ERC721 tokens represent unique digital assets on a blockchain, different from regular cryptocurrencies like Bitcoin or Ethereum. They’re like special digital collectibles, each with its own distinct value and features. Deploying smart contracts for these tokens on a custom blockchain is like creating a unique rulebook for these collectibles. Imagine if every piece of digital art or game character had its own set of rules, ensuring it’s one-of-a-kind and can be securely bought, sold, or transferred. This process allows developers to bring truly rare and special digital items to life, providing a safe and transparent way for people to own and trade these unique assets on their very own blockchain.
Understanding ERC721 Smart Contracts
Blockchain technology isn’t just about cryptocurrencies; it’s a platform for innovation, and ERC721 tokens are a prime example. These tokens adhere to a particular standard, allowing for unique digital assets on a blockchain.
Imagine ERC721 as a rulebook defining how non-fungible tokens (NFTs) should work. Unlike regular cryptocurrencies where each token is interchangeable, ERC721 tokens are like digital snowflakes—each one is unique. This standard sets guidelines ensuring these tokens can be created, owned, and traded uniquely.
Overview of the Structure and Functions of ERC721 Contracts
Imagine ERC721 contracts as the rulebook for creating and managing special digital items on a blockchain. They’re like the blueprint that tells the blockchain how to handle these unique items.
1. Creating Unique Items
Think of these contracts as factories. They have a function called mint that acts like a machine creating new, one-of-a-kind digital items. Each item is special and cannot be duplicated.
2. Keeping Track of Ownership
Imagine these contracts as a record-keeper. They have a function called transferFrom that helps in moving these digital items from one person to another securely. It’s like changing the owner of a unique digital collectible or asset.
3. Identifying Each Item
These contracts also have a way to assign a unique ID, let’s call it a ‘digital fingerprint,’ to each item. This makes sure that every digital item is different and can be easily identified on the blockchain.
4. Security and Uniqueness
These contracts ensure that no one can create fake copies of your unique digital item. They make sure that each item is special, just like a rare collector’s item in the real world.
In simple words, ERC721 contracts are like a set of rules ensuring that every digital collectible, artwork, or unique item on the blockchain is genuine, can be owned, and traded securely. They help create a world where digital uniqueness matters just as much as real-world uniqueness.
Requirements for deploying ERC721 Smart Contract
- MetaMask Wallet: Essential for managing transactions and engaging with the network. For installation, access the following link: MetaMask Installation.
- Remix IDE: An inclusive development environment utilized for coding, testing, and deploying smart contracts. To explore Remix IDE, visit: remix.ethereum.org.
- Solidity Language: Understanding Solidity, the programming language for smart contracts, is crucial for writing the ERC20 token contract code.
- Gas Fees on Main Network: Having coins to cover gas fees is essential when deploying smart contracts on the mainnet.
- IPFS: IPFS CLI installed, IPFS is a decentralized peer-to-peer network protocol designed to create a distributed method of storing and sharing hypermedia and files on the internet.
Deploying Your ERC721 Token Contract
Now that we know what ERC-721 tokens are and how they work, let’s see how we can build and deploy our own tokens.
Before proceeding further make sure you have setup your metamask wallet with your coin network, Here we are already connected to metamask with our newtum coin.so, Let’s take a clear look at it:
Adding Files to IPFS
Before writing our NFT contract, we need to host our art for NFT and create a metadata file; for this, we’ll use IPFS – a peer-to-peer file storing and sharing distributed system. Download and install IPFS CLI based on your Operating system by following the installation guide in IPFS docs.
Following are the steps for hosting the image and metadata file.
Step 1: Creating IPFS repo.
Start the IPFS repo by typing the following in a terminal/cmd window.
ipfs init
Step 2: Starting the IPFS daemon.
Start the IPFS daemon, open a separate terminal/cmd window, and type the following.
ipfs daemon
Step 3: Adding an image to IPFS
Go to the first terminal window and add the image to IPFS (art.png here).
ipfs add art.png
Copy the hash starting with Qm and add the “https://ipfs.io/ipfs/” prefix to it; it must look something like this https://ipfs.io/ipfs/QmQEVVLJUR1WLN15S49rzDJsSP7za9DxeqpUzWuG4aondg
Step 4: Adding JSON file to IPFS
Next, you have to add a JSON file to IPFS. Begin by creating a JSON file and saving it on the same directory in the image form.
