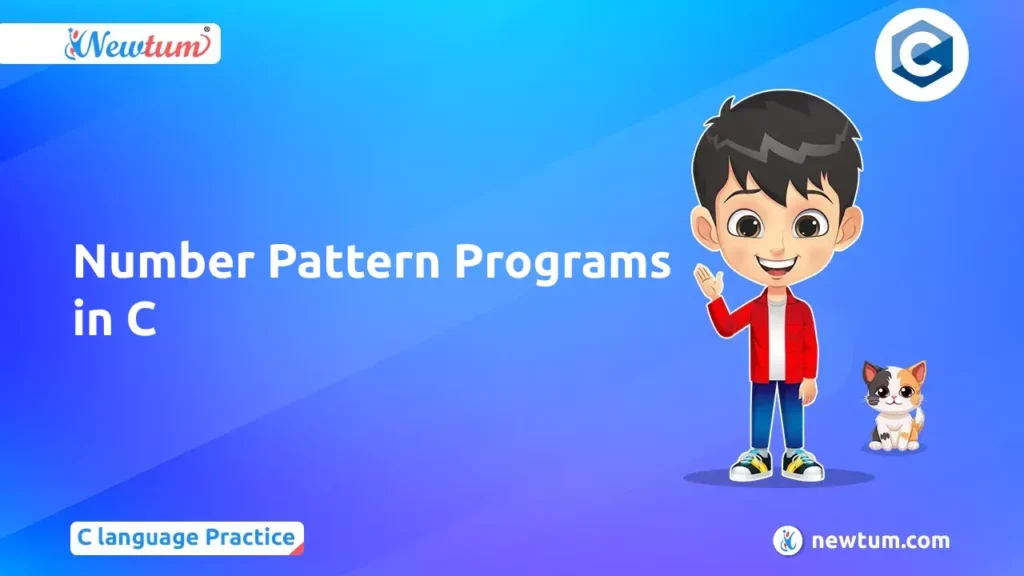
Developing number pattern programs is an important part of learning to program because they improve your problem-solving skills. This article will delve into the world of C programming’s number pattern programs. Let’s dive into C’s number pattern programs to learn, solve problems, and explore.
What are the types of number pattern programs?
In C programming, number pattern programs can be classified into various types based on their shapes, structures, and numerical sequences. Here are some common types of number pattern programs:
Table of Contents
Method 1- Square Number Patterns
#include <stdio.h> int main() { int N, i, j; printf("Enter the number of rows and columns: "); scanf("%d", &N); for (i = 1; i<= N; i++) { for (j = 1; j <= N; j++) { printf("%d ", j); } printf("\n"); } return 0; }
Explanation of the code-
1. User Input: The program prompts the user to enter the number of rows and columns for the pattern. The input value is stored in the variable `N` using the `scanf()` function.
2. Outer Loop (Rows): An outer loop iterates from 1 to `N`, controlling the number of rows in the pattern. Inside this loop, each iteration represents a new row in the pattern.
3. Inner Loop (Columns): Within the outer loop, there’s an inner loop that also iterates from 1 to `N`. This loop controls the number of columns in each row of the pattern.
4. Printing Numbers: Inside the inner loop, the program prints the value of `j`, representing the column number, followed by a space. This prints the numbers 1 to `N` horizontally for each row.
5. Newline: After printing all numbers for a row, a newline character (`\n`) is printed to move to the next line, forming rows in the pattern.
This process continues until all rows have been printed, completing the desired pattern.
Output:
Enter the number of rows and columns: 4
1 2 3 4
1 2 3 4
1 2 3 4
1 2 3 4
Method 2- Triangle Number Patterns
#include <stdio.h> //header file for standard input and output functions int main() { //main function int rows, i, j, num = 1; //declaring variables for number of rows, loop counters and starting number printf("Enter the number of rows: "); //prompting user to enter the number of rows scanf("%d", &rows); //reading the input from user and storing it in 'rows' variable for (i = 1; i <= rows; i++) { //outer loop for rows for (j = 1; j <= i; j++) { //inner loop for columns printf("%d ", num); //printing the current number num++; //incrementing the number } printf("\n"); //printing new line after each row } return 0; //returning 0 to indicate successful execution }
Explanation of the code-
1. User Input: The program prompts the user to enter the number of rows for the triangle pattern. The input value is stored in the variable `rows` using `scanf()` function.
2. Nested Loop: Two nested `for` loops are used to generate the triangle pattern. The outer loop (`i`) controls the number of rows, iterating from 1 to the value of `rows`. The inner loop (`j`) controls the number of columns in each row, iterating from 1 to the value of `i`.
3. Printing Numbers: Within the inner loop, the variable `num` is printed, representing the numbers in the triangle pattern. After printing each number, `num` is incremented by 1 to print the next sequential number.
4. Newline: After printing all numbers for a row, a newline character (`\n`) is printed to move to the next line, forming rows in the triangle pattern.
Output:
Enter the number of rows: 6
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
16 17 18 19 20 21
Method 3 – Pyramid Number Patterns
#include <stdio.h> //include standard input/output library int main() { //main function int rows, i, j, space; //declare variables for number of rows, loop counters, and space printf("Enter the number of rows: "); //prompt user to enter number of rows scanf("%d", &rows); //read input and store in rows variable for (i = 1; i <= rows; i++) { //outer loop for rows for (space = 1; space <= rows - i; space++) { //inner loop for spaces printf(" "); //print space } for (j = 1; j <= i; j++) { //inner loop for numbers printf("%d ", j); //print numbers } printf("\n"); //move to next line } return 0; //return 0 to indicate successful execution }
Explanation of the code-
1. User Input: The program prompts the user to enter the number of rows for the triangle pattern. The input value is stored in the variable `rows` using `scanf()` function.
