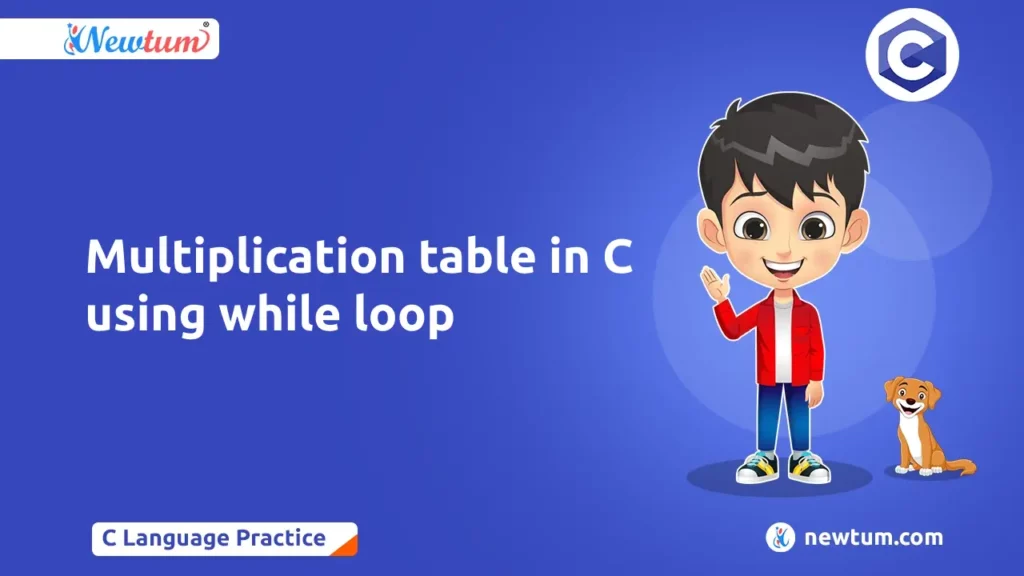
This blog introduces multiplication table and C programming, guiding beginners through while loops, and enhancing their understanding of programming logic and mathematical operations.
Understanding multiplication tables is fundamental to developing math and problem-solving skills. It forms the basis of various mathematical concepts and helps with quick mental calculations. Moreover, understanding multiplication tables is required in fields like science, engineering, and computer programming.
Definition
Multiplication tables are structured grids displaying the products of two numbers, typically ranging from 1 to 10 or 12. They hold immense significance as fundamental tools in arithmetic, aiding in rapid calculations and problem-solving.
How do multiplication tables aid in mathematical understanding?
By memorizing these tables, individuals develop strong numerical fluency, enhancing their overall mathematical comprehension. Mastery of multiplication tables facilitates easier understanding of mathematical operations, patterns, and relationships.
Commonly used multiplication tables (e.g., 12×12)
Commonly used tables include the 12×12 grid, covering products up to 144, and the 10×10 grid, often used in primary education. Understanding these tables lays a solid foundation for advanced mathematical concepts and real-world applications.
Multiplication table program in C using while loop
// Multiplication table in c using while loop #include<stdio.h> int main() { int i=1,m,a; int num=10; printf("Enter the table's number:"); scanf("%d",&a); while(i<=num) { printf("\n%d*%d=%d",i,a,i*a); i++; } return 0; }
Explanation of the code:
This C program generates a multiplication table for a given number entered by the user.
- Initialization: Variables `i`, `a`, and `num` are initialized. `i` serves as the loop counter, `a` stores the user input for the table number, and `num` determines the size of the table.
- User Input: The program prompts the user to enter the number for which they want to generate the multiplication table.
- While Loop: The program utilizes a `while` loop to iterate from 1 to `num`. Within the loop, each multiplication operation (`i * a`) is printed in the format `i*a=i*a`.
Output:
Enter the table's number:12
1*12=12
2*12=24
3*12=36
4*12=48
5*12=60
6*12=72
7*12=84
8*12=96
9*12=108
10*12=120
Learn more about the Multiplication Table in Python Using Recursion and Multiplication Table in Python Using Function now!
Real-world Applications and Extensions
A. Incorporating the multiplication table into larger C programs:
Multiplication tables can be integrated into various larger C programs, such as:
- Financial applications: Calculating compound interest or amortization schedules.
- Scientific simulations: Implementing algorithms that require repetitive mathematical computations.
- Data analysis: Generating datasets with simulated data for testing algorithms or models.
B. Using multiplication tables for educational purposes:
– Interactive learning tools: Developing educational software to help students practice multiplication tables interactively.
– Classroom activities: Designing games or exercises that reinforce multiplication skills.
– Curriculum integration: Incorporating multiplication tables into lesson plans across various subjects like mathematics, science, and computer science.
C. Exploring other applications of multiplication tables in programming:
– Pattern recognition: Utilizing multiplication tables to identify patterns and relationships in data.
– Algorithm optimization: Leveraging precomputed multiplication tables to optimize algorithms and reduce computational overhead.
– Code optimization: Replacing repetitive multiplication operations with table lookups for improved performance in performance-critical applications.
Finally, by utilizing while loops to generate multiplication tables in C, we have strengthened our understanding of basic programming ideas and mathematical principles. Learning multiplication tables enhances mathematical understanding, problem-solving skills, and programming abilities across various fields through practice and investigation.
In conclusion, we’ve explored how to create multiplication tables in C using while loops, reinforcing fundamental programming concepts and mathematical skills. Further practice and exploration can deepen understanding. Mastering multiplication tables not only enhances programming proficiency but also fosters strong mathematical foundations, crucial for problem-solving in various disciplines. Unlock your potential with Newtum – Where learning meets innovation. Explore our courses today to embark on a journey of continuous growth and mastery