Check out the file format of JSON mentioned below,
{ "name": "NFT Art", "description": "This image shows the true nature of NFT.", "image": "https://ipfs.io/ipfs/QmZzBdKF7sQX1Q49CQGmreuZHxt9sVB3hTc3TTXYcVZ7jC" }
Now, add the JSON file.
ipfs add nft.json
Take the hash beginning with Qm and prefix it with “https://ipfs.io/ipfs/”; it must look something like this https://ipfs.io/ipfs/QmUFbUjAifv9GwJo7ufTB5sccnrNqELhDMafoEmZdPPng7
Save this URL. We’ll need this to mint our NFT.
Creating Our Own Token
For ease and security, we’ll use the OpenZeppelin ERC-721 contract to create our NFT. With OpenZeppelin, we don’t need to write the whole ERC-721 interface. Instead, we can import the library contract and use its functions.
Head over to the Ethereum Remix IDE and make a new Solidity file, for example – Chef.sol
On line 11, replace the name and Symbol of token from “Chef”, “CHEF”
Paste the following code into your new Solidity script:
// SPDX-License-Identifier: MIT pragma solidity ^0.8.20; import "@openzeppelin/contracts@5.0.0/token/ERC721/ERC721.sol"; import "@openzeppelin/contracts@5.0.0/token/ERC721/extensions/ERC721URIStorage.sol"; import "@openzeppelin/contracts@5.0.0/token/ERC721/extensions/ERC721Burnable.sol"; import "@openzeppelin/contracts@5.0.0/access/Ownable.sol"; contract Chef is ERC721, ERC721URIStorage, ERC721Burnable, Ownable { constructor(address initialOwner) ERC721("Chef", "CHEF") Ownable(initialOwner) {} function safeMint(address to, uint256 tokenId, string memory uri) public onlyOwner { _safeMint(to, tokenId); _setTokenURI(tokenId, uri); } // The following functions are overrides required by Solidity. function tokenURI(uint256 tokenId) public view override(ERC721, ERC721URIStorage) returns (string memory) { return super.tokenURI(tokenId); } function supportsInterface(bytes4 interfaceId) public view override(ERC721, ERC721URIStorage) returns (bool) { return super.supportsInterface(interfaceId); } }
Code Explaination:
Line 1: Specifying SPDX license type as MIT. This indicates that the code is licensed under the MIT License, which is a permissive open-source license allowing the code to be used, modified, and distributed with very few restrictions.
Line 2: Declaring the Solidity version as ^0.8.20. This indicates that the code is written using Solidity version 0.8.20 or a compatible version.
Line 4-7: Importing necessary contracts from the OpenZeppelin library.
- ERC721.sol: This contract is imported from the OpenZeppelin library and represents the basic implementation of the ERC721 standard. ERC721 is the standard for non-fungible tokens (NFTs).
- ERC721URIStorage.sol: This contract extends ERC721 and adds functionality for storing and managing metadata URIs associated with NFTs. Metadata URIs typically point to additional information about the NFT.
- ERC721Burnable.sol: This contract also extends ERC721 and adds the ability to burn (destroy) NFTs. It provides a function for permanently removing NFTs from circulation, and only the owner can invoke it.
- Ownable.sol: This contract is used for access control. It allows you to specify an owner who has special privileges within the contract. The owner can perform certain actions that other users cannot.
Line 9: Starting the contract named Chef and mentioning that it extends the ERC721, ERC721URIStorage, and Ownable contracts. This means that the Chef contract inherits the functionality and properties defined in these contracts.
Line 10: The constructor function begins here. Constructors are special functions in Solidity that are executed only once during contract deployment. This constructor takes one argument, initialOwner, which is an Ethereum address.
Line 11: This line calls the constructor of the ERC721 contract with the arguments “Chef” and “CHEF”. It initializes the NFT contract with a name of “Chef” and a symbol of “CHEF.”
