Programming is often about repetition – performing the same task multiple times with variations. This is where loops come into play. Among the various loop types, the ‘For Loop’ is versatile and widely used in C programming. In this blog, we will dive into the intricacies of the ‘For Loop’ in C. We will start by understanding its syntax, explore its flow diagram, and then apply our knowledge through a practical example. By the end of this journey, you’ll have a deep understanding of ‘For Loops’ and how to use them effectively in your C programs.
Understanding the Syntax of the ‘For Loop’
Before we embark on our exploration of the ‘For Loop,’ let’s get familiar with its syntax. The ‘For Loop’ comprises three essential components:
1. Initialization statement
2. Conditional statement
3. Increment or decrement statement
Here’s the basic structure of a ‘For Loop’ in C:
for (Initialization; Condition; Increment) { // Code to be executed repeatedly }
– The ‘Initialization’ statement sets the loop control variable and initializes it to a specific value.
– The ‘Condition’ is a Boolean expression that defines when the loop should continue or terminate.
– The ‘Increment’ statement updates the loop control variable after each iteration.
Each of these components is essential for controlling the flow of the ‘For Loop’ effectively.
Flow Diagram of the ‘For Loop’
Visualizing how a ‘For Loop’ operates can be incredibly helpful. Let’s take a look at a flow diagram that represents the execution of a ‘For Loop’:
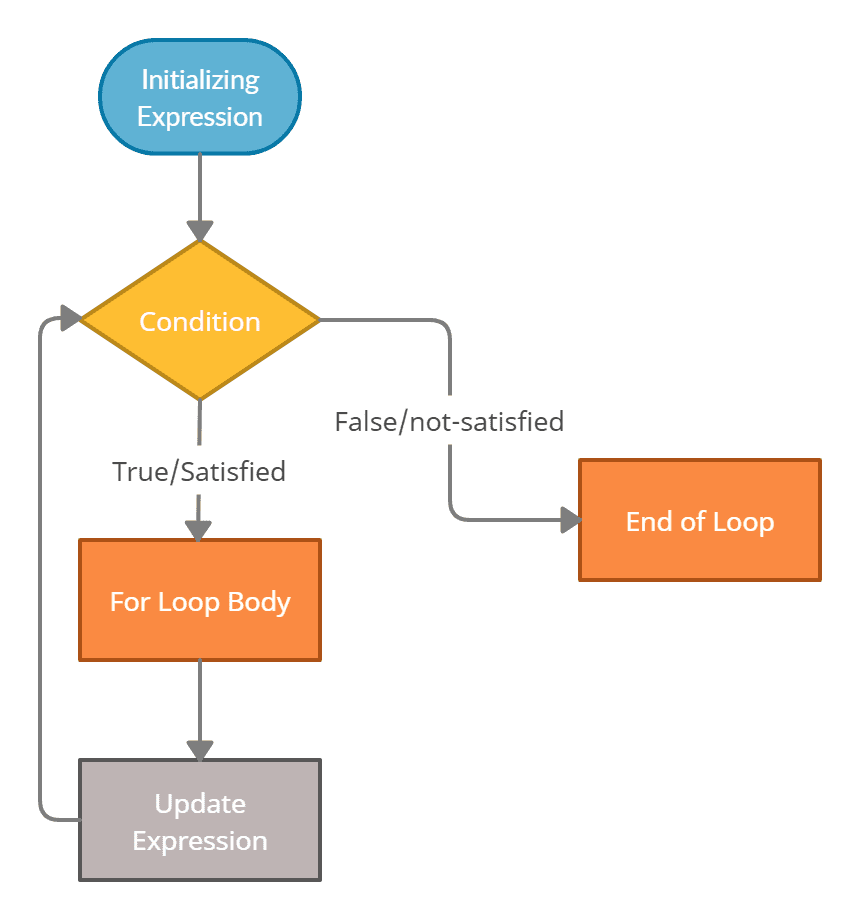
The flow diagram illustrates the key steps:
1. Initialization: The loop control variable is initialized.
2. Condition Check: The condition is evaluated. If it’s true, the loop proceeds; otherwise, it exits.
3. Loop Body: The code within the loop is executed.
4. Increment: After each iteration, the loop control variable is updated.
5. Condition Check (again): The condition is checked again. If true, the loop repeats the process; otherwise, it terminates.
