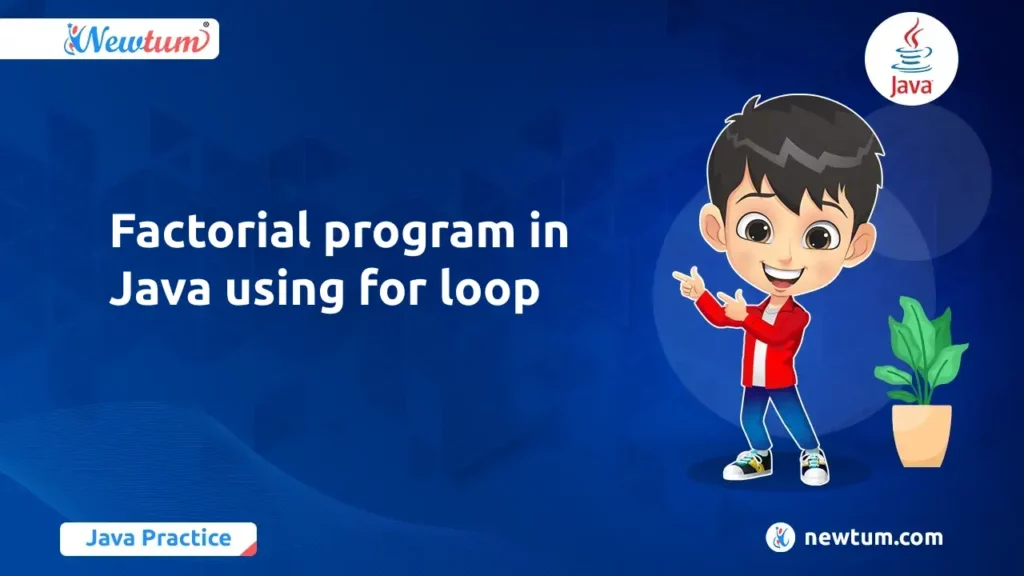
The Java factorial program uses a for loop to efficiently calculate factorials by iterating through numbers and multiplying each one, providing simplicity and clarity. In this blog, we will learn about finding the factorial program in Java using a for loop, through the sample code explained along output. In this blog, we will explain how to write a program to find factorial of a number in Java using a for loop, with sample code and output explanations.
Java Program to find factorial of a number using for loop
For instance, if we wish to determine the factorial of 8, the following code will provide the answer.
// Factorial program in java using for loop import java.util.Scanner; public class factorialEx { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number: "); int number = sc.nextInt(); long fact = 1; for(int i = 1; i <= number; i++) { fact = fact * i; } System.out.println("Factorial of "+number+" is: "+fact); } }
Explanation of the code-
Step-by-step explanation of the provided Java code for calculating the factorial using a for loop:
1. Import Scanner Class: The code begins with importing the `Scanner` class from the `java.util` package to read input from the user.
2. Declare Class and Main Method: The class `factorialEx` is declared with the `main` method as the entry point of the program.
3. Create Scanner Object: An instance of the `Scanner` class named `sc` is created to read input from the user.
4. Prompt User for Input: The program prompts the user to enter a number using `System.out.print` and `sc.nextInt()` reads the input number provided by the user.
5. Initialize Variables: Variables `number` to store the input number and `fact` to store the factorial are declared and initialized to 1.
6. Calculate Factorial Using For Loop: A for loop is used to iterate from 1 to the input number (`i <= number`). In each iteration, the `fact` variable is multiplied by the loop counter `i`.
7. Print Result: After the loop completes, the program prints the calculated factorial using `System.out.println`, concatenating the input number and its factorial.
This code efficiently calculates the factorial of the input number using a for loop and displays the result to the user.
Output:
Enter the number: 8
Factorial of 8 is: 40320
Learn the same concept in different programming languages such as the factorial of a number in C#
Why factorials are useful in mathematics and programming?
- Factorials are mathematical operations that involve multiplying a given number by all positive integers less than or equal to it.
- They are denoted by the symbol “!” and are commonly used in combinatorial mathematics and probability theory.
- Factorials are useful for calculating the number of permutations and combinations in various scenarios.
- In programming, factorials are often used in algorithms and mathematical computations, such as calculating the number of ways to arrange a set of objects or solving problems involving arrangements and permutations.
- They provide a concise way to express and compute the number of possible outcomes in a given scenario, making them an essential concept in both mathematics and programming.
Overview of the factorial program its purpose and how it works
A factorial program is designed to calculate the factorial of a given number. The factorial of a non-negative integer n is the product of all positive integers less than or equal to n. For example, the factorial of 5 (denoted as 5!) is calculated as 5 x 4 x 3 x 2 x 1, which equals 120.
The program typically takes a number as input and computes its factorial using a loop or recursive function. Here’s how it generally works:
1. Input: The program prompts the user to enter a number for which the factorial needs to be calculated.
2. Calculation: It then calculates the factorial using either a loop (such as a for loop or a while loop) or a recursive function. The calculation involves multiplying the number by all positive integers less than it, down to 1.
3. Output: Finally, the program displays the factorial of the input number as the output.
Tips and Tricks for Coding
Check out some tips and tricks for better implementation of the factorial program in Java using a for loop, along with scenarios where they can be applied effectively:
1. Input Validation: Ensure that the input provided by the user is valid and within the acceptable range. Prompt the user to re-enter the input if it is negative or exceeds a certain limit.
2. Handling Edge Cases: Consider edge cases such as factorial of 0 or 1 separately, as their results are predefined. This can help improve the efficiency of the program and make it more readable.
3. Optimizing Loop: Optimize the for loop by starting from 2 instead of 1, as the factorial of 1 is always 1. This can save unnecessary iterations and improve performance, especially for larger numbers.
4. Using Data Types: Choose appropriate data types based on the expected range of input values. For example, use ‘long’ instead of ‘int’ to accommodate larger factorial values without overflow.
5. Error Handling: Implement error handling mechanisms to gracefully handle exceptions such as input mismatch or arithmetic overflow. Provide informative error messages to guide users in case of incorrect input.
6. Modularization: Break down the factorial calculation logic into separate methods or functions for better code organization and reusability. This can enhance readability and maintainability, especially in larger projects.
7. Testing: Test the program with various input values, including boundary cases and edge cases, to ensure its correctness and robustness. Consider using automated testing frameworks for thorough testing coverage.
By following these tips and considering different scenarios, you can create a more robust and efficient factorial program in Java using a for loop.
In conclusion, the factorial program in Java using a for loop offers a practical way to calculate factorials efficiently. Understanding this concept enhances problem-solving skills. Explore the treasure of coding knowledge through blogs and various courses on the Newtum website to broaden your understanding and become a proficient programmer.
Factorial program in Java using for loop- FAQ
Answer- A factorial of a non-negative integer n is the product of all positive integers less than or equal to n, denoted by n!.
Answer- The Java program calculates factorials using a for loop, iterating through numbers from 1 to the input number and multiplying them.
Answer- Factorials are useful for solving problems involving permutations, combinations, and mathematical computations in algorithms.
Answer- Input validation ensures that the user provides valid input within an acceptable range, enhancing the program’s robustness and usability.
Answer- The for loop can be optimized by starting from 2 instead of 1 to skip unnecessary iterations, improving performance for larger input values.