Best Explanation of Logical AND operator in C Programming Language. With Student Grading Program. An expression containing a logical operator returns either 0 or 1 depending upon whether the expression results in true or false. Logical operators are commonly used in decision-making in C programming.
The ‘&&’ operator returns true when both the conditions under consideration are satisfied. Otherwise, it returns false. For example, a && b returns true when both a and b are true (i.e. non-zero).
To learn more about C Programming, please read this blog first, “C Programming Complete Guide“. You can watch the video over here, which explains the blog in a simple video with VFX animation.
Table of Contents
Logical AND Operator in C Programming Language With Example
Declaring Variables in C Program, We will declare a float variable as a “percentage” to take input from the user.
main() { float percentage; }
We have printf statements, followed by the scanf statement to accept user input.
main() { float percentage; scanf("%f",percentage); }
To make it more friendly, we will add a few statements using printf.
main() { float percentage; printf("Calculate Division by Percentage"); printf("\nPlease Enter Percentage: "); scanf("%f",&percentage); }
Now your program will be more user-friendly to understand the user.
Program Logic for Student Grading Program
Let’s implement the Program Logic for Student Grading Program in C language. if the percentage >= 60, it’s the first Division.
main() { float percentage; printf("Calculate Division by Score"); printf("\nPlease Enter Percentage: "); scanf("%f",&percentage); if(percentage >= 60) printf("First Division "); }
Here we have used the “AND” operator. And this operator requires both conditions to be true. Please make sure that all the statements are followed by a semicolon. Next line, we will write the condition for the 3rd division. i.e. percentage greater than 40 and less than 50.
main() { float percentage; printf("Calculate Division by Score"); printf("\nPlease Enter Percentage: "); scanf("%f",&percentage); if(percentage >= 60) printf("First Division "); }
Now we have the final condition, i.e. less than 40. Its failed.
main() { float percentage; printf("Calculate Division by Score"); printf("\nPlease Enter Percentage: "); scanf("%f",&percentage); if(percentage >= 60) printf("First Division "); if(percentage >= 50 && percentage <60 ) printf("Second Division "); if(percentage >= 40 && percentage <50 ) printf("Third Division "); if(percentage < 40) printf("FAILED"); }
Let’s execute the program.
Output :
Let’s see the output.
Calculate Division by Score
Please Enter Percentage: 55
Second Division
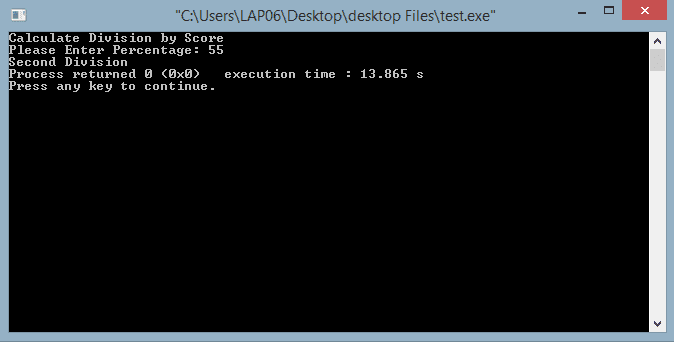
Well, Let’s iterate through the program. You entered the value 55, and the Computer reached the first condition. It checks whether the percentage is greater than 60 or not.
The answer is negative; hence this statement of printf is not executed. In the next line, we will check two conditions. First, it’s greater than 50; yes, that’s true. Next is less than 60, it’s also true. When we put “AND” to join the conditions, the result of both the conditions should be true, then only it is considered true.
Well, the statement “Second Division” is printed. But the program won’t stop here. Since there are a few more lines yet to be executed, It will go to the next line. Here it will check a percentage greater than 50, and the answer is true; the Next condition in the. Same “IF” statement is a percentage less than 50. The result is false. So in this “IF” statement, we have one condition as true and one condition as false. So the final result is false since we have AND operator.
I hope you understand this part. If we have both conditions true in “IF” ‘s && statement, then it’s completely considered true. Well, the program doesn’t stop here; it goes to the next line again. Here the condition is a percentage of less than 40. The answer is false. Now it’s the last line, the end of the function.
Well, this is how we handle multiple conditions in “if and else” using operators. There is more to be learned about IF and ELSE statements.
How to view all topics on C Programming
Watch the full programming series on Amazon Prime free with Amazon Prime Membership.
- Amazon Prime link for the USA: https://www.amazon.com/dp/B07WFYJW6G
- Amazon Prime link for the UK: https://www.amazon.co.uk/dp/B07WFYQZ1G
- For Other Countries: To watch C Programming Content in another country, please visit the URL:https://newtum.com/course-details/c-programming-online
Frequently Asked Questions about Logical And Operators in C Programming
Six types of operators, namely
1. Greater than — x > y
2. Greater than equal to — x>= y
3. Less than — x < y
4. Less than equal to — x<= y
5. Equal to — x==y
6. Not equal to — x!=y
If and else can have multiple conditions using AND, OR, and Not Operators. We can use else if statements to avoid unwanted execution. Well, that’s about IF and ELSE. We will study more about it in the coming chapters. Practice well.