Line 12: This line calls the constructor of the Ownable contract, setting the initial owner of the Chef contract to the address provided as initialOwner.
Line 15: Declaring the function safeMint with three arguments: to (the address of the receiver of the NFT token), tokenId (the unique identifier for the token), and uri (the URI of the JSON file associated with the token). This function can only be called by the contract owner (specified by the onlyOwner modifier).
Line 19: Minting a new token by calling the _safeMint function inherited from the ERC721 contract. It creates a new token and assigns it to the specified receiver’s address.
Line 20: Setting the token URI (metadata URI) associated with the token using the _setTokenURI function inherited from the ERC721URIStorage contract. The URI is set based on the provided tokenId and uri.
Line 25-32: Implementing overrides required by Solidity for the ERC721 and ERC721URIStorage contracts. tokenURI is a function that retrieves the metadata URI associated with a given tokenId. It’s marked as public and view, indicating that it’s a read-only function and can be called by anyone.
Line 34-41: Implementing the supportsInterface function, which is required by the ERC721 and ERC721URIStorage contracts. This function checks whether a given interfaceId is supported by the contract and returns a boolean value accordingly.
By combining these functionalities and contracts, the code creates a custom ERC721 token contract named Chef. This contract allows the contract owner to safely mint new tokens, associate metadata URIs with them, and supports the necessary interfaces defined by the ERC721 standard.
When you’re finished, compile the smart contract and deploy it using Injected Provider (make sure to select your network on Metamask before compiling the contract). Then, paste your wallet address into the box just near the Deploy button to define the initialOwner parameter of the constructor function. Lastly, click Deploy on Remix.IDE.
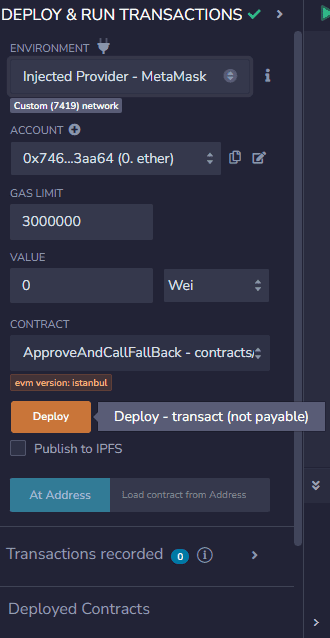
If you receive an error message before deployment – “This contract may be abstract”, make sure to select the appropriate contract under the Contract tab.
Confirm the transaction in Metamask:
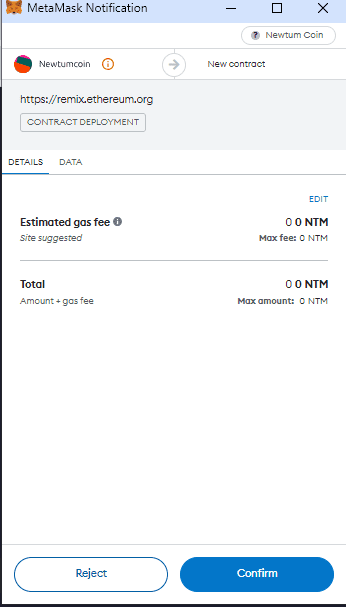
Now go to the “Deployed Contracts” section in Remix and expand the deployed contract. You’ll see a bunch of functions/methods. Expand the safeMint function and add the following details:
- Add your wallet address in the _to the field.
- Enter any Big number value in the _tokenId field (we suggest 1 since it’s the first token being minted).
- Add the URI of the JSON file in the _uri field, which we obtained in the previous section.
Click on transact and confirm the transaction from MetaMask. It could take a couple of minutes but you can always confirm the transaction was executed via a block explorer. Now you have the NFT on the your chain.
You can check other details like name, symbol, ownerOf, or tokenURI by entering the token id we mentioned earlier.
Deploying ERC721 tokens on custom blockchains expands opportunities for creating unique digital assets with individual ownership and proven scarcity. This technology not only revolutionizes digital collectibles but also offers diverse applications across industries, promising a future where ownership and authenticity of digital assets are securely managed on decentralized networks.