2. Outer Loop (Rows): An outer loop (`i`) iterates from 1 to the specified number of rows. Each iteration represents a new row in the triangle pattern.
3. Inner Loop (Spaces): Within the outer loop, an inner loop (`space`) controls the number of spaces printed before the numbers in each row. It calculates the number of spaces based on the difference between the total number of rows and the current row number.
4. Printing Spaces: Inside the space loop, spaces are printed using `printf(” “)` to create the indentation for each row.
5. Inner Loop (Numbers): Another inner loop (`j`) is used to print the numbers in each row. It iterates from 1 to the current row number (`i`), printing the numbers sequentially.
6. Newline: After printing all spaces and numbers for a row, a newline character (`\n`) is printed to move to the next line, forming the triangle pattern.
Output:
Enter the number of rows: 6
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
1 2 3 4 5 6
Method 4 – Diamond Number Patterns
#include <stdio.h> //header file for standard input and output functions int main() { //main function int n, i, j; //declaring variables for number of rows and counters printf("Enter the number of rows: "); //prompting user to enter the number of rows scanf("%d", &n); //reading the input and storing it in variable n for (i = 1; i <= n; i++) { //outer loop for printing the upper half of the diamond for (j = 1; j <= n - i; j++) { //inner loop for printing spaces printf(" "); //printing a space } for (j = 1; j <= 2 * i - 1; j++) { //inner loop for printing asterisks printf("*"); //printing an asterisk } printf("\n"); //printing a new line } for (i = n - 1; i >= 1; i--) { //outer loop for printing the lower half of the diamond for (j = 1; j <= n - i; j++) { //inner loop for printing spaces printf(" "); //printing a space } for (j = 1; j <= 2 * i - 1; j++) { //inner loop for printing asterisks printf("*"); //printing an asterisk } printf("\n"); //printing a new line } return 0; //returning 0 to indicate successful execution of the program }
Explanation of the code-
1. User Input: The program prompts the user to enter the number of rows for the diamond pattern. The input value is stored in the variable `n` using `scanf()` function.
2. Upper Half of Diamond: The first `for` loop (`i`) iterates from 1 to `n`, controlling the number of rows in the upper half of the diamond. Within this loop, the number of spaces (`n – i`) and asterisks (`2 * i – 1`) are determined and printed accordingly to form each row.
3. Lower Half of Diamond: After completing the upper half of the diamond, the program enters a second `for` loop to print the lower half. This loop iterates from `n – 1` down to 1, mirroring the upper half’s structure.
4. Printing Spaces and Asterisks: In both halves of the diamond, nested `for` loops (`j`) are used to print spaces and asterisks as per the calculated values.
5. Newline: After printing each row, a newline character (`\n`) is printed to move to the next line, completing the diamond pattern.
Output:
Enter the number of rows: 5
*
***
*****
*******
*********
*******
*****
***
*
Method 5 – Spiral Number Patterns
#include <stdio.h> //include standard input/output library int main() { //main function int n; //declare variable n to store input printf("Enter the number of rows: "); //prompt user to enter number of rows scanf("%d", &n); //read input and store in n int arr[n][n]; //declare 2D array with size n x n int row_start = 0; //initialize starting row index int row_end = n - 1; //initialize ending row index int col_start = 0; //initialize starting column index int col_end = n - 1; //initialize ending column index int num = 1; //initialize number to be printed while (row_start <= row_end && col_start <= col_end) { //loop until starting row and column index are less than or equal to ending row and column index //print top row from left to right for (int i = col_start; i <= col_end; i++) { //loop through columns from starting to ending index arr[row_start][i] = num; //store number in current row and column num++; //increment number } row_start++; //increment starting row index //print right column from top to bottom for (int i = row_start; i <= row_end; i++) { //loop through rows from starting to ending index arr[i][col_end] = num; //store number in current row and column num++; //increment number } col_end--; //decrement ending column index //print bottom row from right to left for (int i = col_end; i >= col_start; i--) { //loop through columns from ending to starting index arr[row_end][i] = num; //store number in current row and column num++; //increment number } row_end--; //decrement ending row index //print left column from bottom to top for (int i = row_end; i >= row_start; i--) { //loop through rows from ending to starting index arr[i][col_start] = num; //store number in current row and column num++; //increment number } col_start++; //increment starting column index } //print spiral pattern printf("Spiral Number Pattern:\n"); for (int i = 0; i < n; i++) { //loop through rows for (int j = 0; j < n; j++) { //loop through columns printf("%d\t", arr[i][j]); //print number at current row and column } printf("\n"); //move to next line } return 0; //return 0 to indicate successful execution }
Explanation of the code-
1. User Input: The program prompts the user to enter the number of rows for the spiral pattern. The input value is stored in the variable `n` using `scanf()` function.
2. Initialize Variables: Variables are initialized to keep track of the starting and ending row and column indices, along with a variable `num` to store the numbers to be printed.
3. Generate Spiral Pattern: Using a while loop, the program iterates through the matrix in a spiral pattern, filling it with numbers incrementally from 1 to `n*n`.
4. Store Numbers: Numbers are stored in the 2D array `arr` at the appropriate row and column indices as the loop progresses.
5. Print Spiral Pattern: After filling the array, the program prints the generated spiral pattern by iterating through the array and printing each number, formatted neatly in rows and columns.
Output:
Enter the number of rows: 4
Spiral Number Pattern:
1 2 3 4
12 13 14 5
11 16 15 6
10 9 8 7
In conclusion, number pattern programs in C are vital for learning programming, improving problem-solving skills, and nurturing creativity. Just as you’ve learned Number Pattern Programs, you can explore other concepts and programming languages with Newtum.