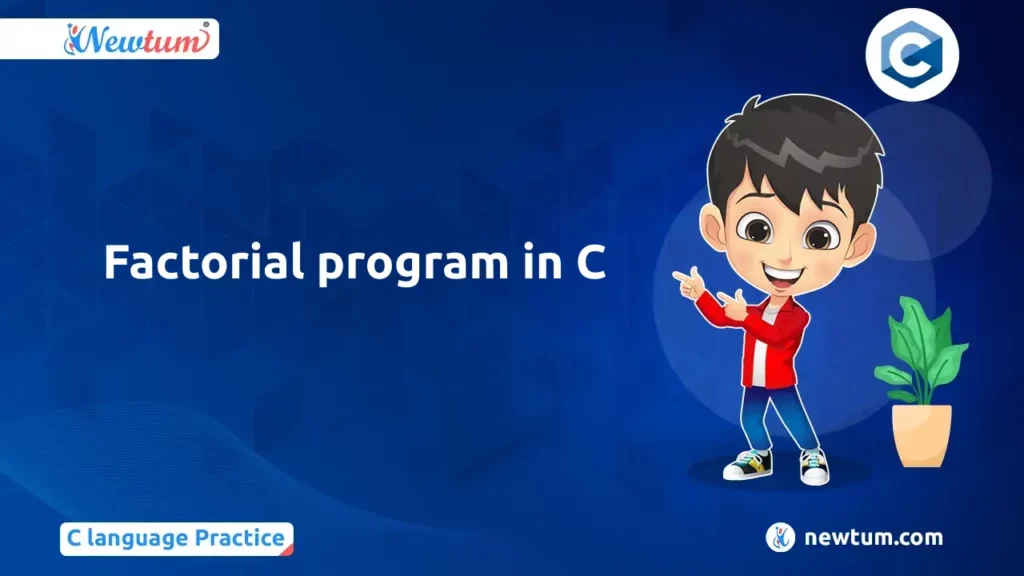
Factorial program in C using Recursion
This recursive approach simplifies factorial calculation by breaking down the problem into smaller sub-problems, making the code concise and elegant. However, excessive recursion for large inputs may lead to stack overflow issues. Take look of given code for understanding of Factorial Program in C using Recursion.
program to find factorial of a number in C using recursion
//include necessary header files #include <stdio.h> //function prototype for factorial function int factorial(int n); //main function int main() { //declare variables int num, result; //get input from user printf("Enter a number: "); scanf("%d", &num); //call factorial function and store the result in 'result' variable result = factorial(num); //print the result printf("Factorial of %d is %d", num, result); return 0; } //recursive function to calculate factorial int factorial(int n) { //base case if (n == 0) return 1; //recursive call else return n * factorial(n - 1); }
Explanation of the code-
This C program calculates the factorial of a given number using recursion. Here’s a breakdown of the code:
1. Header Files: The necessary header file `<stdio.h>` is included to use standard input and output functions.
2. Function Prototype: The `factorial()` function prototype is declared before the `main()` function. This informs the compiler about the function’s signature.
3. Main Function:
– Variables `num` and `result` are declared to store user input and the factorial result, respectively.
– The user is prompted to enter a number, and the input is stored in the `num` variable.
– The `factorial()` function is called with `num` as an argument, and the returned result is stored in the `result` variable.
– The factorial result is printed using `printf()`.
4. Factorial Function (Recursive):
– The `factorial()` function takes an integer `n` as input and returns an integer.
– It serves as a recursive function to calculate the factorial.
– In the base case (when `n` is 0), the function returns 1.
– Otherwise, it recursively calls itself with `n-1` until the base case is reached, multiplying `n` with the result of the recursive call.
Output:
Enter a number: 7
Factorial of 7 is 5040
Factorial program in C using Function
The Factorial program in C using Function calculates the factorial of a given number by employing a user-defined function. It provides a structured approach, enhancing code organization and readability. This method encapsulates the factorial logic into a reusable unit, promoting efficient and scalable programming practices.
Program to find factorial of a number in C using Function
//include standard input/output library #include <stdio.h> //function to calculate factorial int factorial(int n) { //declare and initialize result variable int result = 1; //loop to multiply numbers from 1 to n for(int i = 1; i <= n; i++) { result *= i; } //return the result return result; } //main function int main() { //declare and initialize input variable int n; //prompt user to enter a number printf("Enter a number: "); //read input from user scanf("%d", &n); //call factorial function and store the result in a variable int fact = factorial(n); //print the result printf("Factorial of %d is %d", n, fact); //return 0 to indicate successful execution return 0; }
Explanation of the code-
Below is the step-by-step explanation of the provided C program for calculating factorial using a for loop:
1. Header File: The program includes the `<stdio.h>` header file for using standard input/output functions.
2. Factorial Function:
– The `factorial()` function takes an integer `n` as input and returns an integer, which is the factorial of `n`.
– It initializes a variable `result` to 1 to store the factorial.
– Using a for loop, it iterates from 1 to `n`, multiplying each number with `result`.
– After the loop, the calculated factorial value is returned.
3. Main Function:
– The `main()` function:
– Declares an integer variable `n` to store user input.
– Prompts the user to enter a number.
