In today’s blog post, we are diving into the programming world to understand the intricacies of the ‘Average Percentage Calculator in JavaScript’. This JavaScript program is built to calculate the average percentage, a task that’s commonly needed in various fields. The program takes user input, performs the computations, and returns the average percentage. Understanding this program will not only enhance your JavaScript skills but also provide you with an essential tool that can be used in numerous applications. Be it in education to calculate average grades, in finance to calculate returns or in analytics to calculate user engagement. This program showcases the flexibility and power of JavaScript. Let’s dive in!
Average Percentage Calculator in Javascript Program
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Average Percentage Calculator</title> <!-- This is the CSS code for styling the input form --> <style> form { text-align: center; margin-top: 50px; } label { display: inline-block; width: 150px; text-align: left; } input[type="number"] { width: 50px; } input[type="button"] { margin-top: 10px; padding: 5px 10px; font-size: 16px; cursor: pointer; } #result { margin-top: 20px; font-size: 18px; font-weight: bold; text-align: center; } </style> </head> <body> <!-- This is the HTML code for the input form --> <form> <label for="math">Math Percentage:</label> <input type="number" id="math" name="math" min="0" max="100"><br><br> <label for="science">Science Percentage:</label> <input type="number" id="science" name="science" min="0" max="100"><br><br> <label for="english">English Percentage:</label> <input type="number" id="english" name="english" min="0" max="100"><br><br> <label for="history">History Percentage:</label> <input type="number" id="history" name="history" min="0" max="100"><br><br> <input type="button" value="Calculate" onclick="calculateAverage()"><br><br> </form> <!-- This is where the result will be displayed --> <div id="result"></div> <!-- This is the JavaScript code for calculating the average percentage --> <script> // Function to calculate the average percentage function calculateAverage() { // Get the input values from the form var math = document.getElementById("math").value; var science = document.getElementById("science").value; var english = document.getElementById("english").value; var history = document.getElementById("history").value; // Calculate the average percentage var average = (parseFloat(math) + parseFloat(science) + parseFloat(english) + parseFloat(history)) / 4; // Display the result document.getElementById("result").innerText = "The average percentage is: " + average.toFixed(2) + "%"; } </script> </body> </html>
Explanation of the Code:
This HTML document creates a simple average percentage calculator. The form collects percentages for Math, Science, English, and History.
- Each input field is a number input restricted between 0 and 100. When the “Calculate” button is clicked, the
calculateAverage()
JavaScript function is triggered. - This function retrieves the input values, converts them to floats, calculates their average, and displays the result.
- The form and result are styled using CSS for better readability and user experience.
- The result is shown in the
div
with the IDresult
, displaying the average percentage formatted to two decimal places. - This tool is useful for students and educators to quickly compute average percentages.
Output
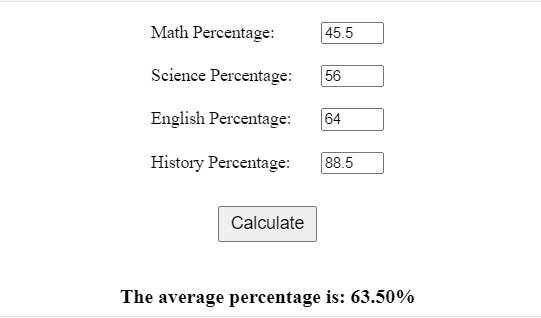
Debugging Common Issues
When developing the Average Percentage Calculator in JavaScript, you may encounter several common issues. Here’s a guide to help you debug these problems effectively:
1. Incorrect Output:
- Issue: The calculated average percentage is not accurate.
- Solution: Ensure that you are correctly summing up the percentages and dividing by the number of subjects. Check for any off-by-one errors in loops or incorrect variable initializations.
2. NaN (Not a Number) Errors:
- Issue: The output shows “NaN” instead of the average percentage.
- Solution: This usually occurs when non-numeric values are processed. Verify that all input values are parsed as floats using
parseFloat()
and check for empty inputs or non-numeric characters.
3. Input Validation:
- Issue: Users can input values outside the expected range (e.g., negative percentages or values over 100).
- Solution: Add input validation to ensure that values are between 0 and 100. Use conditional statements to alert the user if inputs are invalid.
4. Unresponsive Button:
- Issue: The “Calculate” button does not trigger the calculation.
- Solution: Ensure that the
onclick
event is correctly set up. Verify the function name and check for any JavaScript errors in the console that may be preventing the function from executing.
5. Incorrect Display:
- Issue: The result is not displayed or updated correctly.
- Solution: Check that you are targeting the correct HTML element to display the result. Ensure that the element’s inner text is updated within the function and that there are no typos in the element ID.
6. Browser Compatibility:
- Issue: The program does not work as expected in all browsers.
- Solution: Test your program in multiple browsers to ensure compatibility. Use standard JavaScript functions and avoid browser-specific features that may not be supported universally.
By systematically addressing these common issues, you can ensure that your Average Percentage Calculator functions correctly and provides accurate results.
Introducing the Average Percentage Formula
The Average Percentage formula is a simple yet fundamental concept in mathematics. It is used to calculate the average of a set of percentages, which is essential in various fields like education, finance, analytics, and more. The formula requires adding up all the percentages and then dividing by the number of percentages to find the average. This calculation can help you understand the overall status or performance when you have multiple percentages for different areas. For instance, in education, it can help calculate a student’s overall performance by averaging his or her scores in different subjects. Understanding and mastering this formula is not just important for students but for anyone dealing with data and analytics.
Understanding the Concept and Formula of Average Percentage: A Prerequisite for Programming
Before we delve into writing the ‘Average Percentage Calculator in JavaScript’, it is crucial to have depth understanding of the concept of Average Percentage. The average percentage is calculated by adding all the percentages and dividing by the total number of percentages. It is a highly useful formula in various fields like education, finance, and analytics. To understand the formula in detail, please refer to ‘Average Percentage Formula‘. Similarly, if you are interested in understanding the area of triangle, you can use the ‘Average Percentage Calculator‘ tool to play around and learn.
In conclusion, the ‘Average Percentage Formula’ is a fundamental mathematical formula that has been beautifully translated into a ‘JavaScript Program’. This program not only aids in calculating average percentages but also opens up a world of possibilities for integrating this functionality into other applications. As we have seen, understanding this formula and its programming counterpart can lead to enhanced analytical skills and a deeper understanding of data. So, embrace the ‘Average Percentage Formula’, master it, and let it pave the way to your success in programming and beyond.
FAQs about Average Percentage Calculator in Javascript
The ‘Average Percentage Calculator in JavaScript’ is a program designed to compute the average percentage of given values using JavaScript.
It takes user inputs for percentages, calculates their average, and displays the result.
Ensure all input values are valid numbers and are parsed correctly with parseFloat().
Verify that the onclick event is properly set up and that there are no JavaScript errors in the console.
Yes, by modifying the HTML form and JavaScript function to include additional input fields.