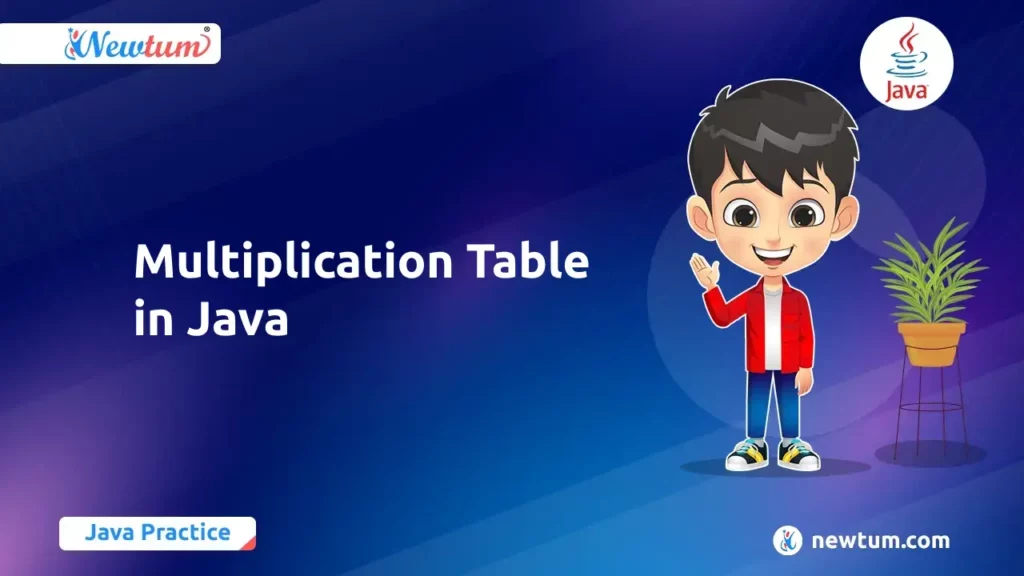
The multiplication table in Java is a fundamental concept for beginners, aiding in understanding arithmetic operations and loop structures. It involves generating a grid of numbers representing the products of two factors. Through simple code, it fosters comprehension of iteration and mathematical principles, serving as a cornerstone in programming education.
Multiplication table in Java- Code
// Multiplication table in Java import java.util.Scanner; public class Multiplication_Table { public static void main(String[] args) { Scanner s = new Scanner(System.in); System.out.print("Enter number:"); int n=s.nextInt(); for(int i=1; i <= 10; i++) { System.out.println(n+" * "+i+" = "+n*i); } } }
Learn more about the Multiplication Table in Python Using Recursion and Multiplication Table in Python Using Function now!
Explanation of the code-
Here is the explanation of the above given Java code for generating a multiplication table:
1. Import Necessary Packages: The code starts by importing the `Scanner` class from the `java.util` package to facilitate user input.
2. Define the Class: The class `Multiplication_Table` is defined to encapsulate the functionality of the program.
3. Main Method: The `main` method is declared as the entry point of the program.
4. User Input: A `Scanner` object `s` is created to read user input. The user is prompted to enter a number for which the multiplication table needs to be generated.
5. Loop for Multiplication Table: A `for` loop is used to iterate from 1 to 10. Within each iteration:
   – The product of the input number (`n`) and the loop counter (`i`) is calculated (`n * i`).
   – The result is printed in the format `n * i = result`.
6. End of Program: Once all multiples are printed, the program execution ends.
This code efficiently generates a multiplication table for the input number provided by the user, displaying the results up to the 10th multiple.
Output:
Enter number:6
6 * 1 = 6
6 * 2 = 12
6 * 3 = 18
6 * 4 = 24
6 * 5 = 30
6 * 6 = 36
6 * 7 = 42
6 * 8 = 48
6 * 9 = 54
6 * 10 = 60
Customization Options
You can customize the program to generate multiplication tables of different sizes or start from different numbers by adjusting the input parameters and loop conditions.
1. Customizing Table Size: To generate a multiplication table of a different size, users can modify the loop condition in the `for` loop. For example, to generate a table up to the 12th multiple, change `i <= 10` to `i <= 12`.
2. Customizing Starting Number: Users can also customize the starting number of the multiplication table by changing the initial value of the loop counter (`i`). For instance, to start the table from the 5th multiple, initialize `i` to 5 instead of 1.
3. User Input: Alternatively, users can prompt for input to specify the size of the table and the starting number dynamically. To achieve this, read input from the user for these parameters and utilize them in the loop conditions and calculations.
4. Modularization: For better code organization and reusability, consider encapsulating the multiplication table generation logic into a separate method. This
Learn How to Generate Multiplication table in C using for loop Now!
Practical Applications of Multiplication table in Java
Multiplication tables are not just mathematical exercises but also have practical applications in various real-world scenarios:
1. Education: Multiplication tables are fundamental in mathematics education, helping students learn and understand multiplication concepts and memorize multiplication facts.
2. Finance: Financial analysts and professionals often use multiplication tables for calculations involving interest rates, investments, and financial projections.
3. Engineering: Engineers utilize multiplication tables for computations related to scaling, dimensions, and conversions in various engineering disciplines such as civil engineering, mechanical engineering, and electrical engineering.
4. Computer Science: In computer science and programming, we utilize multiplication tables in algorithm design, optimization techniques, and data structures.
5. Gaming: Game developers may use multiplication tables for generating game assets, implementing game mechanics, or optimizing game algorithms.
6. Statistics: Statisticians and data analysts use multiplication tables for computations related to probability distributions, statistical models, and data analysis.
7. Business: Multiplication tables are essential in business operations for calculations involving sales projections, revenue forecasts, and profit margins.
8. Architecture: Architects may employ multiplication tables for scaling drawings, calculating dimensions, and designing structures in architectural projects.
9. Science: Scientists utilize multiplication tables for calculations in various scientific fields such as physics, chemistry, biology, and environmental science.
10. Healthcare: Healthcare professionals may use multiplication tables for dosage calculations, medical measurements, and medical research.
In conclusion, we’ve explored the significance of multiplication tables in our blog on ‘Multiplication table in Java’, understanding its practical applications and customization options. We encourage readers to use this knowledge and explore further programming concepts on Newtum. Keep learning, practicing, and expanding your programming skills to unlock new opportunities.
Multiplication table in Java- FAQ
Answer – Yes, you can adjust the loop condition to generate tables of different sizes.
Answer – Absolutely, simply modify the initial value of the loop counter.
Answer – No, they have practical applications in finance, engineering, gaming, and more.
Answer – You can create a separate method to generate the table.
Answer – No, you can predefine the parameters for table generation within the code.