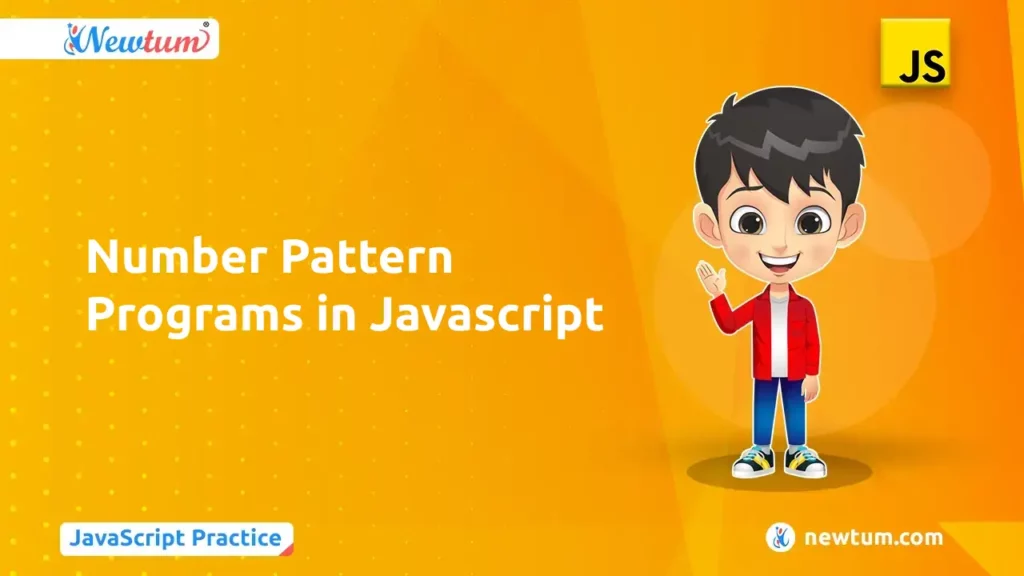
In this blog, dive into the captivating concept of Number Pattern Programs in JavaScript, where you’ll blend math with creativity through coding. Discover the vast range of patterns you can create using JavaScript, from basic shapes like squares and triangles to intricate designs resembling fractals.
Table of Contents
Example code for Javascript Number Pattern
<html> <head> <title>JavaScript Number Patterns</title> <script type="text/javascript"> // Number Pattern Programs in javascript var num; var no=prompt("Please provide a number for the no of rows to be print in a pattern..."); for(var m=1;m<=no;m++) { for(var n=1;n<=m;n++) { document.write("0"+n+" "); } document.write("<br />"); } </script> </head> <body></body> </html>
Explanation of the Code:
This JavaScript code generates number patterns based on user input.
1. It prompts the user to input the number of rows for the pattern.
2. It iterates through each row from 1 to the user-defined number.
3. Within each row loop, it iterates from 1 to the current row number.
4. It prints the current column number with a leading ‘0’ and a space.
5. After each row, it adds a line break to start a new row.
6. The pattern is then displayed on the webpage.
Output:
01
01 02
01 02 03
01 02 03 04
01 02 03 04 05
01 02 03 04 05 06
Basic Number Patterns in JavaScript:
A. Ascending Order Pattern
// Ascending Order Number Pattern in JavaScript <html> <head> <title>JavaScript Number Patterns</title> <script type="text/javascript"> // Number Pattern Programs in javascript var num; var rows=prompt("Please provide a number for the no of rows to be print in a pattern..."); for (let i = 1; i <= rows; i++) { let pattern = ""; // Initialize an empty string for the pattern in each row for (let j = 1; j <= i; j++) { // Loop to generate numbers in ascending order pattern += j + " "; // Concatenate numbers with a space } document.write(pattern); // Output the pattern for each row document.write("<br />"); } </script> </head> <body></body> </html>
Explanation of the code:
Below JavaScript code generates a number pattern in ascending order based on the user-provided number of rows.
