Division program in C Programming, step-by-step guide with an easy explanation and detailed source code.
Table of Contents
Introduction: Division program in C
A division of two numbers is required in mathematical problems. Well, complicated and real-life problems involve many more mathematical functions but for learning it’s important to learn basically by a Division program in C Programming. This program explains “how to write a program that divides two numbers in the simplest possible way. All the variables used are float since division may give a decimal output which requires float to store decimal values in the result.”
This program accepts two inputs scanf input function. The first number accepts the Numerator and the second number is the denominator. After the division, the output is displayed using the printf statement. The division process in C is used in many different programs like calculating the discount percentage, calculating interest, etc. Division programs explain the basic usage of the division operator and how to use division in complicated Programs.
To know more about C Programming and its history please read this blog first “C Programming Complete Guide 2022“.
Division program with int Data Type
Now we write an example that will do the division work. Before we write a program. Since we need to understand this concept we have explained this method.
Declaring Variables in C Program
Here, to start we will create a program that will Division two numbers. Here we will create 3 integers using the keyword int variables a, b, and c variable to store the result.
main() { int a, b, c; }
Now, we write printf so that the user will understand to enter any number
main() { int a, b, c; printf("\n Welcome to Division Program"); printf("\n Enter value for a"); }
then we write scanf statements to accept user input.
main() { int a, b, c; printf("\n Welcome to Division Program"); printf("\n Enter value for a"); scanf("%d",&a); }
Business Logic to Division in C Programming
Write a similar statement for variable b.
main() { int a, b, c; printf("\n Welcome to Division Program"); printf("\n Enter value for a : "); scanf("%d",&a); printf("\n Enter value for b : "); scanf("%d",&b); c = a/b; printf("\n Division Result : %d",c); }
Let’s run the program. Now here we are entering value 10 as the first value and the second value is 4. You can view the source code from the Newtum GitHub repository link over here.
Output :
Welcome to Division Program
Enter value for a : 10
Enter value for b : 4
Division Result : 2
We divide 10/4 its 2.5. So our answer is wrong.
You can watch the video over here which explains the blog in a simple video with VFX animation.
Division program with float Data Type
There is a reason for this and we did it purposely. Because this will explain the difference between integer and float. Since we declare the variable as integer and it can’t hold the decimal.
To get the correct output we will have to change the integer to float.
Declaring Variables in C Program
Here, to start we will create a program that will division of two numbers. Here we will create 3 float using the keyword int variables a, b, and c variable to store the result.
main() { float a, b, c; }
Now we write printf so that the user will understand to enter any number for the division,
main() { float a, b, c; printf("\n Welcome to Division Program"); printf("\n Enter value for a"); }
then we write scanf statements to accept user input.
main() { float a, b, c; printf("\n Welcome to Division Program"); printf("\n Enter value for a"); scanf("%f",&a); }
Business Logic to Division in C Programming
Write a similar statement for variable b.
main() { float a, b, c; printf("Welcome to Division Program"); printf("\n Enter value for a : "); scanf("%f",&a); printf("\n Enter value for b : "); scanf("%f",&b); c=a/b; printf("\n Division Result : %f",c); }
Let’s run the program. Now here we are entering value 10 as the first value and the second value is 4.
Welcome to Division Program
Enter value for a : 10
Enter value for b : 4
Output :
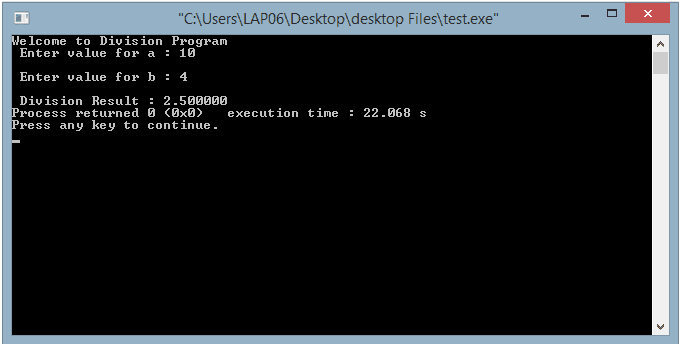
Welcome to Division Program
Enter value for a : 10
Enter value for b : 4
Division Result : 2.5
The output is 2.5. That’s right.
How to view all topics on C Programming
Watch full programming series on Amazon Prime free with Amazon Prime Membership.
- Amazon Prime link for the USA: https://www.amazon.com/dp/B07WFYJW6G
- Amazon Prime link for the UK: https://www.amazon.co.uk/dp/B07WFYQZ1G
For Other Countries: To watch C Programming Content in another country, please visit the URL: https://newtum.com/course-details/c-programming-online