed repeatedly }
- Initialization: This statement sets up a loop counter variable at the beginning.
- Condition: This expression determines whether the loop continues iterating. As long as the condition evaluates to true, the loop keeps running.
- Increment/Decrement: This statement updates the loop counter variable after each iteration. It’s commonly used to increment (increase) the counter, but it can also be decremented (decrease) for counting down.
How It Works:
- The initialization statement is executed only once, at the start of the loop.
- The condition is checked. If it’s true, the code block within the curly braces is executed.
- After the code block finishes, the increment/decrement statement updates the loop counter.
- The condition is checked again. This cycle continues as long as the condition remains true.
- Once the condition becomes false, the loop terminates.
Benefits of the For Loop Approach
While there are other ways to calculate factorials in C, using a for loop offers distinct advantages:
- Conciseness and Readability: Compared to manual calculations, the for loop condenses the process into a structured and easy-to-understand block of code. It eliminates repetitive multiplication steps, making the logic clear and efficient.
- Flexibility for Different Numbers: The beauty of the for loop lies in its adaptability. By simply changing the loop condition, you can calculate factorials for any non-negative integer. This makes the code reusable and versatile for various factorial calculations.
In conclusion, this blog equipped you with the knowledge to calculate factorials using for loops in C. You’ve grasped the concept of factorials and how for loops provide a structured approach for repetitive tasks. Feel free to experiment with the code! Change the input number and observe the results. Factorials have applications beyond basic math, extending to areas like probability and computer science. Ready to delve deeper? Explore more programming concepts and languages with courses and blogs offered by Newtum!
Factorial Program in C Using for loop- FAQ
No, this program is designed to calculate factorials only for non-negative integers. It includes error handling to notify users if they enter negative numbers.
The for loop initializes a loop counter variable (`i`), continues iterating as long as the condition (`i <= num`) is true, and updates the loop counter (`i++`) after each iteration.
Yes, this program efficiently calculates factorials for reasonably large numbers. However, extremely large input numbers may exceed the data type’s capacity, leading to inaccurate results.
Understanding factorials is crucial in C programming as it provides a foundation for solving various mathematical and programming challenges efficiently, such as permutations, combinations, and recursive algorithms.
To handle larger numbers, you can optimize the loop condition or use data types with higher precision, like `long long` or `BigInteger` in Java.