1. It prompts the user to input the number of rows for the pattern.
2. It initializes an empty string `pattern` for each row.
3. Using nested loops, it iterates through each row and column to generate the numbers in ascending order.
4. Inside the inner loop, it concatenates the numbers with a space.
5. The pattern for each row is then outputted using `document.write()`.
6. Finally, `<br />` is added to move to the next line for the next row.
This process repeats until the specified number of rows is reached, displaying the ascending number pattern.
Output:
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
B. Descending Order Pattern
// Descending Order Pattern number in javascript <html> <head> <title>JavaScript Number Patterns</title> <script type="text/javascript"> // Number Pattern Programs in javascript var num; var rows=prompt("Please provide a number for the no of rows to be print in a pattern..."); for (var i = rows; i >= 1; --i) { // Initialize an empty string for the current row's pattern var pattern = ''; // Loop to generate numbers in descending order for the current row for (var j = 1; j <= i; ++j) { pattern += j + ' '; } // Output the pattern for the current row to the console document.write(pattern); // Output the pattern for each row document.write("<br />"); } </script> </head> <body></body> </html>
Explanation of the code:
The above JavaScript code generates a descending order number pattern based on user input:
1. Prompt the user to enter the number of rows for the pattern.
2. Iterate from the given number of rows to 1 in a decrementing manner.
3. Within each iteration, initialize an empty string to store the pattern for the current row.
4. Use a nested loop to generate numbers in descending order for the current row, appending each number to the pattern string.
5. Output the pattern for the current row to the document using document.write().
6. Repeat steps 3-5 for each row, printing the pattern on a new line each time.
The above code dynamically creates a descending number pattern based on user input, demonstrating JavaScript’s looping and string manipulation capabilities.
Output:
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
C. Triangle Patterns
// Triangle Pattern number in javascript <html> <head> <title>JavaScript Number Patterns</title> <script type="text/javascript"> // Number Pattern Programs in javascript var num; var rows=prompt("Please provide a number for the no of rows to be print in a pattern..."); for (var i = 1; i <= rows; ++i) { // Initialize an empty string for the current row's pattern var pattern = ''; // Loop to generate numbers for the current row for (var j = 1; j <= i; ++j) { pattern += j + ' '; } document.write(pattern); // Output the pattern for each row document.write("<br />"); } </script> </head> <body></body> </html>
Explanation of the code:
Above given code generates a triangle number pattern based on user input. Below is step-by-step explanation:
1. Prompt the user to enter the number of rows for the triangle pattern.
2. Iterate from 1 to the given number of rows.
3. Within each iteration, initialize an empty string to store the pattern for the current row.
4. Use a nested loop to generate numbers for the current row, appending each number to the pattern string.
5. Output the pattern for the current row to the document using document.write().
6. Repeat steps 3-5 for each row, printing the pattern on a new line each time.
This code dynamically creates a triangle number pattern based on user input, showcasing JavaScript’s looping and string manipulation capabilities.
Output:
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
Advanced Number Patterns in JavaScript:
There are many advanced number patterns in Javascript, but we are only going to learn a few which are the most popular ones. So let’s explore a few codes to understand advanced number patterns in Javascript:
A. Diamond Patterns
// Diamond Pattern number in javascript <!DOCTYPE html> <html> <head> <title>JavaScript Number Patterns</title> </head> <body> <script type="text/javascript"> // Number Pattern Programs in JavaScript var rows = parseInt(prompt("Please provide a number for the number of rows to be printed in the pattern...")); var i, j, space; // Upper part of the diamond for (i = 1; i <= rows; i++) { // Printing spaces for (space = i; space < rows; space++) { document.write(" "); } // Printing numbers in ascending order for (j = 1; j <= i * 2 - 1; j++) { document.write(j + " "); } document.write("<br>"); } // Lower part of the diamond for (i = rows - 1; i >= 1; i--) { // Printing spaces for (space = rows; space > i; space--) { document.write(" "); } // Printing numbers in ascending order for (j = 1; j <= i * 2 - 1; j++) { document.write(j + " "); } document.write("<br>"); } </script> </body> </html>
Explanation of the code:
Code generates a diamond number pattern based on user input – explanation:
1. Prompt the user to enter the number of rows for the diamond pattern.
2. Iterate from 1 to the given number of rows to print the upper part of the diamond.
3. Within each iteration, print the appropriate number of spaces to align the numbers correctly.
4. Print the numbers in ascending order for each row.
5. Repeat steps 2-4 to print the lower part of the diamond in descending order.
6. Output the diamond pattern to the document, with each row separated by line breaks.
Output:
It prints the diamond pattern to the console with numbers arranged in ascending order in each row, forming a diamond shape.
