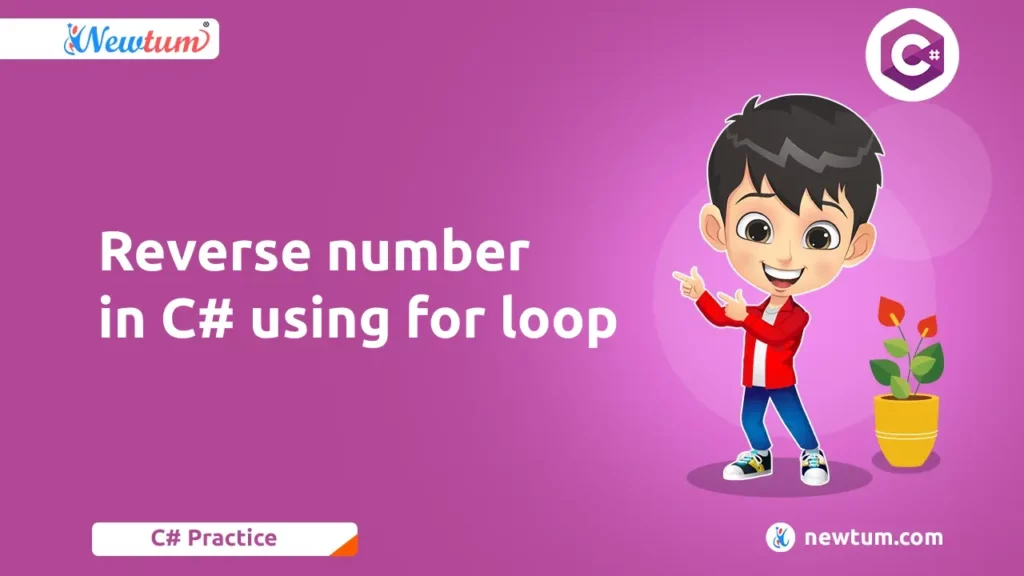
In this blog, we’ll explore how to reverse a number in C# using a for loop. We’ll dive deep into the concept, provide detailed code examples, explain the underlying algorithm, and discuss practical applications. By the end of this journey, you’ll have a solid understanding of number reversal in C# and its significance in programming.
Reverse Number in C# using for loop- Algorithm
Learn the algorithm of Reverse number in C# using for loop involves breaking down the process into simple steps:
1. Initialization: Begin by initializing variables to store the original number and the reversed number. Additionally, determine the number of digits in the original number, which helps in controlling the loop.
2. Iterating Through Digits: Use a for loop to iterate through each digit of the original number. Start from the last digit and move towards the first digit.
3. Extracting Digits Within each iteration, extract the current digit from the original number using arithmetic operations.
4. Appending Digits: Append the extracted digit to the reversed number, ensuring proper placement based on position.
5. Displaying the Reversed Number: After iterating through all digits, display the reversed number to the user.
Reverse Number in C# using for loop- Code
Below is given code of the reverse number in C# using for loop
//Reverse Number in C# using for loop// using System; class Program { static void Main(string[] args) { int number = 1234567; int reversedNumber = 0; for (int i = number; i != 0; i /= 10) { int digit = i % 10; reversedNumber = (reversedNumber * 10) + digit; } Console.WriteLine("Reversed number: " + reversedNumber); } }
Explanation of the Code:
1. We initialize the original number `number` to 12345 and the reversed number `reversedNumber` to 0.
2. The for loop iterates through each digit of the original number. It starts from `number` and continues until it becomes 0, reducing `number` by a factor of 10 in each iteration.
3. Within each iteration, the modulo `%` operator extracts the last digit of `number`, which is then appended to `reversedNumber`.
4. Finally, the reversed number is displayed to the user using `Console.WriteLine()`.
Output:
Reversed number: 7654321
Explore the fascinating world of Reverse number program in PHP using while loop, Now!
Reverse number in C# using for loop-Practical Applications
The ability to reverse numbers in C# using a for loop has numerous practical applications:
1. Data Processing: Reversing numbers is essential in tasks like interpreting data in reverse chronological order or processing log files.
2. Cryptography: Reversing numbers can be used in cryptographic algorithms for encryption and decryption.
3. Mathematical Computations: Reverse numbers are utilized in mathematical computations and algorithmic challenges to solve specific problems efficiently.
4. User Interface Design: Reversed numbers can enhance user interface elements like countdown timers and progress bars, improving user experience.
5. Game Development: Reverse numbers can be used in game mechanics such as scoring systems and countdown timers to enhance gameplay.
Common Errors and Solutions for Reverse Number in C# using for loop:
1. Error: Infinite loop.
Cause: Missing or incorrect termination condition in the for loop.
Solution: Verify the termination condition to ensure it will eventually evaluate to false.
Example:
for (int i = 0; i >= 0; i++) // Incorrect termination condition
{
// Code logic
}
2. Error: Incorrect reversal.
Cause: Flawed logic for reversing the number within the for loop.
Solution: Review the reversal logic to ensure proper manipulation of digits.
Example:
int reversedNumber = 0;
for (int i = number; i > 0; i /= 10)
{
reversedNumber = (reversedNumber * 10) + (i % 10); // Correct reversal logic
}
3. Error: Variable initialization issue.
Cause: Variables used in the for loop are not properly initialized.
Solution: Ensure all variables are initialized before entering the for loop.
Example:
int number;
  for (int i = 0; i < 10; i++)
  {
    number += i; // Variable 'number' not initialized
  }
4. Error: Type conversion error.
Cause: Incorrect data type conversions for variables involved in the reversal process.
Solution: Validate data type conversions to prevent unexpected behavior.
Example:
int digit = num % 10; // Correct data type conversion
Don’t stop just by learning this concept, also learn Reverse number program in PHP using for loop
5. Error: Compilation error.
Cause: Syntax errors or incorrect statement structure.
Solution: Check for syntax errors and ensure all statements are properly formatted.
Example:
for (int i = 0; i < 10; i++) // Correct syntax
  {
    // Code logic
  }
In conclusion, delving into reverse number in C# using for loop enhances coding skills and problem-solving abilities. Experimentation is key to mastery, and Newtum offers accessible tutorials in various programming languages, fostering continuous learning. Keep coding, exploring, and enjoy the journey of mastering programming.
FAQ about Reverse number in C# using for loop
Answer: Reversing numbers in C# is essential for various applications like data processing, cryptography, and mathematical computations.
Answer: The for loop in C# allows us to iterate through each digit of the number and manipulate them to achieve reversal efficiently.
Answer: Yes, while loops can also be used, but for loops offer more concise control over iterations and are often preferred for such tasks.
Answer: Common errors include infinite loops, incorrect reversal logic, variable initialization issues, type conversion errors, and compilation errors.
Answer: You can prevent errors by carefully reviewing your code, validating variable initialization, data type conversions, and loop termination conditions.
Answer: You can explore tutorials and courses on platforms like Newtum, which offer comprehensive learning resources in various programming languages, including C#.