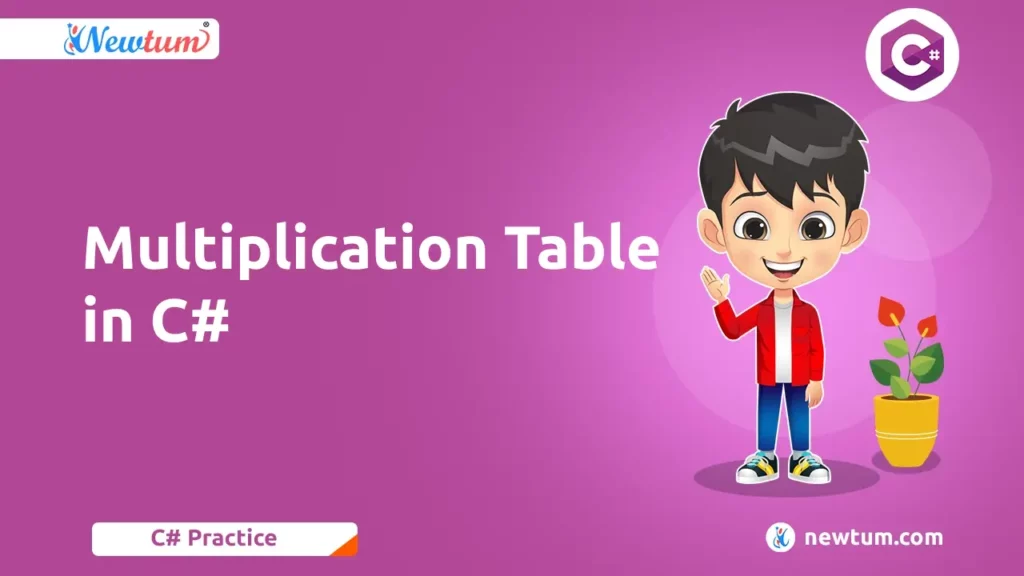
In this blog, we’ll explore how to create a multiplication table in C#. Multiplication tables are grids of numbers that show the results of multiplying two factors. Understanding them is important for math and programming.
Multiplication table in C# – Code
Creating a multiplication table in C# involves generating a grid of numbers by using loops. It’s a fundamental concept in programming and math, aiding in understanding arithmetic operations.
// multiplication table in C# using System; namespace DisplayMultiplicationTable { class Program { static void Main(string[] args) { Console.WriteLine("Multiplication Table"); Console.Write("Enter the table number:\t"); int n = int.Parse(Console.ReadLine()); int i; for(i=1;i<=10;i++) { int product = n * i; Console.Write("{0} X {1} = {2} \n",n,i,product); } } } }
Explanation of the code:
1. The code utilizes the `System` namespace, which provides access to the Console class for input/output operations.
2. The `Main` method serves as the entry point of the program.
3. The user is prompted to enter the number for which the multiplication table needs to be generated. The input is read using `Console.ReadLine()` and parsed into an integer variable `n`.
4. Multiplication Table Generation:
A `for` loop is used to iterate from 1 to 10, generating each row of the multiplication table.
Within each iteration, the product of the input number (`n`) and the loop counter (`i`) is calculated and stored in the variable `product`.
The result is then printed in the format `{number} X {iteration} = {product}` using `Console.Write()`.
5. Once all products are printed, the program execution ends.
Learn more about the Factorial of a number in PHP today!
Output:
Multiplication Table
Enter the table number:4
4 X 1 = 4
4 X 2 = 8
4 X 3 = 12
4 X 4 = 16
4 X 5 = 20
4 X 6 = 24
4 X 7 = 28
4 X 8 = 32
4 X 9 = 36
4 X 10 = 40
Customization Options for C# Multiplication Table
Our previous exploration covered the basics of creating a multiplication table in C#.
A. User Input for Table Size:
- Challenge: The default table size might not always be ideal.
- Solution: Prompt the user to enter their desired table size (number of rows and columns) using Console.ReadLine().
- Implementation: Inside your for loops, use the user-provided input as the loop condition to determine the number of iterations.
B. Starting Number Configuration:
- Challenge: The table traditionally starts at 1.
- Solution: Allow users to define the starting number for the multiplication sequence.
- Implementation: Introduce a variable to store the user’s chosen starting number. Modify the inner loop’s condition to iterate from the chosen starting number instead of 1.
C. Displaying Specific Ranges:
- Challenge: Displaying the entire table might not be necessary.
- Solution: Implement user input to specify the starting and ending numbers for both rows and columns. This allows users to generate a custom sub-section of the multiplication table.
- Implementation: Collect user input for starting and ending values. Modify your for loops to iterate within these specified ranges.
Advance Customization in Multiplication table in C#
These are just a few ways to customize your multiplication table program. You can further explore:
- Formatting: Enhance the table’s appearance by adjusting spacing, alignment, and adding borders using string manipulation techniques.
- Headers and Labels: Include clear headers for rows and columns to improve readability.
- Error Handling: Implement validation to handle invalid user input (e.g., negative numbers or non-numeric characters).
Learn more about the Multiplication Table in Python Using Recursion and Multiplication Table in Python Using Function now!
Advantages of creating Multiplication table in C#
Creating a multiplication table in C# offers several benefits:
1. Enhanced Understanding: Building a multiplication table enhances comprehension of arithmetic operations and patterns, promoting a deeper understanding of mathematical concepts.
2. Practical Application: Multiplication tables are crucial tools in real-world scenarios like budgeting, engineering calculations, and data analysis.
3. Problem-Solving and Transferable Skills: Generating a multiplication table in C# enhances problem-solving skills through logical thinking and algorithmic understanding, transferring skills like loop usage and data manipulation to other programming tasks.
4. Programming Proficiency: C# programming proficiency is enhanced by creating a multiplication table through loop implementation, user input handling, and output formatting.
5. Interactive Learning: Incorporating user input and interactive features into the multiplication table program engages users and facilitates interactive learning experiences.
6. Customization and Flexibility: C# offers developers the ability to customize multiplication tables to meet specific requirements, such as adjusting table size, starting number, or displaying specific ranges.
In conclusion, our blog has delved into the educational and practical significance of multiplication tables in C#. We encourage readers to experiment with the provided code on the Newtum Compiler, fostering hands-on learning. Explore Newtum’s tutorials and courses for further programming insights, and embark on a rewarding journey of coding proficiency. Happy coding!
Multiplication table in C# – FAQ
Answer: Multiplication tables aid in understanding arithmetic operations, loop structures, and problem-solving techniques in programming.
Answer: Yes, you can customize the table size by adjusting the loop conditions and user input handling.
Answer: Multiplication tables are used in various real-world scenarios such as budgeting, engineering calculations, and data analysis.
Answer: It enhances problem-solving skills, logical thinking, and understanding of programming concepts like loops and user input handling.
Answer: Newtum offers tutorials and courses on C# programming and other programming languages to help you enhance your skills.