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
B. Pascal’s Triangle
// Pascal's Triangle number in javascript <!DOCTYPE html> <html> <head> <title>Pascal's Triangle Numbers</title> </head> <body> <script type="text/javascript"> // Function to calculate factorial function factorial(n) { if (n === 0 || n === 1) return 1; else return n * factorial(n - 1); } // Function to calculate binomial coefficient function binomialCoefficient(n, r) { return factorial(n) / (factorial(r) * factorial(n - r)); } // Function to generate Pascal's Triangle function generatePascalsTriangle(rows) { var pascalsTriangle = []; for (var i = 0; i < rows; i++) { var row = []; for (var j = 0; j <= i; j++) { row.push(binomialCoefficient(i, j)); } pascalsTriangle.push(row); } return pascalsTriangle; } // Function to display Pascal's Triangle function displayPascalsTriangle(triangle) { for (var i = 0; i < triangle.length; i++) { document.write(triangle[i].join(" ") + "<br>"); } } // Number of rows in Pascal's Triangle var rows = parseInt(prompt("Please enter the number of rows for Pascal's Triangle:")); // Generate Pascal's Triangle var pascalsTriangle = generatePascalsTriangle(rows); // Display Pascal's Triangle displayPascalsTriangle(pascalsTriangle); </script> </body> </html>
Explanation of the code:
1. Define functions to calculate factorial and binomial coefficient.
2. Create a function to generate Pascal’s Triangle by iterating through each row and column.
3. For each row, calculate the binomial coefficient for each element.
4. Store the triangle in an array.
5. Prompt the user to enter the number of rows.
6. Generate Pascal’s Triangle based on the input.
7. Display the triangle by joining each row’s elements and outputting them with line breaks.
Output:
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
C. Floyd’s Triangle
// Floyd's Triangle number in JavaScript <!DOCTYPE html> <html> <head> <title>Floyd's Triangle Numbers</title> </head> <body> <script type="text/javascript"> // Function to generate Floyd's Triangle function generateFloydsTriangle(rows) { var triangle = []; var count = 1; for (var i = 0; i < rows; i++) { var row = []; for (var j = 0; j <= i; j++) { row.push(count); count++; } triangle.push(row); } return triangle; } // Function to display Floyd's Triangle function displayFloydsTriangle(triangle) { for (var i = 0; i < triangle.length; i++) { document.write(triangle[i].join(" ") + "<br>"); } } // Number of rows in Floyd's Triangle var rows = parseInt(prompt("Please enter the number of rows for Floyd's Triangle:")); // Generate Floyd's Triangle var floydsTriangle = generateFloydsTriangle(rows); // Display Floyd's Triangle displayFloydsTriangle(floydsTriangle); </script> </body> </html>
Explanation of the code:
This JavaScript code is based on user input. Here is an explanation:
1. Define a function to generate Floyd’s Triangle, initializing an empty array to store the triangle.
2. Use nested loops to iterate through each row and column, incrementing a counter to populate the triangle.
3. Store each row in the triangle array.
4. Prompt the user to enter the number of rows.
5. Generate Floyd’s Triangle based on the input.
6. Display the triangle by joining each row’s elements and outputting them with line breaks.
Output:
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
Explore the fascinating world of ‘Number Pattern Programs in JavaScript’ and unleash your creativity. Dive into the provided code on Newtum Compiler, experiment, and learn hands-on. Newtum offers user-friendly tutorials and courses to support your learning journey. Keep coding, keep exploring, and enjoy the rewarding experience of programming!