Practical Example: Calculating the Sum of Numbers
Now that we have a solid grasp of the ‘For Loop’ syntax and its flow, let’s put this knowledge into practice. We’ll work through a practical example of using a ‘For Loop’ to calculate the sum of numbers from 1 to N.
Problem Statement:
Suppose you need to find the sum of all numbers from 1 to N, where N is a positive integer provided by the user.
Solution:
#include <stdio.h> int main() { int N, sum = 0; printf("Enter a positive integer N: "); scanf("%d", &N); for (int i = 1; i <= N; i++) { sum += i; } printf("The sum of numbers from 1 to %d is %d\n", N, sum); return 0; }
In this example, we:
- Initialize a variable ‘sum’ to store the sum of numbers.
- Prompt the user to input a positive integer ‘N.’
- Use a ‘For Loop’ to iterate from 1 to ‘N’ and add each number to ‘sum.’
- Display the final sum.
Let’s say the user enters ‘5’ as ‘N.’ The program will then calculate and display the sum of numbers from 1 to 5, which is 15.
This example demonstrates how the ‘For Loop’ simplifies repetitive tasks, making it an essential tool in programming.
‘For Loop’ Variations and Use Cases
The ‘For Loop’ is a versatile construct with various applications and variations. Let’s explore a few of them:
1. Nested ‘For Loops’ and Multi-Dimensional Arrays:
‘For Loops’ can be nested within one another to work with multi-dimensional arrays. This is particularly useful when dealing with tables, grids, or matrices.
for (int i = 0; i < rows; i++) { for (int j = 0; j < columns; j++) { // Access and process elements of a 2D array } }
2. Looping with Different Increment Styles:
The ‘For Loop’ isn’t limited to a simple increment by 1. You can customize the increment or decrement value to match the requirements of your specific task.
for (int i = 0; i < 10; i += 2) { // Loop with a step size of 2 }
3. Using ‘For Loops’ in Real-World Scenarios:
‘For Loops’ are invaluable in real-world applications, from processing data in arrays to iterating through files and directories. Whether you’re calculating averages, searching for specific values, or performing data transformations, ‘For Loops’ can streamline your code and enhance efficiency.
‘For Loops’ can be adapted to suit various scenarios, making them an indispensable tool for programmers.
Tips and Best Practices
To make the most of ‘For Loops’ in your programming endeavors, consider the following tips and best practices:
1. Use Meaningful Variable Names: Choose descriptive variable names to enhance code readability and maintainability. Instead of ‘i’ or ‘j,’ use names that indicate their purpose.
2. Ensure Loop Termination: Carefully evaluate your loop’s conditional statement to ensure it terminates as expected. A mistake here can lead to infinite loops.
3. Initialize Variables Properly: Initialize loop control variables before using them to prevent unexpected behavior.
4. Use Comments: Add comments to clarify the purpose of the ‘For Loop’ and its components, especially if the loop is complex or the logic isn’t immediately obvious.
5. Test and Debug: Thoroughly test your ‘For Loop’ to ensure it functions as intended. Use debugging tools and step through your code to identify and rectify errors.
6. Optimize Performance: While coding, consider ways to optimize your ‘For Loops’ for better performance. Reducing unnecessary calculations or minimizing I/O operations can make a significant difference in large-scale applications.
7. Keep Code DRY (Don’t Repeat Yourself): Avoid duplicating code within loops. If you find yourself repeating the same or similar code, consider creating a function to encapsulate that logic.
With this newfound understanding, you can confidently use ‘For Loops’ in your coding projects, whether you’re building software applications, analyzing data, or working on complex algorithms. Remember that practice is key to mastering this construct, so keep experimenting and refining your ‘For Loop’ skills.
Our blog on ‘For Loops in C’ is only a guide for your coding journey. Visit Newtum’s website and browse our broad online coding courses to realize your full coding potential. We provide the resources and knowledge you require to excel in every aspect of programming, from PHP, C Programming for kids, and Java. Happy coding!