– Reads the input number using `scanf()`.
– Calls the `factorial()` function with the input number and stores the result in the variable `fact`.
– Prints the calculated factorial using `printf()`.
4. Execution:
– When the program is executed, it prompts the user to enter a number.
– Upon entering the number, the program calculates its factorial using the `factorial()` function.
– Finally, it displays the result using `printf()`.
Output:
Enter a number: 14
Factorial of 14 is 1278945280
Factorial program in C using While Loop
The factorial program in C using a while loop calculates the factorial of a given number iteratively. It initializes a result variable to 1 and multiplies it with each number from 1 to the input number using a while loop. Finally, it prints the calculated factorial.
Program to find factorial of a number in C using While loop
#include <stdio.h> //include standard input/output library int main() { //main function int num, factorial = 1; //declare variables for input and factorial printf("Enter a number: "); //prompt user to enter a number scanf("%d", &num); //read input from user and store in num variable while (num > 0) { //while loop to calculate factorial factorial *= num; //multiply factorial by num num--; //decrement num by 1 } printf("Factorial of %d is %d", num, factorial); //print the result return 0; //return 0 to indicate successful execution }
Explanation of the code-
Here’s the step-by-step explanation of the provided C program for calculating factorial using a while loop:
1. Header File Inclusion: The program includes the `<stdio.h>` header file for using standard input/output functions.
2. Main Function: Declares two integer variables `num` and `factorial`. Prompts the user to enter a number and reads the input using `scanf()`.
3. While Loop for Factorial Calculation: Enters a while loop with the condition `num > 0`. Multiplies `factorial` by `num` and decrements `num` by 1 in each iteration until `num` becomes 0.
4. Result Printing: After the loop, it prints the calculated factorial using `printf()`.
5. Execution: Upon execution, the program calculates the factorial of the entered number using the while loop and displays the result.
Output:
Enter a number: 11
Factorial of 0 is 39916800
Factorial program in C using For Loop
The factorial program in C using a for loop calculates the factorial of a given number efficiently. By iterating through the numbers from 1 to the input number, it multiplies each value to calculate the factorial. This approach provides a simple and concise solution for factorial computation in C.
Program to find factorial of a number in C using for loop
#include <stdio.h> //include standard input/output library int main() { //main function int num, fact = 1; //declare variables for number and factorial, initialize factorial to 1 printf("Enter a number: "); //prompt user to enter a number scanf("%d", &num); //read input from user and store in num variable for(int i = 1; i <= num; i++) { //for loop to iterate from 1 to num fact *= i; //multiply factorial by current value of i } printf("Factorial of %d is %d", num, fact); //print the factorial of the input number return 0; //return 0 to indicate successful execution }
Here’s a concise explanation of the provided C program for calculating factorial using a for loop:
1. Header File Inclusion: The program includes `<stdio.h>` for standard input/output functions.
2. Main Function: Declares integer variables `num` and `fact`, initializing `fact` to 1.
3. User Input: Prompts the user to enter a number and reads it using `scanf()`.
4. Factorial Calculation: Executes a for loop from 1 to `num` and then Multiplies `fact` by the loop index `i` in each iteration.
5. Result Printing: Displays the calculated factorial using `printf()`.
6. Execution: Upon execution, it computes and prints the factorial of the entered number.
Output:
Enter a number: 16
Factorial of 16 is 2004189184
Comparison of all method of Factorial Program in C
This table compares all four methods to calculate factorials in C: recursion, using a separate function, while loop, and for loop. Each method has specific advantages and considerations, such as ease of implementation, code structure, efficiency, and applicability for various scenarios.
Method | Description | Factors |
Recursion | Uses a function that calls itself to calculate factorial | Easy to implementElegant and concise code.May lead to stack overflow for large inputs |
Function | Utilizes a separate function to compute factorial | Modular code structurePromotes code reuse. May incur function call overhead |
While loop | Iterates through a loop until factorial is computed | Flexible loop control Suitable for unknown iterationsCan be less efficient for large inputs |
For loop | Iterates through a loop with a predetermined number of iterations | For Loop Iterates through a loop with a predetermined number of iterationsDirect control over loop iterations Typically more efficient Clear loop structure |
In conclusion, this blog explored four methods for calculating factorials in C: recursion, function, while loop, and for loop. Understanding these approaches enhances problem-solving skills and promotes code versatility. Experimenting with the provided code fosters deeper learning, while Newtum offers a platform to explore more programming concepts and languages. Happy coding!
This was brief about different methods of calculating the Factorial program in C, Now excel each method such as factorial program in c using for loop, factorial program in c using function and factorial program in c using while loop.
Factorial Program in C- FAQ
Answer- Recursion offers a concise and elegant solution, simplifying the factorial calculation process by breaking it down into smaller sub-problems.
Answer- The function method encapsulates the factorial logic into a separate reusable unit, enhancing code modularity and readability.
Answer- Excessive recursion can consume large amounts of memory due to function calls being added to the call stack, potentially leading to a stack overflow error.
Answer- The while loop is ideal for scenarios where the number of iterations is not predetermined, providing flexibility in loop control.
Answer- The for loop provides direct control over loop iterations and typically offers a more efficient and clear loop structure for factorial calculation.