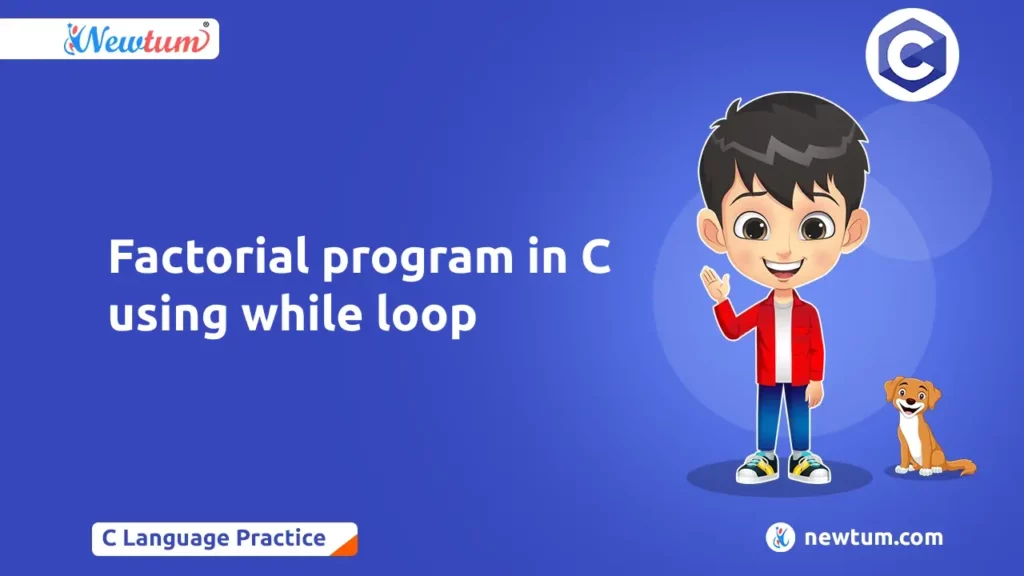
This blog focuses on creating a C program that calculates factorials using a while loop. Before we get into the technical specifics, we’ll start with a basic introduction and explain the code and its output.
Code- Factorial Program in C using while loop
// Factorial program in C using while loop #include<stdio.h> int main(){ int i=1,f=1,num; printf("Enter a number: "); scanf("%d",&num); while(i<=num){ f=f*i; i++; } printf("Factorial of %d is: %d",num,f); return 0; }
Explanation of the code-
This C program computes the factorial of a given number using a while loop. Here’s how it works:
1. Variable Declarations: Three variables are declared: `i` to serve as a loop counter initialized to 1, `f` to store the factorial initialized to 1, and `num` to store the user input.
2. While Loop Structure: The while loop executes as long as `i` is less than or equal to `num`. Inside the loop:
– `f` is multiplied by `i`, updating the factorial value.
– `i` is incremented by 1.
3. Factorial Calculation: The loop continues until `i` exceeds `num`, ensuring that all numbers from 1 to `num` are multiplied together to compute the factorial.
4. Display Result: After the loop, the calculated factorial is printed using `printf()`.
Output:
Enter a number: 5 Factorial of 5 is: 120
Learn the same concept in different programming languages such as the factorial of a number in C#
Understanding the While Loop:
While loops in C repeatedly execute a block of code as long as a specified condition remains true. In this program:
- The loop initializes `i` to 1.
- It continues as long as `i` is less than or equal to `num`.
- Within each iteration, `f` is updated by multiplying it with `i`, and `i` is incremented.
- While loops are ideal for situations where the number of iterations is not predetermined, as in this factorial calculation scenario.
Benefits of Using While Loops
Using while loops in programming offers several benefits which are mentioned below:
1. Dynamic Iteration: While loops are ideal for scenarios where the number of iterations is not predetermined, allowing flexibility for repetitive tasks.
2. Conditional Control: They provide the ability to control loop execution based on conditions, enabling precise control over loop behavior.
3. Conditional Logic: While loops can incorporate conditional statements (if-else) within their structure, facilitating complex decision-making and logic execution within the loop. This enhances the versatility of while loops in handling diverse programming tasks efficiently.
Real-life Applications of Factorial Programs
Factorial programs using while loops have practical applications in various real-life scenarios:
1. Computational Mathematics: Factorials are fundamental in combinatorial mathematics, probability theory, and mathematical analysis, where permutations, combinations, and probability calculations are involved.
2. Algorithm Design: Factorial computation forms the basis of algorithms in fields such as cryptography, optimization, and artificial intelligence. For instance, factorial calculations are employed in generating permutations and combinations, essential in algorithm design.
3. Statistical Analysis: Factorials are utilized in statistical analysis, particularly in calculating permutations and combinations in experiments, surveys, and data analysis.
4. Engineering Calculations: In engineering disciplines, factorial calculations are applied in areas like reliability analysis, system design, and optimization tasks.
5. Financial Modeling: Factorial computations play a role in financial modeling, especially in scenarios involving compound interest, investment analysis, and risk assessment.
Overall, factorial programs using while loops find extensive usage across various domains, showcasing their importance and relevance in practical applications beyond theoretical programming concepts.
Optimization Techniques for Factorial Calculation:
Factorial computation can be optimized for better performance and efficiency. Some optimization techniques are mentioned below:
1. Memoization: Store previously computed factorial values in a cache to avoid redundant calculations. This technique improves efficiency, especially for recursive factorial functions.
2. Iterative Approach: While loops can sometimes be more efficient than recursive functions for factorial calculation, as they eliminate the overhead of function calls and stack operations.
3. Advanced Mathematical Properties: Exploit mathematical properties of factorials, such as the factorial recurrence relation or Stirling’s approximation, to optimize calculations for large inputs.
4. Data Type Optimization: Use appropriate data types, such as long int or unsigned long long, to handle larger factorial values without overflow or loss of precision.
In conclusion, we’ve explored ‘factorial calculation using while loops in C’, understanding its structure and functionality. Experiment with the code by inputting different numbers to deepen your understanding. Factorials extend beyond basic math, finding applications in various fields. Explore Newtum for various programming courses and blogs, allowing you to deepen your understanding of programming languages and concepts.
Factorial Program in C Using while loop- FAQ
Answer- No, this program is designed to calculate factorials only for non-negative integers.
Answer- The program expects whole numbers (integers) as input. If you enter a non-integer value, the program may behave unpredictably.
Answer- The while loop continues to execute as long as a specified condition (i <= num) remains true. It iterates through each number from 1 to num, multiplying them to calculate the factorial.
Answer- Yes, the program’s capability depends on the data type used. Larger numbers may exceed the data type’s capacity, leading to incorrect results or overflow issues.
Answer- Yes, optimization techniques such as memoization, using mathematical properties, or employing more efficient algorithms can enhance the program’s performance for larger inputs.