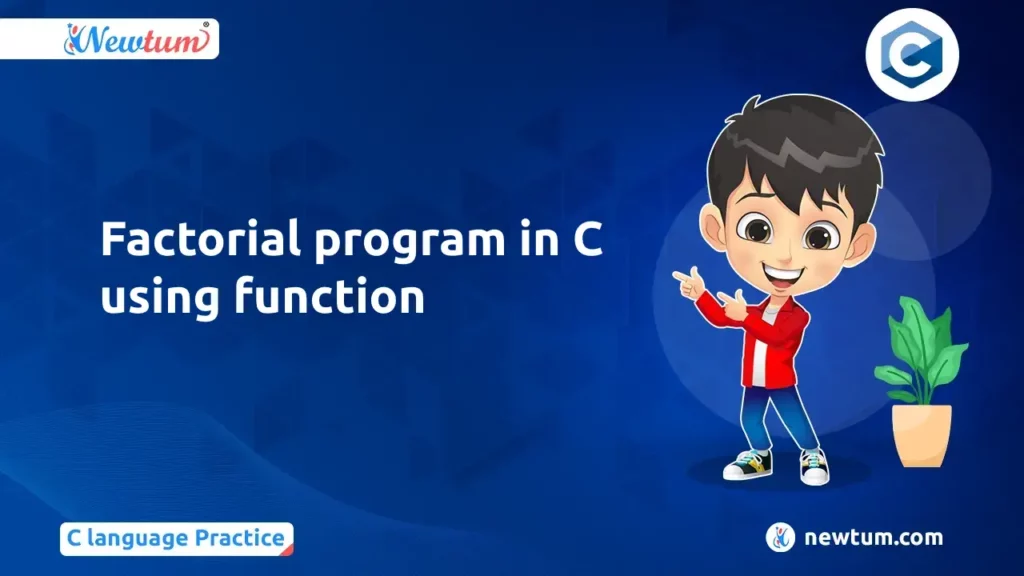
While there are multiple ways to calculate factorials in C programming, such as using for loops and while loops, this blog will focus exclusively on using functions. Functions offer a straightforward, step-by-step process, facilitating an easier understanding of the concept and enabling the creation of more intricate programs down the line. Let’s delve into discovering how to compute factorials using functions in C!
Introduction of Function in C Language
In C programming, functions are like tools in a toolbox (A), each with a specific job. This keeps the program organized and easier to find when needed (B). There is a special function called main(), like a hammer, but you can add custom functions like a fancy screwdriver (C). These functions are like special helpers that can be used repeatedly in the program. By using functions, your code is clean and easy to understand, similar to a well-organized toolbox.
Factorial Program in C using function – Code
// Factorial program in C using function #include<stdio.h> int findFactorial(int); int main(){ int i,factorial,num; printf("Enter a number: "); scanf("%d",&num); factorial = findFactorial(num); printf("Factorial of %d is: %d",num,factorial); return 0; } int findFactorial(int num){ int i,f=1; for(i=1;i<=num;i++) f=f*i; return f; }
Learn the factorial number in php here!
Explanation of the code:
The provided C program calculates the factorial of a given number using a function. Here’s a breakdown:
1. Function Declaration: The code begins with the declaration of a function `findFactorial(int)` which takes an integer argument and returns an integer.
2. Main Function: Inside the `main()` function, variables `i`, `factorial`, and `num` are declared. The user is prompted to enter a number, which is stored in `num`.
3. Factorial Calculation: The `findFactorial()` function calculates the factorial of the input number using a for loop, multiplying each number from 1 to `num`.
4. Result Display: The calculated factorial is stored in the variable `factorial`, which is then displayed along with the input number using `printf()` statement.
5. Return: The function returns the calculated factorial value, which is then assigned to `factorial` in the `main()` function.
6. Completion: Finally, the program returns 0 to indicate successful execution.
Output:
Enter a number: 4
Factorial of 4 is: 24
Learn the same concept in different programming languages such as the factorial of a number in C#
How to write the factorial function?
A. Declare and define a function named `findFactorial` to calculate factorial.
B. The function accepts an integer parameter `num` and returns an integer representing the factorial.
C. In the main program, call `findFactorial(num)` to compute the factorial of a user-input number `num`. Store the result in a variable like `factorial`.
This modular approach enhances code organization and readability, separating the factorial logic into a reusable function for better maintainability.
Benefits of Using Functions
A. Functions offer several advantages for factorial calculation, such as encapsulating the factorial logic into a modular and reusable unit. This enhances code organization, reduces redundancy, and promotes code reuse, leading to more efficient and scalable programs.
B. By using functions, code readability, and maintainability are significantly improved. Functions abstract away complex logic, making the code easier to understand and modify. This modular approach enables developers to focus on specific tasks within the program, resulting in cleaner and more manageable codebases.
C. Embracing functions for factorial calculation encourages developers to apply the same approach to solve other programming problems. This fosters good coding practices, promotes code reuse, and enhances problem-solving skills, ultimately leading to the development of more robust and scalable software solutions.
Tips and Tricks for Better Implementation
1. Dealing with Errors: Explain how the program manages unusual situations, such as negative numbers or non-whole number inputs. Discuss the error messages or validation methods used to handle these cases smoothly.
2. Understanding Efficiency: Describe the time and space complexity of the factorial function, analyzing its efficiency and any performance considerations when dealing with large inputs.
3. Alternative Approach: Introduce a recursive version of the factorial function and compare it to the iterative one. Highlight the differences and trade-offs between these two methods.
4. Real-World Examples: Provide some common scenarios where factorial calculations are used, such as in math, probability, and combination problems.
5. Optimization: Discuss ways to improve the performance of the factorial function, like memoization or using mathematical properties.
6. Extension Exercises: Suggest additional exercises for readers to explore factorial-related algorithms or coding challenges.
7. Using Functions in Other Languages: The concept of functions and calculating factorials extends to other programming languages as well. You can find examples of implementing this in languages like Python or Java.
8. Resources for Learning More: There are many great online tutorials, books, and coding platforms like Newtum where you can practice and deepen your understanding of functions and calculating factorials.
In conclusion, we’ve explored the ‘factorial program in C using functions’, an up-scaling understanding of modular programming. Functions play a crucial role in organizing code and fostering scalability. As you continue your journey, delve deeper into C programming and explore advanced concepts with Newtum. Happy coding!
Factorial Program in C Using Function- FAQ
Answer –This program does not handle non-integer inputs; it expects the user to enter whole numbers only.
Answer – The `findFactorial()` function uses a for loop to calculate the factorial of the input number.
Answer – Functions help organize code, promote code reuse, and improve maintainability by encapsulating specific tasks.
Answer – Yes, you can optimize the code or use larger data types to handle larger factorial calculations.
Answer – Functions enhance code organization, reduce redundancy, and promote code reuse, leading to more efficient and scalable programs.